User.java User is a class that represents a user in the music player application. Every User contains a library which stores all their Playlists. A User can create Playlists or add Playlists made by other users to their library. Additionally, they can also share Playlists with other Users. Each User has the following attributes: username - Represents the username of the user The name of a user should never be null library Represents the library of the User. A list that contains all the playlists the user has created or added. Every user's library will start with a playlist called "Liked" which stores the songs that the user has liked. This playlist should have a creation Date of 0. Save for the Liked playlist, a user's library should be sorted in order of recency. That is, a more recently created playlist should appear earlier than an older one. currentPlaying Represents the song the user is currently listening to. -If it is null, then the user is not listening to any song. User should have a constructor that takes in the username. If an invalid value is passed for name, simply default to an empty String. Don't forget to create "Liked" playlist in the user's library! This playlist should have a creation Date of 0 A User should also support the following behaviors: • createPlaylist () - Takes in a String and int, where the String is the title of the Playlist the user wants to create, and the int representing its age. If a playlist with this title already exists in the user's library, exit the method. - HINT: getPlaylist, detailed below, might come in handy Creates a new Playlist with the given title and adds it to the appropriate position in the user's library. Set the initial values of the likes and dislikes of the playlist to 0. Remember that the "Liked" playlist stays in the first index of the library. Every Playlist after that should be appended to the end. addPlaylist () Takes in a Playlist and adds it to the appropriate position in the user's library. In the tie case for playlist age, add the newly created list in front of the existing one(s). If a playlist having this title already exists in the user's library, "overwrite" the existing playlist by removing it and adding the new one. Returns true if an overwrite was performed, false otherwise. getPlaylist () - Takes in a String parameter and returns the Playlist in the user's library which has the same title. Returns null if no such playlist exists. remove Playlist () - - Removes a specific Playlist from the user's library. Note: The "Liked" playlist and any other playlist that the user has created themself should not be removed from their library. Takes in title of the playlist to be removed, returns a boolean representing whether the playlist was removed. ⚫ likeSong() - - Takes in a Song and likes it. If the User has previously liked the same Song, the Song should not be liked again. Remember to add the Song that the User liked to the user's "Liked" playlist. dislikeSong () Takes in a Song and dislikes it. Contrary to liking a Song, a User can dislike a Song as many times as they want. ⚫ like Playlist () 。 Takes in a Playlist and likes it. • A User can like a Playlist an unlimited number of times. dislike Playlist () 。 Takes in a Playlist and dislikes it. • A User can dislike a Playlist an unlimited number of times. share Playlist () - Takes in a User and a number representing the index of the Playlist the user wants to share from their library. When a Playlist is shared with a User, it simply gets added to their library. playSong () - Essentially a setter method for currentPlaying - Takes a Song and updates the internal state of the User to reflect that they are currently listening to this song. pauseSong () Pauses the Song the user is listening to by setting currentPlaying to null. ⚫ toString() - If the User is currently listening to a song, returns: o (username} is listening to (song_title}, Duration: {song_duration), Likes: (song_likes), Dislikes: {song dislikes} If the User isn't listening to a song, returns: o (username} is idle Getter and setter methods for instance variables as required. For setters, note that if an invalid value is passed in, you should not modify the value of the instance variable.
User.java User is a class that represents a user in the music player application. Every User contains a library which stores all their Playlists. A User can create Playlists or add Playlists made by other users to their library. Additionally, they can also share Playlists with other Users. Each User has the following attributes: username - Represents the username of the user The name of a user should never be null library Represents the library of the User. A list that contains all the playlists the user has created or added. Every user's library will start with a playlist called "Liked" which stores the songs that the user has liked. This playlist should have a creation Date of 0. Save for the Liked playlist, a user's library should be sorted in order of recency. That is, a more recently created playlist should appear earlier than an older one. currentPlaying Represents the song the user is currently listening to. -If it is null, then the user is not listening to any song. User should have a constructor that takes in the username. If an invalid value is passed for name, simply default to an empty String. Don't forget to create "Liked" playlist in the user's library! This playlist should have a creation Date of 0 A User should also support the following behaviors: • createPlaylist () - Takes in a String and int, where the String is the title of the Playlist the user wants to create, and the int representing its age. If a playlist with this title already exists in the user's library, exit the method. - HINT: getPlaylist, detailed below, might come in handy Creates a new Playlist with the given title and adds it to the appropriate position in the user's library. Set the initial values of the likes and dislikes of the playlist to 0. Remember that the "Liked" playlist stays in the first index of the library. Every Playlist after that should be appended to the end. addPlaylist () Takes in a Playlist and adds it to the appropriate position in the user's library. In the tie case for playlist age, add the newly created list in front of the existing one(s). If a playlist having this title already exists in the user's library, "overwrite" the existing playlist by removing it and adding the new one. Returns true if an overwrite was performed, false otherwise. getPlaylist () - Takes in a String parameter and returns the Playlist in the user's library which has the same title. Returns null if no such playlist exists. remove Playlist () - - Removes a specific Playlist from the user's library. Note: The "Liked" playlist and any other playlist that the user has created themself should not be removed from their library. Takes in title of the playlist to be removed, returns a boolean representing whether the playlist was removed. ⚫ likeSong() - - Takes in a Song and likes it. If the User has previously liked the same Song, the Song should not be liked again. Remember to add the Song that the User liked to the user's "Liked" playlist. dislikeSong () Takes in a Song and dislikes it. Contrary to liking a Song, a User can dislike a Song as many times as they want. ⚫ like Playlist () 。 Takes in a Playlist and likes it. • A User can like a Playlist an unlimited number of times. dislike Playlist () 。 Takes in a Playlist and dislikes it. • A User can dislike a Playlist an unlimited number of times. share Playlist () - Takes in a User and a number representing the index of the Playlist the user wants to share from their library. When a Playlist is shared with a User, it simply gets added to their library. playSong () - Essentially a setter method for currentPlaying - Takes a Song and updates the internal state of the User to reflect that they are currently listening to this song. pauseSong () Pauses the Song the user is listening to by setting currentPlaying to null. ⚫ toString() - If the User is currently listening to a song, returns: o (username} is listening to (song_title}, Duration: {song_duration), Likes: (song_likes), Dislikes: {song dislikes} If the User isn't listening to a song, returns: o (username} is idle Getter and setter methods for instance variables as required. For setters, note that if an invalid value is passed in, you should not modify the value of the instance variable.
Chapter9: Using Classes And Objects
Section: Chapter Questions
Problem 2CP
Related questions
Question

Transcribed Image Text:User.java
User is a class that represents a user in the music player application. Every User contains a library
which stores all their Playlists. A User can create Playlists or add Playlists made by other
users to their library. Additionally, they can also share Playlists with other Users.
Each User has the following attributes:
username
-
Represents the username of the user
The name of a user should never be null
library
Represents the library of the User.
A list that contains all the playlists the user has created or added.
Every user's library will start with a playlist called "Liked" which stores the songs that the user
has liked. This playlist should have a creation Date of 0.
Save for the Liked playlist, a user's library should be sorted in order of recency. That is, a more
recently created playlist should appear earlier than an older one.
currentPlaying
Represents the song the user is currently listening to.
-If it is null, then the user is not listening to any song.
User should have a constructor that takes in the username.
If an invalid value is passed for name, simply default to an empty String.
Don't forget to create "Liked" playlist in the user's library!
This playlist should have a creation Date of 0
A User should also support the following behaviors:
• createPlaylist ()
- Takes in a String and int, where the String is the title of the Playlist the user wants to create,
and the int representing its age. If a playlist with this title already exists in the user's library, exit
the method.
-
HINT: getPlaylist, detailed below, might come in handy
Creates a new Playlist with the given title and adds it to the appropriate position in the
user's library. Set the initial values of the likes and dislikes of the playlist to 0.
Remember that the "Liked" playlist stays in the first index of the library. Every Playlist after
that should be appended to the end.
addPlaylist ()
Takes in a Playlist and adds it to the appropriate position in the user's library.
In the tie case for playlist age, add the newly created list in front of the existing one(s).
If a playlist having this title already exists in the user's library, "overwrite" the existing playlist by
removing it and adding the new one.
Returns true if an overwrite was performed, false otherwise.
getPlaylist ()
-
Takes in a String parameter and returns the Playlist in the user's library which has the same
title.
Returns null if no such playlist exists.

Transcribed Image Text:remove Playlist ()
-
-
Removes a specific Playlist from the user's library.
Note: The "Liked" playlist and any other playlist that the user has created themself should not
be removed from their library.
Takes in title of the playlist to be removed, returns a boolean representing whether the playlist
was removed.
⚫ likeSong()
-
-
Takes in a Song and likes it.
If the User has previously liked the same Song, the Song should not be liked again.
Remember to add the Song that the User liked to the user's "Liked" playlist.
dislikeSong ()
Takes in a Song and dislikes it.
Contrary to liking a Song, a User can dislike a Song as many times as they want.
⚫ like Playlist ()
。 Takes in a Playlist and likes it.
• A User can like a Playlist an unlimited number of times.
dislike Playlist ()
。 Takes in a Playlist and dislikes it.
• A User can dislike a Playlist an unlimited number of times.
share Playlist ()
- Takes in a User and a number representing the index of the Playlist the user wants to
share from their library.
When a Playlist is shared with a User, it simply gets added to their library.
playSong ()
-
Essentially a setter method for currentPlaying
- Takes a Song and updates the internal state of the User to reflect that they are currently
listening to this song.
pauseSong ()
Pauses the Song the user is listening to by setting currentPlaying to null.
⚫ toString()
-
If the User is currently listening to a song, returns:
o
(username} is listening to (song_title}, Duration:
{song_duration), Likes: (song_likes), Dislikes:
{song dislikes}
If the User isn't listening to a song, returns:
o (username} is idle
Getter and setter methods for instance variables as required. For setters, note that if an invalid
value is passed in, you should not modify the value of the instance variable.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 1 steps

Recommended textbooks for you
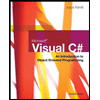
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
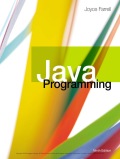
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
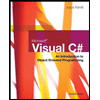
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
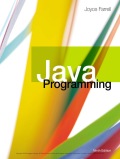
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT