Filename: runlength_decoder.py Starter code for data: hw4data.py Download hw4data.py Download hw4data.pyYou must provide a function named decode() that takes a list of RLE-encoded values as its single parameter, and returns a list containing the decoded values with the “runs” expanded. If we were to use Python annotations, the function signature would look similar to this: def decode(data : list) -> list: Run-length encoding (RLE) is a simple, “lossless” compression scheme in which “runs” of data. The same value in consecutive data elements are stored as a single occurrence of the data value, and a count of occurrences for the run. For example, using Python lists, the initial list: [‘P’,‘P’,‘P’,‘P’,‘P’,‘Y’,‘Y’,‘Y’,‘P’,‘G’,‘G’] would be encoded as: ['P', 5, 'Y', 3, ‘P’, 1, ‘G’, 2] So, instead of using 11 “units” of space (if we consider the space for a character/int 1 unit), we only use 8 “units”. In this small example, we don’t achieve much of a savings (and indeed the smaller your overall data size, the less opportunity for any compression algorithm to save much space) but imagine if we had a run of 30 or 40 P’s and 10 Y’s. The space savings begin to add up. Our RLE scheme for this assignment uses the format shown in the example above. Data is contained in a list such that the elements follow the pattern: Notice the data contained in our list is heterogeneous. In other words, it's NOT all the same type. strings and integers are interleaved in the list. Be sure to consider this as you're designing your solution. Do this: Write a program that decompresses the data included in the file starter file hw4data.py. IMPORTANT: Import hw4data.py, do NOT copy/paste the data! If you copy-paste and introduce a subtle data error due to "fat fingering" or some other mistake, your RLE program may not work correctly and you will lose points. The data is a Python file - import it as any other "outside asset" file (e.g. external functions) and proceed from there. There are six data values of increasing size and complexity: DATA0, DATA1, …DATA5. Your program must provide a function named decode(list) that takes a list of RLE-encoded values and returns a list containing the decoded values with the “runs” expanded. We will call your decode() function in our test suite, so name the function exactly as specified, and make sure it returns a Python list. Optional: We're using some fun compressed data, but it's all textual. If you want to actually see the original information, you can convert the decompressed (decoded) list into one single string value, then display that string on the screen using the print() statement. Again, this step is optional and for your personal enjoyment to view the data. Given the example data discussed above, the screen output would be something like this: Notes WE do not want to see your decoded data printed out. IF you perform this optional step be sure to keep this separate from the core functionality you're submitting for your assignment. WARNING: Do NOT use your decode() function for optional actions described above. You are free to create other helper functions to convert and display the decompressed data. You will lose points if your decode() function does more than the specified decompression algorithm. You can assume “good” data and it conforms to the RLE scheme described without any missing elements. What to submit: Your solution in a file named: runlength_decoder.py, which contains the function decode() as specified above. You may include other files with any “helper” functions you’ve written. Documentation/comments in runlength_decoder.py should indicate any additional helper files you’ve included
-
- Filename: runlength_decoder.py
- Starter code for data: hw4data.py Download hw4data.py
- Download hw4data.pyYou must provide a function named decode() that takes a list of RLE-encoded values as its single parameter, and returns a list containing the decoded values with the “runs” expanded. If we were to use Python annotations, the function signature would look similar to this:
def decode(data : list) -> list:
Run-length encoding (RLE) is a simple, “lossless” compression scheme in which “runs” of data. The same value in consecutive data elements are stored as a single occurrence of the data value, and a count of occurrences for the run.
For example, using Python lists, the initial list:
[‘P’,‘P’,‘P’,‘P’,‘P’,‘Y’,‘Y’,‘Y’,‘P’,‘G’,‘G’]
would be encoded as:
['P', 5, 'Y', 3, ‘P’, 1, ‘G’, 2]
So, instead of using 11 “units” of space (if we consider the space for a character/int 1 unit), we only use 8 “units”. In this small example, we don’t achieve much of a savings (and indeed the smaller your overall data size, the less opportunity for any compression
algorithm to save much space) but imagine if we had a run of 30 or 40 P’s and 10 Y’s. The space savings begin to add up.Our RLE scheme for this assignment uses the format shown in the example above. Data is contained in a list such that the elements follow the pattern:
Notice the data contained in our list is heterogeneous. In other words, it's NOT all the same type. strings and integers are interleaved in the list. Be sure to consider this as you're designing your solution.
Do this:
- Write a program that decompresses the data included in the file starter file hw4data.py.
IMPORTANT: Import hw4data.py, do NOT copy/paste the data! If you copy-paste and introduce a subtle data error due to "fat fingering" or some other mistake, your RLE program may not work correctly and you will lose points. The data is a Python file - import it as any other "outside asset" file (e.g. external functions) and proceed from there. - There are six data values of increasing size and complexity: DATA0, DATA1, …DATA5. Your program must provide a function named decode(list) that takes a list of RLE-encoded values and returns a list containing the decoded values with the “runs” expanded. We will call your decode() function in our test suite, so name the function exactly as specified, and make sure it returns a Python list.
Optional:
We're using some fun compressed data, but it's all textual. If you want to actually see the original information, you can convert the decompressed (decoded) list into one single string value, then display that string on the screen using the print() statement. Again, this step is optional and for your personal enjoyment to view the data. Given the example data discussed above, the screen output would be something like this:
Notes-
WE do not want to see your decoded data printed out. IF you perform this optional step be sure to keep this separate from the core functionality you're submitting for your assignment.
WARNING: Do NOT use your decode() function for optional actions described above. You are free to create other helper functions to convert and display the decompressed data. You will lose points if your decode() function does more than the specified decompression algorithm. -
You can assume “good” data and it conforms to the RLE scheme described without any missing elements.
What to submit:
-
- Your solution in a file named: runlength_decoder.py, which contains the function decode() as specified above.
- You may include other files with any “helper” functions you’ve written. Documentation/comments in runlength_decoder.py should indicate any additional helper files you’ve included
![[ 'P', 5, 'Y', 3,...]
↑
X
value
occurrence
in run](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe4ce8336-35c9-4006-8537-7cebe9e23665%2Fc73a3d3c-eee9-4461-ba19-6d47910a7ea8%2Fbrtde15_processed.jpeg&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

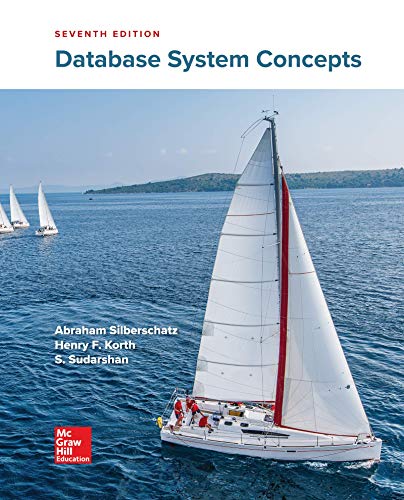
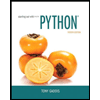
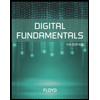
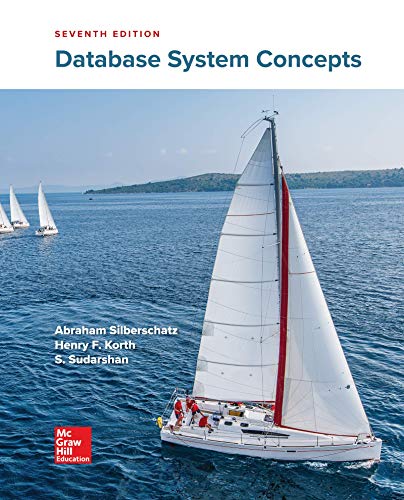
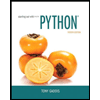
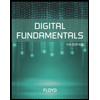
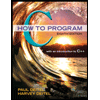
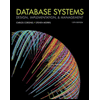
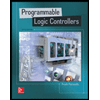