Write a program that plays a number guessing game with a Human user. The Human user will think of a number between LOWER_LIMIT and UPPER_LIMIT, inclusive. (LOWER_LIMIT and UPPER_LIMIT will be global constants, typically 1 and 100, but your program must still work if they are set to something different. You may assume that LOWER_LIMIT < UPPER_LIMIT.) The program will make guesses and the user will tell the program to guess higher or lower. A sample run of the program might look like this: Ready to play (y/n)? y Think of a number between 1 and 100. My guess is 50. Enter 'l' if your number is lower, 'h' if it is higher, 'c' if it is correct: h My guess is 75. Enter 'l' if your number is lower, 'h' if it is higher, 'c' if it is correct: h My guess is 88. Enter 'l' if your number is lower, 'h' if it is higher, 'c' if it is correct: 1 My guess is 81. Enter 'l' if your number is lower, 'h' if it is higher, 'c' if it is correct: c Great! Do you want to play again (y/n)? y Think of a number between 1 and 100. My guess is 50. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: 1 My guess is 25. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: h My guess is 37. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: c Great! Do you want to play again (y/n)? n The strategy that the program will use goes like this: Every time the program makes a guess it should guess the midpoint of the remaining possible values. Consider the first example above, in which the user has chosen the number 81: On the first guess, the possible values are 1 to 100. The midpoint is 50. The user responds by saying "higher" On the second guess the possible values are 51 to 100. The midpoint is 75. The user responds by saying "higher" On the third guess the possible values are 76 to 100. The midpoint is 88. The user responds by saying "lower" On the fourth guess the possible values are 76 to 87. The midpoint is 81. The user responds "correct"
Write a program that plays a number guessing game with a Human user. The Human user will think of a number between LOWER_LIMIT and UPPER_LIMIT, inclusive. (LOWER_LIMIT and UPPER_LIMIT will be global constants, typically 1 and 100, but your program must still work if they are set to something different. You may assume that LOWER_LIMIT < UPPER_LIMIT.) The program will make guesses and the user will tell the program to guess higher or lower. A sample run of the program might look like this: Ready to play (y/n)? y Think of a number between 1 and 100. My guess is 50. Enter 'l' if your number is lower, 'h' if it is higher, 'c' if it is correct: h My guess is 75. Enter 'l' if your number is lower, 'h' if it is higher, 'c' if it is correct: h My guess is 88. Enter 'l' if your number is lower, 'h' if it is higher, 'c' if it is correct: 1 My guess is 81. Enter 'l' if your number is lower, 'h' if it is higher, 'c' if it is correct: c Great! Do you want to play again (y/n)? y Think of a number between 1 and 100. My guess is 50. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: 1 My guess is 25. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: h My guess is 37. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: c Great! Do you want to play again (y/n)? n The strategy that the program will use goes like this: Every time the program makes a guess it should guess the midpoint of the remaining possible values. Consider the first example above, in which the user has chosen the number 81: On the first guess, the possible values are 1 to 100. The midpoint is 50. The user responds by saying "higher" On the second guess the possible values are 51 to 100. The midpoint is 75. The user responds by saying "higher" On the third guess the possible values are 76 to 100. The midpoint is 88. The user responds by saying "lower" On the fourth guess the possible values are 76 to 87. The midpoint is 81. The user responds "correct"
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter5: Repetition Statements
Section5.5: A Closer Look: Loop Programming Techniques
Problem 14E
Related questions
Question
Provide full C++ code

Transcribed Image Text:Write a program that plays a number guessing game with a Human user. The Human user will think of a number between LOWER_LIMIT and UPPER_LIMIT, inclusive. (LOWER_LIMIT and UPPER_LIMIT will be global constants, typically 1 and 100, but your program must still work if they are set to something
different. You may assume that LOWER_LIMIT < UPPER_LIMIT.) The program will make guesses and the user will tell the program to guess higher or lower.
A sample run of the program might look like this:
Ready to play (y/n)? y
Think of a number between 1 and 100.
My guess is 50. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: h
My guess is 75. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: h
My guess is 88. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: 1
My guess is 81. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: c
Great! Do you want to play again (y/n)? y
Think of a number between 1 and 100.
My guess is 50. Enter '1' if your number is
My guess is 25. Enter '1' if your number is
My guess is 37. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: c
Great! Do you want to play again (y/n)? n
The strategy that the program will use goes like this: Every time the program makes a guess it should guess the midpoint of the remaining possible values. Consider the first example above, in which the user has chosen the number 81:
On the first guess, the possible values are 1 to 100. The midpoint is 50.
The user responds by saying "higher"
On the second guess the possible values are 51 to 100. The midpoint is 75.
The user responds by saying "higher"
lower, 'h' if it is
lower, 'h' if it is
On the third guess the possible values are 76 to 100. The midpoint is 88.
The user responds by saying "lower"
On the fourth guess the possible values are 76 to 87. The midpoint is 81.
The user responds "correct"
Additional Requirements
int main() {
The purpose of the assignment is to practice writing functions. Although it would be possible to write the entire program in the main function, your solution should be heavily structured. Most of the point penalties given on this assignment will be for not following the instructions below carefully. The main
function must look exactly like this. Copy and paste this code into your file, and don't edit it:
char response;
cout << "Ready to play (y/n)? ";
cin>> response;
higher, 'c' if it is correct: 1
higher, 'c' if it is correct: h
while (response ==
'y') {
playOneGame ( ) ;
cout << "Great! Do you want to play again (y/n)? ";
cin >> response;
}
The playOneGame() function must implement a complete guessing game using the range LOWER_LIMIT to UPPER_LIMIT.
In addition, you must implement the following helper functions to be invoked inside your playOneGame() function:
void getUser ResponseToGuess(int guess, char& result)
The getUserResponseToGuess() function should prompt the user to enter 'h', 'I', or 'c' (as shown in the sample output). It should set its "result" parameter equal to whatever the user enters in response. It should do this ONE time, and should not do anything else. Note that printing the guess is part of the
prompt.
int getMidpoint (int low, int high)
The getMidpoint() function should accept two integers, and it should return the midpoint of the two integers. If there are two values in the middle of the range then you should consistently chose the smaller of the two.
![Additional Requirements
1) You must write a function for each hand type. Each function must accept a const int array that contains HAND_SIZE integers, each representing one of the HAND_SIZE cards in the hand, and must return "true" if the hand contains the cards indicated by the name of the function, "false" if it does not. The functions must have the following prototypes.
bool contains Pair (const int hand[ ]);
bool contains TwoPair (const int hand[ ]);
bool contains Three0faKind (const int hand[ ]);
bool contains Straight (const int hand[ ]);
bool contains FullHouse (const int hand []);
bool contains FourOfaKind (const int hand []);
Important! In the descriptions below, a pair is defined as exactly two of the same card. If there are more than two of the same card, that is not a pair. Similarly, a three-of-a-kind is defined as exactly three of the same card. If there are more than three of the same card, that is not a three-of-a-kind. However! Since there is no such hand as five-of-a-kind or
more, four-of-a-kind must return true if there are four or more of the same card.
// post:
returns true if and only if there are one or more pairs in the hand. Note that
// this function returns false if there are more than two of the same card (and no other pairs).
bool containsPair (const int hand[ ]);
// post: returns true if and only if there are two or more pairs in the hand.
bool
contains Two Pair (const int hand[ ]);
// post: returns true if and only if there are one or more three-of-a-kind's in the hand.
bool contains Three0faKind (const int hand[]);
// post: returns true if there are 5 consecutive cards in the hand.
bool
contains Straight (const int hand []);
// post: returns true if there is are one or more pairs and one or more three-of-a-kind's in the hand.
bool contains FullHouse (const int hand[]);
// post: returns true if there are one or more four-of-a-kind's in the hand.
bool contains FourOfaKind (const int hand[ ]);
Some examples:
A hand that contains three-of-a-kind and two other different cards (for example, 1, 1, 1, 2, 3) should return "false" for "containsPair()"
A hand that contains four-of-a-kind should return "false" for "containsPair()" and "contains ThreeOfaKind()"
A hand that contains a full-house should return "true" for contains ThreeOfa Kind() and containsPair().
A hand that contains two-pair should return "true" for containsPair().
Here is a table with some examples that I hope will help clear up any confusion. If there are additional hands that you are unsure about, please ask in the discussion, and I will consider adding rows to this table for further clarification.
hand
pair? two-pair? three-of-a-kind? full-house? four-of-a-kind? straight?
2, 2, 2, 3, 4
F
2, 3, 3, 3, 3
F
2, 2, 3, 3, 3
T
2, 2, 3, 3, 4
T
2, 2, 2, 3, 3, 3 F
9, 2, 4, 3, 6, 5 F
4, 2, 6, 8, 7, 5 F
●
F
int main() {
IF
F
}
T
F
F
F
T
F
T
F
T
F
F
I would suggest that before you submit your program you test it using each of the above examples.
2) You do not need to write a containsHighCard function. All hands contain a highest card. If you determine that a particular hand is not one of the better hand types, then know that it is a High Card hand.
3) Do not sort the cards in the hand. Also, do not make a copy of the hand and then sort that.
4) An important objective of this assignment is to have you practice creating excellent decomposition. Don't worry about efficiency on this assignment. Focus on excellent decomposition, which results in readable code. This is one of those programs where you can rush and get it done but end up with code that is really difficult to read, debug, modify, and
re-use. If you think about it hard, you can think of really helpful ways in which to combine the tasks that the various functions are performing. 5 extra credit points on this assignment will be awarded based on the following criteria: no function may have nested loops in it. If you need nested loops, the inner loop must be turned into a separate function,
hopefully in a way that makes sense and so that the separate function is general enough to be re-used by the other functions. Also, no function other than main() may have more than 5 lines of code. (This is counting declarations, but not counting the function header, blank lines, or lines that have only a curly brace on them.) In my solution I was able to create just 3
helper functions, 2 of which are used repeatedly by the various functions.
These additional criteria are intended as an extra challenge and may be difficult for many of you. If you can't figure it out, give it your best shot, but don't be too discouraged. It's just 5 points. And be sure to study the posted solution carefully.
F
F
T
F
F
F
F
Suggestions
1) I strongly suggest that you never compare cards with each other. This is often the instinctive direction that students go at first. For example, to find whether the hand contains a pair, you may be tempted to compare every card in the hand with every other card in the hand. I suggest that instead, you count how many of each card there are.
2) If you find that you are really struggling with even the containsPair() function, I suggest that you solve an even simpler problem first: write a function that returns true if the hand contains a pair of 2's (false otherwise). Just count how many 2's there are in the hand, and return true if there are exactly two of them (false otherwise).
3) Test these functions independently. Once you are sure that they all work, the program logic for the complete program will be fairly straightforward.
Here is code that tests a containsPair function:
int hand[] = {2, 5, 3, 2, 9};
if (containsPair (hand)) {
}
F
T
F
F
F
F
F
F
F
F
IF
F
IT
T
cout<<"contains a pair" << endl;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1bfda259-7429-4717-b69c-7067c249bba0%2F552aaa1b-a862-4500-80bc-91a8ee32c049%2F5t86uaq_processed.png&w=3840&q=75)
Transcribed Image Text:Additional Requirements
1) You must write a function for each hand type. Each function must accept a const int array that contains HAND_SIZE integers, each representing one of the HAND_SIZE cards in the hand, and must return "true" if the hand contains the cards indicated by the name of the function, "false" if it does not. The functions must have the following prototypes.
bool contains Pair (const int hand[ ]);
bool contains TwoPair (const int hand[ ]);
bool contains Three0faKind (const int hand[ ]);
bool contains Straight (const int hand[ ]);
bool contains FullHouse (const int hand []);
bool contains FourOfaKind (const int hand []);
Important! In the descriptions below, a pair is defined as exactly two of the same card. If there are more than two of the same card, that is not a pair. Similarly, a three-of-a-kind is defined as exactly three of the same card. If there are more than three of the same card, that is not a three-of-a-kind. However! Since there is no such hand as five-of-a-kind or
more, four-of-a-kind must return true if there are four or more of the same card.
// post:
returns true if and only if there are one or more pairs in the hand. Note that
// this function returns false if there are more than two of the same card (and no other pairs).
bool containsPair (const int hand[ ]);
// post: returns true if and only if there are two or more pairs in the hand.
bool
contains Two Pair (const int hand[ ]);
// post: returns true if and only if there are one or more three-of-a-kind's in the hand.
bool contains Three0faKind (const int hand[]);
// post: returns true if there are 5 consecutive cards in the hand.
bool
contains Straight (const int hand []);
// post: returns true if there is are one or more pairs and one or more three-of-a-kind's in the hand.
bool contains FullHouse (const int hand[]);
// post: returns true if there are one or more four-of-a-kind's in the hand.
bool contains FourOfaKind (const int hand[ ]);
Some examples:
A hand that contains three-of-a-kind and two other different cards (for example, 1, 1, 1, 2, 3) should return "false" for "containsPair()"
A hand that contains four-of-a-kind should return "false" for "containsPair()" and "contains ThreeOfaKind()"
A hand that contains a full-house should return "true" for contains ThreeOfa Kind() and containsPair().
A hand that contains two-pair should return "true" for containsPair().
Here is a table with some examples that I hope will help clear up any confusion. If there are additional hands that you are unsure about, please ask in the discussion, and I will consider adding rows to this table for further clarification.
hand
pair? two-pair? three-of-a-kind? full-house? four-of-a-kind? straight?
2, 2, 2, 3, 4
F
2, 3, 3, 3, 3
F
2, 2, 3, 3, 3
T
2, 2, 3, 3, 4
T
2, 2, 2, 3, 3, 3 F
9, 2, 4, 3, 6, 5 F
4, 2, 6, 8, 7, 5 F
●
F
int main() {
IF
F
}
T
F
F
F
T
F
T
F
T
F
F
I would suggest that before you submit your program you test it using each of the above examples.
2) You do not need to write a containsHighCard function. All hands contain a highest card. If you determine that a particular hand is not one of the better hand types, then know that it is a High Card hand.
3) Do not sort the cards in the hand. Also, do not make a copy of the hand and then sort that.
4) An important objective of this assignment is to have you practice creating excellent decomposition. Don't worry about efficiency on this assignment. Focus on excellent decomposition, which results in readable code. This is one of those programs where you can rush and get it done but end up with code that is really difficult to read, debug, modify, and
re-use. If you think about it hard, you can think of really helpful ways in which to combine the tasks that the various functions are performing. 5 extra credit points on this assignment will be awarded based on the following criteria: no function may have nested loops in it. If you need nested loops, the inner loop must be turned into a separate function,
hopefully in a way that makes sense and so that the separate function is general enough to be re-used by the other functions. Also, no function other than main() may have more than 5 lines of code. (This is counting declarations, but not counting the function header, blank lines, or lines that have only a curly brace on them.) In my solution I was able to create just 3
helper functions, 2 of which are used repeatedly by the various functions.
These additional criteria are intended as an extra challenge and may be difficult for many of you. If you can't figure it out, give it your best shot, but don't be too discouraged. It's just 5 points. And be sure to study the posted solution carefully.
F
F
T
F
F
F
F
Suggestions
1) I strongly suggest that you never compare cards with each other. This is often the instinctive direction that students go at first. For example, to find whether the hand contains a pair, you may be tempted to compare every card in the hand with every other card in the hand. I suggest that instead, you count how many of each card there are.
2) If you find that you are really struggling with even the containsPair() function, I suggest that you solve an even simpler problem first: write a function that returns true if the hand contains a pair of 2's (false otherwise). Just count how many 2's there are in the hand, and return true if there are exactly two of them (false otherwise).
3) Test these functions independently. Once you are sure that they all work, the program logic for the complete program will be fairly straightforward.
Here is code that tests a containsPair function:
int hand[] = {2, 5, 3, 2, 9};
if (containsPair (hand)) {
}
F
T
F
F
F
F
F
F
F
F
IF
F
IT
T
cout<<"contains a pair" << endl;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
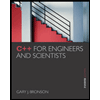
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
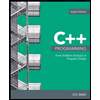
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
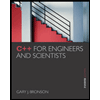
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
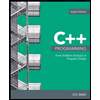
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning