ava Use eclipse for this exercise. Create a class for pets. There should be fields for name, name of owner, species (dog, cat, etc.), breed (basset hound, domestic shorthair, etc.), age (a double), and weight (a double). Create a default constructor that does nothing and a constructor that will initialize all fields using values passed in as parms. Create a method isSpecies which has one parm, a String. Return true if the species in this is the same as the parm, otherwise return false. For example, if the parm is "hamster", then the method will return true if this is a hamster, and will return false if it's not. Create a toString method that returns a String containing all fields. You can create a multi-line String by including newlines; make sure the String is nicely formatted. Do NOT create any set or get methods. Client/demo/driver code (in a separate class): Write a method to read Pets from a file. The method will have one parm which is an array of Pets. The input file is named pets.dat. The data for each field of Pet will be on a separate line. Read in the fields for a Pet, create a Pet object, and add it to the array. Read till end of file. The method will return the number of Pets that were read in and stored in the array. You can assume that the array is big enough to hold all of the Pets in the file. Write a method to print all Pets in the array. The parms are the array and the number of Pets in the array. Don't print array elements that don't contain a Pet. Write a method to print all Pets of a given species. The species, the array, and the number of Pets in the array are parms. In main, create an array of Pets; make your array large enough to hold 25 Pets. Call the method to read Pets, then use the previous method to print all cats and all dogs. Print a heading for the cats and for the dogs. Then print a heading for all Pets and print all of the Pets in the array.

Code
Pet.java
public class Pet
{
private String name,ownerName,species,breed;
private double age,weight ;
public Pet()
{
}
public Pet(String name,String owner,String spe,String breed,double age,double wght)
{
this.name=name;
this.ownerName=owner;
this.species=spe;
this.breed=breed;
this.age=age;
this.weight=wght;
}
@Override
public String toString()
{
String str="";
str+="Name: "+name;
str+="\nOwner Name: "+ownerName;
str+="\nSpecies: "+species;
str+="\nBreed: "+breed;
str+="\nAge: "+age;
str+="\nWeight: "+weight+"\n";
return str;
}
}
petDriver.java with main methid driver class
import java.io.*;
import java.util.*;
public class petDriver {
public static void main(String[] args)
{
ArrayList<Pet> pets = new ArrayList<Pet>();
readData(pets);
printData(pets);
}
private static void printData(ArrayList<Pet> pets)
{
for(Pet p:pets)
{
System.out.println(p);
}
}
private static void readData(ArrayList<Pet> pets)
{
BufferedReader reader;
String name,oname,spe,breed;
double age,weight;
try
{
reader = new BufferedReader(new FileReader("pets.dat"));
String line = reader.readLine();
while (line != null)
{
name=line;
// read next line
line = reader.readLine();
oname=line;
// read next line
line = reader.readLine();
spe=line;
// read next line
line = reader.readLine();
breed=line;
// read next line
line = reader.readLine();
age=Double.parseDouble(line);
// read next line
line = reader.readLine();
weight=Double.parseDouble(line);
// read next line
pets.add(new Pet(name,oname,spe,breed,age,weight));
line = reader.readLine();
}
reader.close();
}
catch (IOException e)
{
System.out.println("File not found");
}
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

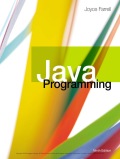
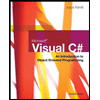
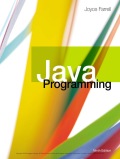
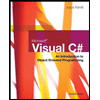