#include #include "HPVISACard.cpp" #include using namespace std; int main() { // Declare variables string type; double amount; int rewardPercentage,choice; // print menu cout<<"Menu"<<"\n1.VISA Card"<<"\n2.HPVISA Card"<>choice; // conditionally execute based on user choice switch(choice){ // case 1 case 1:{ // ask user to enter transaction type cout<<"Enter the transaction type"<>type; // ask user to enter transaction amount cout<<"Enter the transaction amount"<>amount; // ask user to enter reward points cout<<"Enter the reward points"<>rewardPercentage; // create VISACard class object using parameterized constructor VISACard v1(type,amount,rewardPercentage); // print card details by calling suitable methods cout<<"Card details:"<>type; // ask user to enter amount cout<<"Enter the transaction amount"<>amount; // create a object of class HPVISACard HPVISACard hv1(type,amount); // print card details by calling suitable methods cout<<"Card details:"< #include #include "VISACard.cpp" using namespace std; class HPVISACard :public VISACard{ public: void computeRewardPoints() { // declare RewardPoints to store points float RewardPoints; // if type == Fueal if(getType()=="Fuel"){ // calculate 3% amount and add 10 extra points RewardPoints = (amount * (3/100.0))+10; } // else calculate 3% of amount else{ RewardPoints = (amount * (3/100.0)); } // finally print Reward Points cout<<"Reward point:"< #include using namespace std; class VISACard { // protected members of class protected: string type; double amount; // private members of class private: int rewardPercentage; // public members of class public: void computeRewardPoints() { // calculate Reward points based on reward percentage float RewardPoints = amount*(getRewardPercentage()/100.0); // finally print reward points cout<<"Reward point:"<type = type; } void setAmount (double amount){ this->amount = amount; } void setRewardPercentage (int rewardPercentage){ this->rewardPercentage = rewardPercentage; } // getters int getRewardPercentage(){ return rewardPercentage; } string getType(){ return type; } double getAmount(){ return amount; } }; YOU HAVE TO FIND WHY THE OUTPUT IS NOT MATCHING AND MODIFY MY CODE ACCORDING TO THAT.
QUESTION AND COLLEGE COMPILER OUTPUT ATTACHED IN IMAGE . PLEASE SEE
AND MY SOLUTION TO THE PROBLEM IS GIVEN BELOW.
------------MY SOLUTION----------
MAIN.CPP
#include <iostream> #include "HPVISACard.cpp" using namespace std; int main() { // ask user to enter his choice // ask user to enter transaction amount // ask user to enter reward points // print card details by calling suitable methods // ask user to enter amount // create a object of class HPVISACard // print card details by calling suitable methods |
HPVISCACard.cpp
#include <iostream> class HPVISACard :public VISACard{ // if type == Fueal // else calculate 3% of amount // finally print Reward Points // calling super class constructor |
viscard.cpp
#include <iostream> class VISACard { // private members of class // public members of class // finally print reward points public: // constructor with 2 params // setters // getters |
YOU HAVE TO FIND WHY THE OUTPUT IS NOT MATCHING AND MODIFY MY CODE ACCORDING TO THAT.



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 10 images

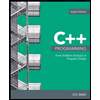
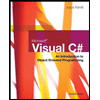
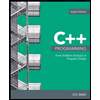
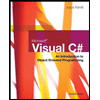