race
.py
keyboard_arrow_up
School
Yale University *
*We aren’t endorsed by this school
Course
351B
Subject
Computer Science
Date
Apr 3, 2024
Type
py
Pages
2
Uploaded by CountIron11913 on coursehero.com
'''
NICK HUGHES
DS2000
HW8
NOV 6TH, 2023
'''
import matplotlib.pyplot as plt
# Import the custom Runner class from the 'runner' module
from runner import Runner
def read_data(filename):
# Initialize an empty list to store Runner objects
runners = []
with open(filename, 'r') as file:
# Read all lines from the file into a list
lines = file.readlines()
# Extract the first line as the header
header = lines[0]
# Extract the remaining lines as data lines
data_lines = lines[1:]
# Iterate through the data lines
for line in data_lines:
# Format each line
data = line.strip().split()
# Extract the first value as the runner's name
name = data[0]
# Extract and convert numeric values as floats and remove the names
runs = [float(run) for run in data[1:] if run.replace('.', '', 1).isdigit()]
runner = Runner(name)
# Iterate through the runs
for run in runs:
# Add each run to the Runner object
runner.add_run(run)
runners.append(runner)
return runners
def main():
runners = read_data('runner_data.txt')
# Create a new figure for the plot
plt.figure() # Iterate through the list of runners with an index
for i, runner in enumerate(runners):
# Different y-values for each runner
runner.y = 10 * (i + 1) # Different color for each runner
# Consulted https://www.geeksforgeeks.org/matplotlib-pyplot-viridis-in-
python/
runner.color = plt.cm.viridis(i / len(runners)) # Iterate through days from 1 to 28
for day in range(1, 29):
# Iterate through the list of runners
for runner in runners:
# Move each runner to the next position
runner.move_next()
# Draw the runner's position
runner.draw()
# Add a legend to the plot
plt.legend(loc="upper left")
# Set the plot title and labels and x-limit
plt.title("February Miles")
plt.xlabel("Distance(Miles)")
plt.xlim(0, 150)
# Save the plot as an image with a day-specific filename
# https://www.geeksforgeeks.org/matplotlib-pyplot-savefig-in-python/ plt.savefig(f"day_{day}.png")
# Clear the current figure for the next day's plot
# https://www.geeksforgeeks.org/matplotlib-pyplot-clf-in-python/ plt.clf()
# Find the runner with the maximum distance traveled
winner = max(runners, key=lambda runner: runner.x) # Print the name and distance of the winner
print(f'The winner is {winner.name} with {winner.x} miles.')
if __name__ == '__main__':
main()
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
a. Add the following operation to the class orderedLinkedList:void mergeLists(orderedLinkedList<Type> &list1,orderedLinkedList<Type> &list2);//This function creates a new list by merging the//elements of list1 and list2.//Postcondition: first points to the merged list// list1 and list2 are emptyConsider the following statements:orderedLinkedList<int> newList;orderedLinkedList<int> list1;orderedLinkedList<int> list2;Suppose list1 points to the list with the elements 2 6 7, and list2 points to the list with the elements 3 5 8. The statement newList.mergeLists(list1, list2); creates a new linked list with the elements in the order 2 3 5 6 7 8, and the object newList points to this list. Also, after the preceding statement executes, list1 and list2 are empty. b. Write the definition of the function template mergeLists to implement the operation mergeLists.
arrow_forward
void Pokedex::wakeupProfessorJimi(std::string) − populates the dynamic array of Entries with data from a valid file path, the function should open the file and use the add (Pokemon*, int) function to insert a Pok ́emon into the correct position in the dynamic array of Entries. Once the position is determined, the Pok ́emon should be inserted into the corresponding position in the list of Pok ́emon belonging to the Entries object. i aready have a pokemon class with enum for types, move class and move class setup and working it's just this one part. here is the text file 1,Bulbasaur,45,49,[Grass,Poison],Fire,Water,[[tackle,40,40,100],[vine whip,45,45,100],[growl,10,10,100]] 2,Ivysaur,60,63,[Grass,Poison],Fire,Water,[[leech seed,45,45,90],[razor leaf,55,55,95],[growl,10,10,100]] 3,Venusaur,80,83,[Grass,Poison],Fire,Water,[[tackle,40,40,100],[leech seed,45,45,90],[razor leaf,55,55,95],[growl,10,10,100]] 4,Charmander,39,43,[Fire],Water,Fire,[[growl,10,10,100],[fire fang,65,65,95]]…
arrow_forward
1.In a loop, fill an array for up to 50 even numbers and a set for up to 50 odd numbers. Use the merge() algorithm to merge these containers into a vector. Display the vector contents to show that all went well.
2. Write a program that copies a source file of integers to a destination file, using stream iterators. The user should supply both source and destination filenames to the program. You can use a while loop approach and a list container with integer data type. Within the loop, read each integer value from the input iterator and add the value to the list container by using push_back() function, then increment the input iterator.Finally, use an output iterator to write the list to the destination file
arrow_forward
Create class Test in a file named Test.java. This class contains a main program that
performs the following actions:
Instantiate a doubly linked list.
Insert strings “a”, “b”, and “c” at the head of the list using three Insert() operations. The state of the list is now [“c”, “b”, “a”].
Set the current element to the second-to-last element with a call to Tail() followed by a call to Previous()Then insert string “d”. The state of the list is now [“c”, “d”, “b”, “a”].
Set the current element to past-the-end with a call to Tail() followed by a call to Next(). Then insert string “e”. The state of the list is now [“c”, “d”, “b”, “a”, “e”] .
Print the list with a call to Print() and verify that the state of the list is correct.
arrow_forward
python exercise:
Write a Python function called calculate_average that takes a list of numbers as input and returns the average of the numbers in the list. If the input list is empty, the function should raise an EmptyListError exception.
Handle the case where the input list is empty by raising a custom EmptyListError exception. This exception should have an error message that says "Cannot calculate average of an empty list".
arrow_forward
1. In a loop, fill an array for up to 50 even numbers and a set for up to 50 odd numbers. Use the merge() algorithm to merge these containers into a vector. Display the vector contents to show that all went well. (In C++ language)
2. Write a program that copies a source file of integers to a destination file, using stream iterators. The user should supply both source and destination filenames to the program. You can use a while loop approach and a list container with integer data type. Within the loop, read each integer value from the input iterator and add the value to the list container by using push_back() function, then increment the input iterator.Finally, use an output iterator to write the list to the destination file. (In C++ language)
arrow_forward
Q1:Write a function that returns a new list by eliminating theduplicate values in the list. Use the following function header:def eliminateDuplicates(lst):Write a test program that reads in a list of integers, invokes the function,and displays the result. Here is the sample run of the program
Enter ten numbers: 2 3 2 1 6 3 4 5 2The distinct numbers are: 1 2 3 6 4 5
Q2:Write a program that reads in your Q1 Pythonsource code file and counts the occurrence of each keyword in the file.Your program should prompt the user to enter the Python source code filename.
This is the Q1: code:
def eliminateDuplictes(lst):newlist = [] search = set() for i in lst: if i not in search: newlist.append(i) search.add(i) return newlist
a=[] n= int(input("Enter the Size of the list:")) for x in range(n):item=input("\nEnter element " + str(x+1) + ":") a.append(item)
result = eliminateDuplictes(a)
print('\nThe distinct numbers are: ',"%s" % (' '.join(result)))
arrow_forward
Add the following operation to the class orderedLinkedList:
void mergeLists(orderedLinkedList<Type> &list1, orderedLinkedList<Type> &list2); //This function creates a new list by merging the //elements of list1 and list2. //Postcondition: first points to the merged list // list1 and list2 are empty
Consider the following statements:
orderedLinkedList<int> newList; orderedLinkedList<int> list1; orderedLinkedList<int> list2;
Suppose list1 points to the list with the elements 2 6 7, and list2 points to the list with the elements 3 5 8. The statement
newList.mergeLists(list1, list2);
creates a new linked list with the elements in the order 2 3 5 6 7 8, and the object newList points to this list. Also, after the preceding statement executes, list1 and list2 are empty.
2. Write the definition of the function template mergeLists to implement the operation mergeLists.
arrow_forward
Filename: runlength_decoder.py
Starter code for data: hw4data.py Download hw4data.py
Download hw4data.pyYou must provide a function named decode() that takes a list of RLE-encoded values as its single parameter, and returns a list containing the decoded values with the “runs” expanded. If we were to use Python annotations, the function signature would look similar to this:def decode(data : list) -> list:
Run-length encoding (RLE) is a simple, “lossless” compression scheme in which “runs” of data. The same value in consecutive data elements are stored as a single occurrence of the data value, and a count of occurrences for the run.
For example, using Python lists, the initial list:
[‘P’,‘P’,‘P’,‘P’,‘P’,‘Y’,‘Y’,‘Y’,‘P’,‘G’,‘G’]
would be encoded as:
['P', 5, 'Y', 3, ‘P’, 1, ‘G’, 2]
So, instead of using 11 “units” of space (if we consider the space for a character/int 1 unit), we only use 8 “units”. In this small example, we don’t achieve much of a savings (and indeed the…
arrow_forward
45-
Which of the following statements about lists in Python is true?
a.
Items within a list are unordered.
b.
A list can grow in size as items are added to it.
c.
All items in a list must have the same type.
d.
Items within a list must be unique.
e.
Items can only be added to a list by creating a new list object.
f.
Lists can only contain items which have a primitive type.
arrow_forward
Implement the following function using C++
remove_redundant()
This method will clear out the duplicate numbers in your linked list. It is also imperative that you will use an instance of this Linked List class to hold the numbers that you have already checked and use the methods in this instance. In the example 10 -> 10 -> 20 -> 30 -> 10, calling remove_redundant() will remove the other 2 10's that occurred later, hereby making the list 10 -> 20 -> 30. Limitation: You cannot have a nested loop here nor use methods that employ loops as the performance of our linked list will suffer.
arrow_forward
11.8 LAB: Dates
Complete the code to implement the following operations:
Complete read_date():
Read an input string representing a date in the format yyyy-mm-dd.
Create a date object from the input string.
Return the date object.
Call read_date() to read four (unique) date objects and store the date objects in a list.
Call sorted() to sort the list of date objects, earliest first. Store the sorted dates in a new list.
Output the sorted_dates, in the format mm/dd/yy.
Hint: Use strftime() to format the date outputs. (See resource below.)
Output the number of days between the last two dates in the sorted list as a positive number.
Output the date that is 3 weeks from the most recent date in the format "July 4, 1776".
Hint: Use timedelta() to set a duration of time for the arithmetic on date objects. (See resources below.)Ex: timedelta(days=50, seconds=27, hours=8, weeks=2) will define a duration of 50 days + 27 seconds + 8 hours + 2 weeks.
Output the full name of the day…
arrow_forward
2. Searches for any number within the sorted list using Binary search. Searches for any number that is not exist in the sorted list. Use exception handling to print out the corresponding error message
arrow_forward
C7 Write a program that will take an integer array(A) and an integer value(v)as input and do the following task
- If the value is present in the list,delete all the elements present before the value- if The value is not present in the list, enqueue it after 1st even number.//Comment per condition Must and explained the enqueue and code part with screenshot.I will up vote your answer immediatly. Kindly provide correct answer.
arrow_forward
Python function getData(fname:str)->ndarray that inputs data from a file into a list, then converts the list to a NumPy array of integer values and returns the array.
The text file (fname) contains:
integer numbers, one per line
numbers are in the range 0 <= n <= 99
r * c (rows * columns) quantity of numbers, where c = r + 1
Example:
15
22
65
4
78
91
7
35
60
16
99
12
==
[[15 22 65 4]
[78 91 7 35]
[60 16 99 12]]
arrow_forward
A. Create function getData(fname:str)->ndarray that inputs data from a file into a list, then converts the list to a NumPy array of integer values and returns the array.
The text file (fname) contains:
integer numbers, one per line
numbers are in the range 0 <= n <= 99
r * c (rows * columns) quantity of numbers, where c = r + 1.
Sample follows:
15
22
65
4
78
91
7
35
60
16
99
12
The function will convert the list of numbers into a 2-dimensional NumPy array with r rows and c columns. Sample follows:
[[15 22 65 4]
[78 91 7 35]
[60 16 99 12]]
B. Create a function getSum(arr:ndarray)->int that returns the sum of all the elements within a 2-dimensional array.
C. For the array example above, a sum of 504 is returned by getSum(arr).
D. The mathematical functions available for NumPy arrays may not be used. Determine the sum by iterating over all the elements of the array.
arrow_forward
The function last_words in python takes one parameter, fname, the name of a text file, and returns a list containing the last word from each line of the file. For example, if the file contents are:
apples are red bananas are yellow limes are green
then the list ["red", "yellow", "green"] should be returned.
NOTE: You may assume the file will contain no blank lines.
BIG HINT: If line is a string representing a line of text (inside a for loop!), then L = line.split() creates a list of the words in the line.
For example:
Test
Result
L = last_words("wordlist1.txt") print(L)
['happy', 'sad', 'angsty', 'euphoric', 'maudlin']
arrow_forward
In Python please:
7.9 LAB: Sorting TV Shows (dictionaries and lists)
Write a program that first reads in the name of an input file and then reads the input file using the file.readlines() method. The input file contains an unsorted list of number of seasons followed by the corresponding TV show. Your program should put the contents of the input file into a dictionary where the number of seasons are the keys, and a list of TV shows are the values (since multiple shows could have the same number of seasons).
Sort the dictionary by key (least to greatest) and output the results to a file named output_keys.txt, separating multiple TV shows associated with the same key with a semicolon (;). Next, sort the dictionary by values (alphabetical order), and output the results to a file named output_titles.txt.
Ex: If the input is:
file1.txt
and the contents of file1.txt are:
20
Gunsmoke
30
The Simpsons
10
Will & Grace
14
Dallas
20
Law & Order
12
Murder, She Wrote
the file…
arrow_forward
C++ The People class holds an unsorted list of humans. Initially, this list is empty. Upon inserting a Human object, it is added to the array at the next available position and the position value in incremented. If the position value is equal to the size value, do not insert the Human. Instead, throw an exception that your driver can catch to print an error that the People object is full.
Create a People class with the following features:
Each People object has a statically allocated array of Human objects.
Size attribute representing the maximum size of the array.
Position attribute representing the next free position in the array.
Default constructor with no arguments.
Search method that takes a string argument and returns a Human object. This method returns the first Human object with a name matching the string argument. In other words, pass the name of a Human as a string, search the list of humans for one with that name. If found, return this human. Else throw an…
arrow_forward
In C++
Define a class called textLines that will be used to store a list of lines of text (each line can be specified as a string).
Use a dynamic array to store the list. In addition, you should have a private data member that specifies the length of the list.
Create a constructor that takes a file name as parameter, and fills up the list with lines from the file. Make sure that you set the dynamic array to expand large enough to hold all the lines from the file. Also, create a constructor that takes an integer parameter that sets the size of an empty list.
Write member functions to:
remove and return the last line from the list
add a new line onto the end of the list, if there is room for it, otherwise print a message and expand the array
empty the entire list
return the number of lines still on the list
take two lists and return one combined list (with no duplicates)
copy constructor to support deep copying
remember the destructor!
arrow_forward
Word FrequencyWrite a python program that reads the contents of a text file. The program should create a dictio-nary inwhich the keys are the individual words found in the file and the values are the number of timeseach word appears. For example, if the word “the” appears 128 times, the dictionary wouldcontain an element with 'the' as the key and 128 as the value. The program should eitherdisplay the frequency of each word or create a second file containing a list of each word and itsfrequency.
arrow_forward
Having trouble with creating the InsertAtEnd function in the ItemNode.h file below.
" // TODO: Define InsertAtEnd() function that inserts a node // to the end of the linked list"
Given main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node.
Ex. if the input is:
4
Kale
Lettuce
Carrots
Peanuts
-------------------------------------------------------
main.cpp
--------------------------------------------------------
#include "ItemNode.h"
int main() { ItemNode *headNode; // Create intNode objects ItemNode *currNode; ItemNode *lastNode;
string item; int i; int input;
// Front of nodes list headNode = new ItemNode(); lastNode = headNode;
cin >> input;
for (i = 0; i < input; i++) { cin >> item;…
arrow_forward
1-Let the list have a head and a tail. That is, a pointer (have a marker) to both the beginning (first Node) of the list and the last Node. What process does Tail facilitate?
2-insert(int index, int element): adds this element to the index position. For example, if index is 4, it adds this element between index 3 and 4 in the list. The size of the list has increased by one.
3-append(int elem): Adds the element to the end of the list. The size of the list has increased by one.
4-get(int index): Returns the element at the index position of the list, no change in the list.
5-remove(int index): Returns the element at the index position of the list. This element is removed from the list and the list size is reduced by one.
6-findMin(): returns the index of the smallest number in the list.
7-findMax(): returns the index of the largest number in the list.
8-search(int elem): searches elem in the list. It returns -1 when you can't find elem's index when you find it.
9-ToArray(): Return an…
arrow_forward
1-Let the list have a head and a tail. That is, a pointer (have a marker) to both the beginning (first Node) of the list and the last Node. What process does Tail facilitate?
2-insert(int index, int element): adds this element to the index position. For example, if index is 4, it adds this element between index 3 and 4 in the list. The size of the list has increased by one.
3-append(int elem): Adds the element to the end of the list. The size of the list has increased by one.
4-get(int index): Returns the element at the index position of the list, no change in the list.
5-remove(int index): Returns the element at the index position of the list. This element is removed from the list and the list size is reduced by one.
6-findMin(): returns the index of the smallest number in the list.
7-findMax(): returns the index of the largest number in the list.
8-search(int elem): searches elem in the list. It returns -1 when you can't find elem's index when you find it.
9-ToArray(): Return an…
arrow_forward
1-Let the list have a head and a tail. That is, a pointer (have a marker) to both the beginning (first Node) of the list and the last Node. What process does Tail facilitate?
2-insert(int index, int element): adds this element to the index position. For example, if index is 4, it adds this element between index 3 and 4 in the list. The size of the list has increased by one.
3-append(int elem): Adds the element to the end of the list. The size of the list has increased by one.
4-get(int index): Returns the element at the index position of the list, no change in the list.
5-remove(int index): Returns the element at the index position of the list. This element is removed from the list and the list size is reduced by one.
6-findMin(): returns the index of the smallest number in the list.
7-findMax(): returns the index of the largest number in the list.
8-search(int elem): searches elem in the list. It returns -1 when you can't find elem's index when you find it.
9-ToArray(): Return an…
arrow_forward
11.11 LAB: Dates
Complete the code to implement the following operations:
Complete read_date():
Read an input string representing a date in the format yyyy-mm-dd.
Create a date object from the input string.
Return the date object.
Call read_date() to read four (unique) date objects and store the date objects in a list.
Call sorted() to sort the list of date objects, earliest first. Store the sorted dates in a new list.
Output the sorted_dates, in the format mm/dd/yy.
Hint: Use strftime() to format the date outputs. (See resource below.)
Output the number of days between the last two dates in the sorted list as a positive number.
Output the date that is 3 weeks from the most recent date in the format "July 4, 1776".
Hint: Use timedelta() to set a duration of time for the arithmetic on date objects. (See resources below.)Ex: timedelta(days=50, seconds=27, hours=8, weeks=2) will define a duration of 50 days + 27 seconds + 8 hours + 2 weeks.
Output the full name of the day…
arrow_forward
Time Write a Python script that extracts and prints a single record from an input file in which organized by line. Each line contains the name of a person (possibly containing multiple followed by the year of his birth. Abbas ibn Firnas ibn Wirda: 809 Muhammad ibn Musa al - Khwarizmi: 780 Abu Al - Walid Muhammad Ibn Ahmad Rushd: 1126 The program uses function extract DataRecord with the specification: @param infile the input text file object @return pants a list containing the name (string) in the first element and the year of bir the second element. If the end of file was reached, an empty list is returned
arrow_forward
how can i remove the spaces at the end of each line in my code ?
code:
def load_course(course_name):try:file = open(course_name+'.txt')return file.readlines()except FileNotFoundError as e:return None
def word_wrap_text(text,lineWidth):words = list(text.split())linelen = 0lines = []line = ''for word in words:if linelen + len(word) > lineWidth:lines.append(line)line = ''line += word + ' 'linelen = len(line)if line !='':lines.append(line)return '\n'.join(lines)
def enhanced_word_wrap_text(text,lineWidth):words = list(text.split())linelen = 0lines = []line = ''for word in words:if linelen + len(word) + 1 > lineWidth:lines.append(line + word[:lineWidth-linelen])line = word[lineWidth-linelen:] + ' 'else:line += word + ' 'linelen = len(line)if line != '':lines.append(line)return '\n'.join(lines)
lineWidth = int(input(""))
while True:course = input("")if course == 'q':breaklines = load_course(course)if lines == None:print('Unable to load course information for course',course)else:for…
arrow_forward
PYTHON 3
def get_graded(infolist): """ param: infolist - a list that contains dictionaries
Each dictionary in the infolist is supposed to have the following string keys: - category - the name of each grade category (string) - weight - the percentage weight of the category (float) - grades - a list of numeric grades for each assignment If no grades are stored for a category, the "grades" item will store an empty list.
return: The function returns a new (nested) list, which contains the extracted grades for each category. If the input is an empty list, the function returns an empty list. """
arrow_forward
Java programming
1. Write the code that will read the inventory information from a file, populate the object, and populate a linked list with all the inventory objects. Then find a particular item in the linked list based on user input and display the information for that item.
Inventory inventory = new Inventory ();
Write the line of code to find an inventory object in the linked list using the property for InventoryID
arrow_forward
program7_1.pyWrite a Python program that creates a list of your friends.
Start with an empty list and then use a while loop to add friends to the list. This loop should repeat until you leave a name blank.
Report the size of your list.
You forgot a good friend. If she is not in the list (let code decide), she should be inserted at the start of the list.
Use a for loop to display the names of your friends on one line, separated by spaces.
A friend has moved away. Remove this friend by name from the list.
Sort the list in alphabetical order and use another loop to display your friends, sorted.
Sample OutputEnter the first name of a friend or ENTER to quit PennyEnter the first name of a friend or ENTER to quit LennyEnter the first name of a friend or ENTER to quit KennyEnter the first name of a friend or ENTER to quit DennyEnter the first name of a friend or ENTER to quit BennyEnter the first name of a friend or ENTER to quitYou made a list of 5 friends.OOPS, you forgot to enter a very…
arrow_forward
7.9 LAB: Sorting TV Shows (dictionaries and lists)
Write a program that first reads in the name of an input file and then reads the input file using the file.readlines() method. The input file contains an unsorted list of number of seasons followed by the corresponding TV show. Your program should put the contents of the input file into a dictionary where the number of seasons are the keys, and a list of TV shows are the values (since multiple shows could have the same number of seasons).
Sort the dictionary by key (least to greatest) and output the results to a file named output_keys.txt, separating multiple TV shows associated with the same key with a semicolon (;). Next, sort the dictionary by values (alphabetical order), and output the results to a file named output_titles.txt.
Ex: If the input is:
file1.txt
and the contents of file1.txt are:
20
Gunsmoke
30
The Simpsons
10
Will & Grace
14
Dallas
20
Law & Order
12
Murder, She Wrote
the file output_keys.txt should…
arrow_forward
6.44 LAB: Contact list (JAVA)
A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program in java that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings), separated by a comma. That list is followed by a name, and your program should output the phone number associated with that name. Output "None" if name is not found. Assume that the list will always contain less than 20 word pairs.
Ex: If the input is:
3 Joe,123-5432 Linda,983-4123 Frank,867-5309 Frank
the output is:
867-5309
Your program must define and call the following method. The return value of getPhoneNumber() is the phone number associated with the specific contact name.public static String getPhoneNumber(String[] nameArr, String[] phoneNumberArr, String contactName, int arraySize)
Hint: Use two arrays: One for…
arrow_forward
.......Python Code.............
Python file:
# Write a function that takes an open hockey file and returns a list of tuples,# where each tuple refers to a team name and that team's maximum number of wins in any year.
from typing import TextIO
def teams_and_most_wins(f: TextIO) -> list[tuple[str, int]]: # Return a list of tuples where each tuple stores a team name and # that team's maximum number of wins in any year.
# DO CODE HERE ... Use this
'''f is an open hockey file.Each year has 4 digits and each number of wins has 2 digits.Every team should appear in exactly one of the tuples.'''
.......File.............
Each line of a hockey file contains a four-digit year, a team name, and a two-digit number of wins.Here is one such file, hockey.txt:>>>2003maple leafs452020maple leafs351995senators181996maple leafs302000senators482012canadiens48>>>The first line of the file, for example, says that in 2003 (year), the maple leafs (team…
arrow_forward
3) in python
Copy the Plain Text UTF-8 version of Bram Sroker’s Dracula from the site:http://www.gutenberg.org/ebooks/345 into a text document called ‘dracula.txt’. Write pythoncode that reads the text document and counts the unique words and stores the words and countsinto a dictionary. Write the words and counts into a text file called ‘dracula words.txt’ by usingthe Python file handle method seen in class. Generate a list called ‘sorted counts’ that containsthe value and key pairs as tuples that are sorted in descending order based on the frequencies ofthe words. For example, it should be in the form:[(7423, ‘the’),(5640, ‘and’),(4499, ‘I’),(4462, ‘to’),(3673, ‘of’),(2891, ‘a’),(2384, ‘in’),(ect.)
Here, the word ‘the’ occurs the most frequently. Output the top 10 most frequently occurringwords. How often does the word ‘Dracula’ come up in the entire Novel?Hint: use list comprehension. For the dictionary ‘counts’ the command ‘counts.items()’ returns anobject of type dict items that…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
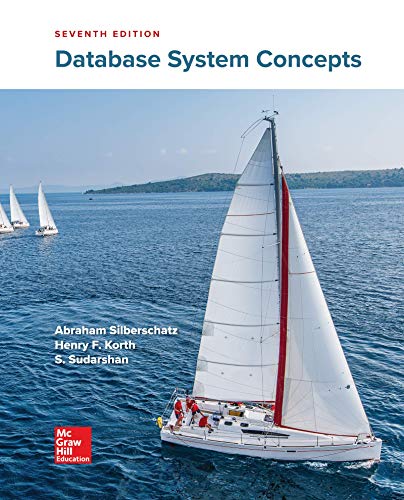
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
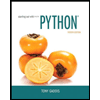
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
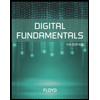
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
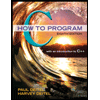
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
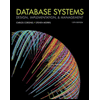
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
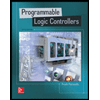
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- a. Add the following operation to the class orderedLinkedList:void mergeLists(orderedLinkedList<Type> &list1,orderedLinkedList<Type> &list2);//This function creates a new list by merging the//elements of list1 and list2.//Postcondition: first points to the merged list// list1 and list2 are emptyConsider the following statements:orderedLinkedList<int> newList;orderedLinkedList<int> list1;orderedLinkedList<int> list2;Suppose list1 points to the list with the elements 2 6 7, and list2 points to the list with the elements 3 5 8. The statement newList.mergeLists(list1, list2); creates a new linked list with the elements in the order 2 3 5 6 7 8, and the object newList points to this list. Also, after the preceding statement executes, list1 and list2 are empty. b. Write the definition of the function template mergeLists to implement the operation mergeLists.arrow_forwardvoid Pokedex::wakeupProfessorJimi(std::string) − populates the dynamic array of Entries with data from a valid file path, the function should open the file and use the add (Pokemon*, int) function to insert a Pok ́emon into the correct position in the dynamic array of Entries. Once the position is determined, the Pok ́emon should be inserted into the corresponding position in the list of Pok ́emon belonging to the Entries object. i aready have a pokemon class with enum for types, move class and move class setup and working it's just this one part. here is the text file 1,Bulbasaur,45,49,[Grass,Poison],Fire,Water,[[tackle,40,40,100],[vine whip,45,45,100],[growl,10,10,100]] 2,Ivysaur,60,63,[Grass,Poison],Fire,Water,[[leech seed,45,45,90],[razor leaf,55,55,95],[growl,10,10,100]] 3,Venusaur,80,83,[Grass,Poison],Fire,Water,[[tackle,40,40,100],[leech seed,45,45,90],[razor leaf,55,55,95],[growl,10,10,100]] 4,Charmander,39,43,[Fire],Water,Fire,[[growl,10,10,100],[fire fang,65,65,95]]…arrow_forward1.In a loop, fill an array for up to 50 even numbers and a set for up to 50 odd numbers. Use the merge() algorithm to merge these containers into a vector. Display the vector contents to show that all went well. 2. Write a program that copies a source file of integers to a destination file, using stream iterators. The user should supply both source and destination filenames to the program. You can use a while loop approach and a list container with integer data type. Within the loop, read each integer value from the input iterator and add the value to the list container by using push_back() function, then increment the input iterator.Finally, use an output iterator to write the list to the destination filearrow_forward
- Create class Test in a file named Test.java. This class contains a main program that performs the following actions: Instantiate a doubly linked list. Insert strings “a”, “b”, and “c” at the head of the list using three Insert() operations. The state of the list is now [“c”, “b”, “a”]. Set the current element to the second-to-last element with a call to Tail() followed by a call to Previous()Then insert string “d”. The state of the list is now [“c”, “d”, “b”, “a”]. Set the current element to past-the-end with a call to Tail() followed by a call to Next(). Then insert string “e”. The state of the list is now [“c”, “d”, “b”, “a”, “e”] . Print the list with a call to Print() and verify that the state of the list is correct.arrow_forwardpython exercise: Write a Python function called calculate_average that takes a list of numbers as input and returns the average of the numbers in the list. If the input list is empty, the function should raise an EmptyListError exception. Handle the case where the input list is empty by raising a custom EmptyListError exception. This exception should have an error message that says "Cannot calculate average of an empty list".arrow_forward1. In a loop, fill an array for up to 50 even numbers and a set for up to 50 odd numbers. Use the merge() algorithm to merge these containers into a vector. Display the vector contents to show that all went well. (In C++ language) 2. Write a program that copies a source file of integers to a destination file, using stream iterators. The user should supply both source and destination filenames to the program. You can use a while loop approach and a list container with integer data type. Within the loop, read each integer value from the input iterator and add the value to the list container by using push_back() function, then increment the input iterator.Finally, use an output iterator to write the list to the destination file. (In C++ language)arrow_forward
- Q1:Write a function that returns a new list by eliminating theduplicate values in the list. Use the following function header:def eliminateDuplicates(lst):Write a test program that reads in a list of integers, invokes the function,and displays the result. Here is the sample run of the program Enter ten numbers: 2 3 2 1 6 3 4 5 2The distinct numbers are: 1 2 3 6 4 5 Q2:Write a program that reads in your Q1 Pythonsource code file and counts the occurrence of each keyword in the file.Your program should prompt the user to enter the Python source code filename. This is the Q1: code: def eliminateDuplictes(lst):newlist = [] search = set() for i in lst: if i not in search: newlist.append(i) search.add(i) return newlist a=[] n= int(input("Enter the Size of the list:")) for x in range(n):item=input("\nEnter element " + str(x+1) + ":") a.append(item) result = eliminateDuplictes(a) print('\nThe distinct numbers are: ',"%s" % (' '.join(result)))arrow_forwardAdd the following operation to the class orderedLinkedList: void mergeLists(orderedLinkedList<Type> &list1, orderedLinkedList<Type> &list2); //This function creates a new list by merging the //elements of list1 and list2. //Postcondition: first points to the merged list // list1 and list2 are empty Consider the following statements: orderedLinkedList<int> newList; orderedLinkedList<int> list1; orderedLinkedList<int> list2; Suppose list1 points to the list with the elements 2 6 7, and list2 points to the list with the elements 3 5 8. The statement newList.mergeLists(list1, list2); creates a new linked list with the elements in the order 2 3 5 6 7 8, and the object newList points to this list. Also, after the preceding statement executes, list1 and list2 are empty. 2. Write the definition of the function template mergeLists to implement the operation mergeLists.arrow_forwardFilename: runlength_decoder.py Starter code for data: hw4data.py Download hw4data.py Download hw4data.pyYou must provide a function named decode() that takes a list of RLE-encoded values as its single parameter, and returns a list containing the decoded values with the “runs” expanded. If we were to use Python annotations, the function signature would look similar to this:def decode(data : list) -> list: Run-length encoding (RLE) is a simple, “lossless” compression scheme in which “runs” of data. The same value in consecutive data elements are stored as a single occurrence of the data value, and a count of occurrences for the run. For example, using Python lists, the initial list: [‘P’,‘P’,‘P’,‘P’,‘P’,‘Y’,‘Y’,‘Y’,‘P’,‘G’,‘G’] would be encoded as: ['P', 5, 'Y', 3, ‘P’, 1, ‘G’, 2] So, instead of using 11 “units” of space (if we consider the space for a character/int 1 unit), we only use 8 “units”. In this small example, we don’t achieve much of a savings (and indeed the…arrow_forward
- 45- Which of the following statements about lists in Python is true? a. Items within a list are unordered. b. A list can grow in size as items are added to it. c. All items in a list must have the same type. d. Items within a list must be unique. e. Items can only be added to a list by creating a new list object. f. Lists can only contain items which have a primitive type.arrow_forwardImplement the following function using C++ remove_redundant() This method will clear out the duplicate numbers in your linked list. It is also imperative that you will use an instance of this Linked List class to hold the numbers that you have already checked and use the methods in this instance. In the example 10 -> 10 -> 20 -> 30 -> 10, calling remove_redundant() will remove the other 2 10's that occurred later, hereby making the list 10 -> 20 -> 30. Limitation: You cannot have a nested loop here nor use methods that employ loops as the performance of our linked list will suffer.arrow_forward11.8 LAB: Dates Complete the code to implement the following operations: Complete read_date(): Read an input string representing a date in the format yyyy-mm-dd. Create a date object from the input string. Return the date object. Call read_date() to read four (unique) date objects and store the date objects in a list. Call sorted() to sort the list of date objects, earliest first. Store the sorted dates in a new list. Output the sorted_dates, in the format mm/dd/yy. Hint: Use strftime() to format the date outputs. (See resource below.) Output the number of days between the last two dates in the sorted list as a positive number. Output the date that is 3 weeks from the most recent date in the format "July 4, 1776". Hint: Use timedelta() to set a duration of time for the arithmetic on date objects. (See resources below.)Ex: timedelta(days=50, seconds=27, hours=8, weeks=2) will define a duration of 50 days + 27 seconds + 8 hours + 2 weeks. Output the full name of the day…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
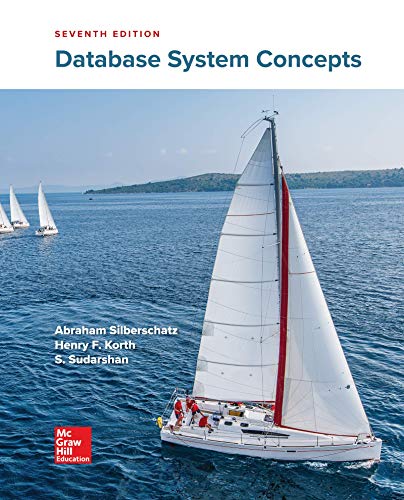
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
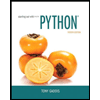
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
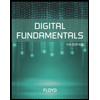
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
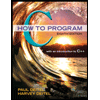
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
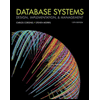
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
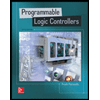
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education