In this exercise, you have to create a class called Point which can calculate distance between two points in the x-y plane. Problem Statement# You have to implement a class called Point that represents a specific point in the x-y plane. It should contain the following: ● fields: ○ x( integer type) ○ y( integer type) ● methods: ○ default constructor that initializes the point at (0,0)(0, 0)(0,0) ○ parameterized constructor that takes input x and y and initializes the point to the respective coordinates. ○ float distance(), a method which calculates the distance of the point (represented by the object) from the origin, i.e. (0,0)(0, 0)(0,0) ○ float distance(x1, y1), a method which calculates the distance between the point represented by the class object and (x1,y1)(x1, y1)(x1,y1) Sample Input# Point p1 = new Point(5, 5); Sample Output# distance() => 7.071 distance(2, 1) => 5.0 Part of the solution import java.lang.Math; class Point { // Private fields private int x; private int y; // Default Constructor public Point() { // Implement the function } // Parameterized Constructor public Point(int x, int y) { // Implement the function } public double distance() { // Implement the function return 0; } public double distance(int x2, int y2) { // Implement the function return 0; } } class Demo { public static void main(String args[]) { Point p1 = new Point(5, 5); System.out.println(p1.distance()); System.out.println(p1.distance(2, 1)); } }
In this exercise, you have to create a class called Point which can calculate distance between two points in the x-y plane. Problem Statement# You have to implement a class called Point that represents a specific point in the x-y plane. It should contain the following: ● fields: ○ x( integer type) ○ y( integer type) ● methods: ○ default constructor that initializes the point at (0,0)(0, 0)(0,0) ○ parameterized constructor that takes input x and y and initializes the point to the respective coordinates. ○ float distance(), a method which calculates the distance of the point (represented by the object) from the origin, i.e. (0,0)(0, 0)(0,0) ○ float distance(x1, y1), a method which calculates the distance between the point represented by the class object and (x1,y1)(x1, y1)(x1,y1) Sample Input# Point p1 = new Point(5, 5); Sample Output# distance() => 7.071 distance(2, 1) => 5.0 Part of the solution import java.lang.Math; class Point { // Private fields private int x; private int y; // Default Constructor public Point() { // Implement the function } // Parameterized Constructor public Point(int x, int y) { // Implement the function } public double distance() { // Implement the function return 0; } public double distance(int x2, int y2) { // Implement the function return 0; } } class Demo { public static void main(String args[]) { Point p1 = new Point(5, 5); System.out.println(p1.distance()); System.out.println(p1.distance(2, 1)); } }
Chapter3: Using Methods, Classes, And Objects
Section: Chapter Questions
Problem 10PE
Related questions
Question
In this exercise, you have to create a class called Point which can calculate distance between two points in the x-y plane.
Problem Statement#
You have to implement a class called Point that represents a specific point in the x-y plane. It should contain the following:
● fields:
○ x( integer type)
○ y( integer type) ● methods:
○ default constructor that initializes the point at (0,0)(0, 0)(0,0)
○ parameterized constructor that takes input x and y and initializes the point to the respective coordinates.
○ float distance(), a method which calculates the distance of the point (represented by the object) from the origin, i.e. (0,0)(0, 0)(0,0)
○ float distance(x1, y1), a method which calculates the distance between the point represented by the class object and (x1,y1)(x1, y1)(x1,y1)
Sample Input#
Point p1 = new Point(5, 5);
Sample Output# distance() => 7.071 distance(2, 1) => 5.0
Part of the solution
import java.lang.Math;
class Point {
// Private fields
private int x;
private int y;
// Default Constructor
public Point() {
// Implement the function
}
// Parameterized Constructor
public Point(int x, int y) {
// Implement the function
}
public double distance() {
// Implement the function
return 0;
}
public double distance(int x2, int y2) {
// Implement the function
return 0;
}
}
class Demo {
public static void main(String args[]) {
Point p1 = new Point(5, 5);
System.out.println(p1.distance());
System.out.println(p1.distance(2, 1));
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
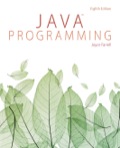
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
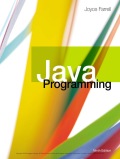
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
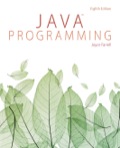
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
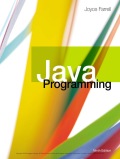
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
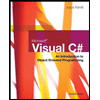
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,