Instructions This assignment must follow directions exactly. Create a class Lab02 with a main method, and put all of the following code into the main method: Print the prompt shown below and ask the user for the number of exemptions. The number of exemptions is an integer. Print the prompt shown below and ask the user for their gross salary. The gross salary represents dollars, which can be entered with or without decimal points. Print the prompt shown below and ask the user for their interest income. The interest income represents dollars, which can be entered with or without decimal points. Print the prompt shown below and ask the user for their capital gains income. The capital gains represents dollars, which can be entered with or without decimal points. Print the prompt shown below and ask the user for the amount of charitable contributions. The charitable contributions represents dollars, which can be entered with or without decimal points. Perform the calculation of total income, as shown in the Formula section. Perform the calculation of the adjusted income, as shown in the Formula section. Perform the calculation of the total tax, as shown in the Formula section. Print out the total income, adjusted gross income, and total tax. The example below shows five lines of user input followed by three lines of program output: Number of Exemptions: 3 Gross Salary: 67234.45 Interest Income: 2145.32 Capital Gains: 523.01 Charitable Contributions: 872.45 Total Income: $69902.78 Adjusted Income: $64530.33 Total Tax: $11508.49 Formulas Total Income = Gross Salary + Interest Income + Capital Gains Adjusted Income = Total Income - (Number of Exemptions * 1500.00) - Charitable Contributions Total Tax computation: 0% on Adjusted Income above $0 and below $10,000 + (<= $10,000) 15% on Adjusted Income above $10,000 and below $32,000 + ( > $10,000 but <= $32,000) 23% on Adjusted Income above $32,000 and below $50,000 + ( > $32,000 but <= $50,000) 28% of Adjusted Income above $50,000 ( > $50,000) For the example above, we compute the Total Tax in the example above as follows: 15% * ($32,000.00-$10,000.00) = $3300.00 23% * ($50,000.00-$32,000.00) = $4140.00 28% * ($64,530.33-$50,000.00) = $4068.49 Adding up the above we get a Total Tax of $11,508.49, that's a significant tax bill. Specifications Your program must meet the following specifications: Work on your own. The name of the source code file must be exactly Lab02.java Name it exactly: upper/lower case letters are important! Comments at the top with your name, short description of this program, e-Name (e-mail), date and course title. The output format must exactly match the example shown above, including blank line, white space and capitalization. Use variables to store everything, don't try to just do all the calculation in a print statement! You may use integer or floating point for the computations. The output should not report more than 2 digits after the decimal. Your program should be accurate to within $0.01.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Instructions
This assignment must follow directions exactly. Create a class Lab02 with a main method, and put all of the following code into the main method:
- Print the prompt shown below and ask the user for the number of exemptions.
The number of exemptions is an integer.
- Print the prompt shown below and ask the user for their gross salary.
The gross salary represents dollars, which can be entered with or without decimal points.
- Print the prompt shown below and ask the user for their interest income.
The interest income represents dollars, which can be entered with or without decimal points.
- Print the prompt shown below and ask the user for their capital gains income.
The capital gains represents dollars, which can be entered with or without decimal points.
- Print the prompt shown below and ask the user for the amount of charitable contributions.
The charitable contributions represents dollars, which can be entered with or without decimal points.
- Perform the calculation of total income, as shown in the Formula section.
- Perform the calculation of the adjusted income, as shown in the Formula section.
- Perform the calculation of the total tax, as shown in the Formula section.
- Print out the total income, adjusted gross income, and total tax.
The example below shows five lines of user input followed by three lines of program output:
Number of Exemptions: 3
Gross Salary: 67234.45
Interest Income: 2145.32
Capital Gains: 523.01
Charitable Contributions: 872.45
Total Income: $69902.78
Adjusted Income: $64530.33
Total Tax: $11508.49
Formulas
Total Income = Gross Salary + Interest Income + Capital Gains
Adjusted Income = Total Income - (Number of Exemptions * 1500.00) - Charitable Contributions
Total Tax computation:
0% on Adjusted Income above $0 and below $10,000 + (<= $10,000)
15% on Adjusted Income above $10,000 and below $32,000 + ( > $10,000 but <= $32,000)
23% on Adjusted Income above $32,000 and below $50,000 + ( > $32,000 but <= $50,000)
28% of Adjusted Income above $50,000 ( > $50,000)
For the example above, we compute the Total Tax in the example above as follows:
15% * ($32,000.00-$10,000.00) = $3300.00
23% * ($50,000.00-$32,000.00) = $4140.00
28% * ($64,530.33-$50,000.00) = $4068.49
Adding up the above we get a Total Tax of $11,508.49, that's a significant tax bill.
Specifications
Your program must meet the following specifications:
- Work on your own.
- The name of the source code file must be exactly Lab02.java
- Name it exactly: upper/lower case letters are important!
- Comments at the top with your name, short description of this program, e-Name (e-mail), date and course title.
- The output format must exactly match the example shown above, including blank line, white space and capitalization.
- Use variables to store everything, don't try to just do all the calculation in a print statement!
- You may use integer or floating point for the computations.
- The output should not report more than 2 digits after the decimal.
- Your program should be accurate to within $0.01.
Note:
- Comments at the top with your name, e-mail, date and course.
- We expect programming assignments to be implemented using Java 1.8 (the version installed in the SB 0208, VMware Horizon, and OpenLab SRC 203 and SB Lab). Your code will be tested on the machines (or machines installed the same Java compiler and JVM) in the either SB 0208, VMware Horizon, or OpenLab, so make sure your code runs on those machines.
- Submit your program to Blackboard.
- No late, read the syllabus for the late policy.
I will test your program as follows (your submitted program name is Lab02.java)
javac Lab02.java
java Lab02
Note: my input values for testing your program varies, for example the exemptions maybe 1 and the Gross Salary may be 48520.31, … etc.



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

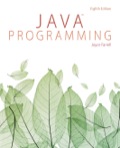
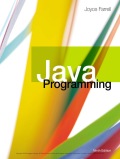
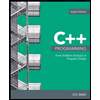
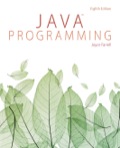
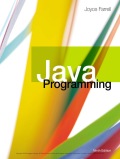
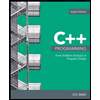
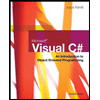