using System; using System.IO; class Program { static void Main() { // Prompt for patient information until the user enters 999 to quit while (true) { Console.Write("Enter patient ID number or 999 to quit >> "); string patientId = Console.ReadLine(); if (patientId == "999") break; Console.Write("Enter last name >> "); string lastName = Console.ReadLine(); Console.Write("Enter balance >> "); decimal balance; while (!decimal.TryParse(Console.ReadLine(), out balance)) { Console.WriteLine("Invalid input. Please enter a valid balance."); } // Create a Patient object and write the data to the file Patient patient = new Patient(patientId, lastName, balance); WritePatientRecord(patient); } Console.WriteLine("Patient records saved to Patients.txt"); } static void WritePatientRecord(Patient patient) { // Open the file in append mode or create it if it doesn't exist using (StreamWriter writer = File.AppendText("Patients.txt")) { // Write the patient data in the specified format writer.WriteLine($"{patient.Id}, {patient.LastName}, {patient.Balance}"); } } } class Patient { public string Id { get; } public string LastName { get; } public decimal Balance { get; } public Patient(string id, string lastName, decimal balance) { Id = id; LastName = lastName; Balance = balance; } } Can someone explain how can this C# code write the output of this program to a file called “Patients.txt”. Do i have to create a text file called “Patients” first? OR what should I do. The information below might help explain what im trying to say
using System; using System.IO; class
Can someone explain how can this C# code write the output of this program to a file called “Patients.txt”. Do i have to create a text file called “Patients” first? OR what should I do.
The information below might help explain what im trying to say


Step by step
Solved in 5 steps with 3 images

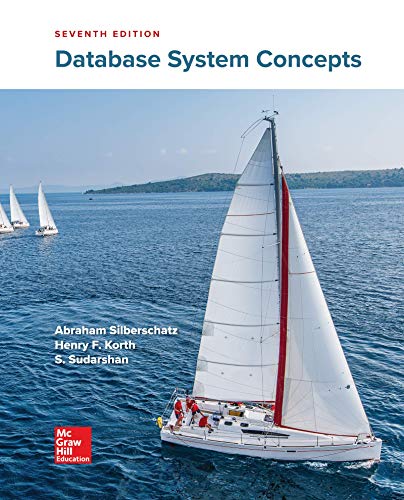
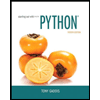
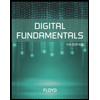
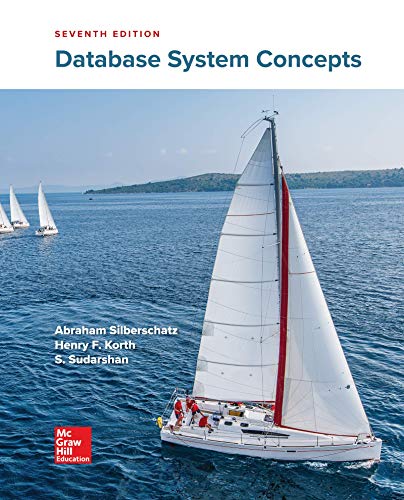
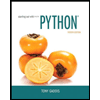
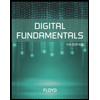
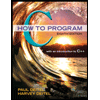
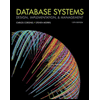
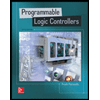