Can you please create 2 files such that it will pass these 2 tests each: 1. PlayerStructureTest: package walking.game.tests; import static check.CheckThat.*; import static check.CheckThat.Condition.*; import org.junit.jupiter.api.*; import org.junit.jupiter.api.condition.*; import check.CheckThat; public class PlayerStructureTest { @BeforeAll publicstaticvoidinit() { CheckThat.theClass("walking.game.player.Player") .thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL); } @Test @DisabledIf(notApplicable) publicvoidfieldScore() { it.hasField("score: int") .thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE) .thatHas(GETTER) .thatHasNo(SETTER); } @Test @DisabledIf(notApplicable) publicvoidfieldDirection() { it.hasField("direction: walking.game.util.Direction") .thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_SUBCLASSES) .thatHas(GETTER) .thatHasNo(SETTER) .withInitialValue("UP"); } @Test @DisabledIf(notApplicable) publicvoidconstructor() { it.hasConstructor(withNoArgs()) .thatIs(VISIBLE_TO_ALL); } @Test @DisabledIf(notApplicable) publicvoidmethodAddToScore() { it.hasMethod("addToScore", withParams("score: int")) .thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL) .thatReturnsNothing(); } @Test @DisabledIf(notApplicable) publicvoidmethodTurn() { it.hasMethod("turn", withNoParams()) .thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL) .thatReturnsNothing(); } } 2. WalkingBoardWithPlayersStructureTest: package walking.game.tests; import static check.CheckThat.*; import static check.CheckThat.Condition.*; import org.junit.jupiter.api.*; import org.junit.jupiter.api.condition.*; import check.CheckThat; public class WalkingBoardWithPlayersStructureTest { @BeforeAll publicstaticvoidinit() { CheckThat.theClass("walking.game.WalkingBoardWithPlayers", withParent("walking.game.WalkingBoard")) .thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL); } @Test @DisabledIf(notApplicable) publicvoidfieldPlayers() { it.hasField("players: array of walking.game.player.Player") .thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE) .thatHasNo(GETTER, SETTER); } @Test @DisabledIf(notApplicable) publicvoidfieldRound() { it.hasField("round: int") .thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE) .thatHasNo(GETTER, SETTER); } @Test @DisabledIf(notApplicable) publicvoidfieldSCORE_EACH_STEP() { it.hasField("SCORE_EACH_STEP: int") .thatIs(USABLE_WITHOUT_INSTANCE, NOT_MODIFIABLE, VISIBLE_TO_ALL) .thatHasNo(GETTER, SETTER) .withInitialValue(13); } @Test @DisabledIf(notApplicable) publicvoidconstructor01() { it.hasConstructor(withArgs("board: array of array of int", "playerCount: int")) .thatIs(VISIBLE_TO_ALL); } @Test @DisabledIf(notApplicable) publicvoidconstructor02() { it.hasConstructor(withArgs("size: int", "playerCount: int")) .thatIs(VISIBLE_TO_ALL); } @Test @DisabledIf(notApplicable) publicvoidmethodInitPlayers() { it.hasMethod("initPlayers", withParams("playerCount: int")) .thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_NONE) .thatReturnsNothing(); } @Test @DisabledIf(notApplicable) publicvoidmethodWalk() { it.hasMethod("walk", withParams("stepCounts: vararg of int")) .thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL) .thatReturns("array of int"); } }
Can you please create 2 files such that it will pass these 2 tests each: 1. PlayerStructureTest: package walking.game.tests; import static check.CheckThat.*; import static check.CheckThat.Condition.*; import org.junit.jupiter.api.*; import org.junit.jupiter.api.condition.*; import check.CheckThat; public class PlayerStructureTest { @BeforeAll publicstaticvoidinit() { CheckThat.theClass("walking.game.player.Player") .thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL); } @Test @DisabledIf(notApplicable) publicvoidfieldScore() { it.hasField("score: int") .thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE) .thatHas(GETTER) .thatHasNo(SETTER); } @Test @DisabledIf(notApplicable) publicvoidfieldDirection() { it.hasField("direction: walking.game.util.Direction") .thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_SUBCLASSES) .thatHas(GETTER) .thatHasNo(SETTER) .withInitialValue("UP"); } @Test @DisabledIf(notApplicable) publicvoidconstructor() { it.hasConstructor(withNoArgs()) .thatIs(VISIBLE_TO_ALL); } @Test @DisabledIf(notApplicable) publicvoidmethodAddToScore() { it.hasMethod("addToScore", withParams("score: int")) .thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL) .thatReturnsNothing(); } @Test @DisabledIf(notApplicable) publicvoidmethodTurn() { it.hasMethod("turn", withNoParams()) .thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL) .thatReturnsNothing(); } } 2. WalkingBoardWithPlayersStructureTest: package walking.game.tests; import static check.CheckThat.*; import static check.CheckThat.Condition.*; import org.junit.jupiter.api.*; import org.junit.jupiter.api.condition.*; import check.CheckThat; public class WalkingBoardWithPlayersStructureTest { @BeforeAll publicstaticvoidinit() { CheckThat.theClass("walking.game.WalkingBoardWithPlayers", withParent("walking.game.WalkingBoard")) .thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL); } @Test @DisabledIf(notApplicable) publicvoidfieldPlayers() { it.hasField("players: array of walking.game.player.Player") .thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE) .thatHasNo(GETTER, SETTER); } @Test @DisabledIf(notApplicable) publicvoidfieldRound() { it.hasField("round: int") .thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE) .thatHasNo(GETTER, SETTER); } @Test @DisabledIf(notApplicable) publicvoidfieldSCORE_EACH_STEP() { it.hasField("SCORE_EACH_STEP: int") .thatIs(USABLE_WITHOUT_INSTANCE, NOT_MODIFIABLE, VISIBLE_TO_ALL) .thatHasNo(GETTER, SETTER) .withInitialValue(13); } @Test @DisabledIf(notApplicable) publicvoidconstructor01() { it.hasConstructor(withArgs("board: array of array of int", "playerCount: int")) .thatIs(VISIBLE_TO_ALL); } @Test @DisabledIf(notApplicable) publicvoidconstructor02() { it.hasConstructor(withArgs("size: int", "playerCount: int")) .thatIs(VISIBLE_TO_ALL); } @Test @DisabledIf(notApplicable) publicvoidmethodInitPlayers() { it.hasMethod("initPlayers", withParams("playerCount: int")) .thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_NONE) .thatReturnsNothing(); } @Test @DisabledIf(notApplicable) publicvoidmethodWalk() { it.hasMethod("walk", withParams("stepCounts: vararg of int")) .thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL) .thatReturns("array of int"); } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Can you please create 2 files such that it will pass these 2 tests each:
1. PlayerStructureTest:
package walking.game.tests;
import static check.CheckThat.*;
import static check.CheckThat.Condition.*;
import org.junit.jupiter.api.*;
import org.junit.jupiter.api.condition.*;
import check.CheckThat;
public class PlayerStructureTest {
@BeforeAll
publicstaticvoidinit() {
CheckThat.theClass("walking.game.player.Player")
.thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL);
}
@Test
@DisabledIf(notApplicable)
publicvoidfieldScore() {
it.hasField("score: int")
.thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE)
.thatHas(GETTER)
.thatHasNo(SETTER);
}
@Test
@DisabledIf(notApplicable)
publicvoidfieldDirection() {
it.hasField("direction: walking.game.util.Direction")
.thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_SUBCLASSES)
.thatHas(GETTER)
.thatHasNo(SETTER)
.withInitialValue("UP");
}
@Test
@DisabledIf(notApplicable)
publicvoidconstructor() {
it.hasConstructor(withNoArgs())
.thatIs(VISIBLE_TO_ALL);
}
@Test
@DisabledIf(notApplicable)
publicvoidmethodAddToScore() {
it.hasMethod("addToScore", withParams("score: int"))
.thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL)
.thatReturnsNothing();
}
@Test
@DisabledIf(notApplicable)
publicvoidmethodTurn() {
it.hasMethod("turn", withNoParams())
.thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL)
.thatReturnsNothing();
}
}
2. WalkingBoardWithPlayersStructureTest:
package walking.game.tests;
import static check.CheckThat.*;
import static check.CheckThat.Condition.*;
import org.junit.jupiter.api.*;
import org.junit.jupiter.api.condition.*;
import check.CheckThat;
public class WalkingBoardWithPlayersStructureTest {
@BeforeAll
publicstaticvoidinit() {
CheckThat.theClass("walking.game.WalkingBoardWithPlayers", withParent("walking.game.WalkingBoard"))
.thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL);
}
@Test
@DisabledIf(notApplicable)
publicvoidfieldPlayers() {
it.hasField("players: array of walking.game.player.Player")
.thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE)
.thatHasNo(GETTER, SETTER);
}
@Test
@DisabledIf(notApplicable)
publicvoidfieldRound() {
it.hasField("round: int")
.thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE)
.thatHasNo(GETTER, SETTER);
}
@Test
@DisabledIf(notApplicable)
publicvoidfieldSCORE_EACH_STEP() {
it.hasField("SCORE_EACH_STEP: int")
.thatIs(USABLE_WITHOUT_INSTANCE, NOT_MODIFIABLE, VISIBLE_TO_ALL)
.thatHasNo(GETTER, SETTER)
.withInitialValue(13);
}
@Test
@DisabledIf(notApplicable)
publicvoidconstructor01() {
it.hasConstructor(withArgs("board: array of array of int", "playerCount: int"))
.thatIs(VISIBLE_TO_ALL);
}
@Test
@DisabledIf(notApplicable)
publicvoidconstructor02() {
it.hasConstructor(withArgs("size: int", "playerCount: int"))
.thatIs(VISIBLE_TO_ALL);
}
@Test
@DisabledIf(notApplicable)
publicvoidmethodInitPlayers() {
it.hasMethod("initPlayers", withParams("playerCount: int"))
.thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_NONE)
.thatReturnsNothing();
}
@Test
@DisabledIf(notApplicable)
publicvoidmethodWalk() {
it.hasMethod("walk", withParams("stepCounts: vararg of int"))
.thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL)
.thatReturns("array of int");
}
}
AI-Generated Solution
Unlock instant AI solutions
Tap the button
to generate a solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
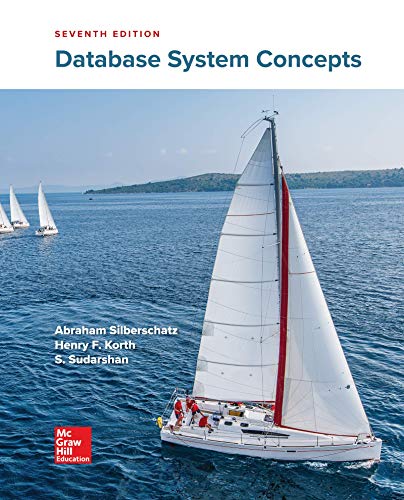
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
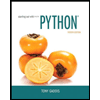
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
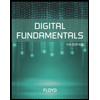
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
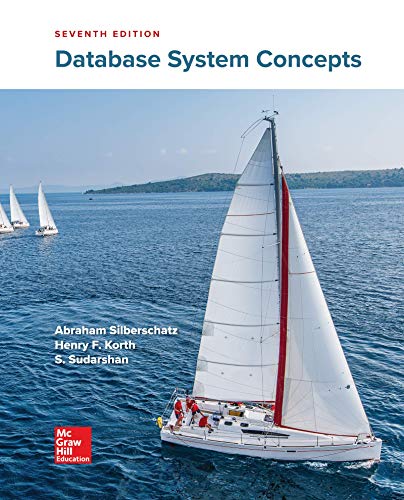
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
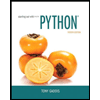
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
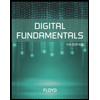
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
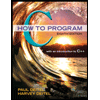
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
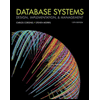
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
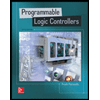
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education