Use java to solve this company wants to make a simple application to keep track of trips and passengers. you got the following information: 3 classes needed one super class Trip and sub classes Passenger and Driver. • It is required to store the whole data in one collection The Trip class has: 1- a number 2-a driver 3-a specific date. 4- In addition, passengers could be added or removed within a given limit for the maximum number of passengers who could be in the same trip. 5- there are other attributes (add at least 3 attributes from your choice). 6- trip number is unique within the same date. The Driver class has: 1- a unique ID 2- a name 3- other attributes (add at least 2 attributes from your choice). the Passenger class has: 1- a unique Civil ID (National ID) 2- a name 3- other attributes (add at least 2 attributes from your choice but one of them should be common with drivers). Moreover, you have been informed that the following operations happen frequently: • Offering a new trip • Adding a passenger to a specific trip • Removing a passenger from a specific trip • Retrieving the average number of passengers per trip of a specified date • Displaying all available trips in a format similar to the following: date1: tripNo1 tripNo2 tripNo3 … date2: tripNo1 tripNo2 tripNo3 … … where dates and trips are sorted in ascending order • Saving all the data into a text file Q1: There are common attributes and methods between passengers and drivers. What is the best choice for designing and writing the codes of these two classes? Explain your answer. Q2: Draw a simple class diagram showing only relationships between the classes. For each class, it is required to implement constructors, setters, getters, toString() method, and any other necessary method • If the user tries to do an operation that could violate the state of objects, the operation should be ignored and the application should display an error message (e.g. adding a passenger to the same trip twice, etc.) • Checking equality of any 2 objects should be done via the equals() method • There is a class that will do the main job of as follows: o It has one collection to store the whole data (all trips) o It has static methods, one for each operation happens frequently o For each adding or removing operation, a message should be displayed to the user to explain the status of the operation (i.e. if it was successful or not) After implementing the required classes, design and implement a testing class to test them as follows: • Create at least 7 trips in at least 3 different dates and add them to the collection that stores the whole data then add and remove some passengers from them. • Try to violate the state of the objects and show that your code prevents all violations. • Show that the other operations that happen frequently are working fine • At the end, the whole data should be saved into a text file and this file should be saved automatically inside the folder contains your Java project.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Use java to solve this
company wants to make a simple application to keep track of trips and
passengers.
you got the following information:
3 classes needed one super class Trip and sub classes Passenger and Driver.
• It is required to store the whole data in one collection
The Trip class has:
1- a number
2-a driver
3-a specific date.
4- In addition, passengers could be added or removed within a given limit for the maximum number of passengers who could be in the
same trip.
5- there are other attributes (add at least 3 attributes from your choice).
6- trip number is unique within the same date.
The Driver class has:
1- a unique ID
2- a name
3- other attributes (add at least 2 attributes from your choice).
the Passenger class has:
1- a unique Civil ID (National ID)
2- a name
3- other attributes (add at least 2 attributes from your choice but one of them should be common with drivers).
Moreover, you have been informed that the following operations happen frequently:
• Offering a new trip
• Adding a passenger to a specific trip
• Removing a passenger from a specific trip
• Retrieving the average number of passengers per trip of a specified date
• Displaying all available trips in a format similar to the following:
date1: tripNo1 tripNo2 tripNo3 …
date2: tripNo1 tripNo2 tripNo3 …
…
where dates and trips are sorted in ascending order
• Saving all the data into a text file
Q1: There are common attributes and methods between passengers and drivers. What is the
best choice for designing and writing the codes of these two classes? Explain your answer.
Q2: Draw a simple class diagram showing only relationships between the classes.
For each class, it is required to implement constructors, setters, getters, toString() method,
and any other necessary method
• If the user tries to do an operation that could violate the state of objects, the operation
should be ignored and the application should display an error message (e.g. adding a
passenger to the same trip twice, etc.)
• Checking equality of any 2 objects should be done via the equals() method
• There is a class that will do the main job of as follows:
o It has one collection to store the whole data (all trips)
o It has static methods, one for each operation happens frequently
o For each adding or removing operation, a message should be displayed to the user to
explain the status of the operation (i.e. if it was successful or not)
After implementing the required classes, design and implement a testing class to test them as
follows:
• Create at least 7 trips in at least 3 different dates and add them to the collection that stores
the whole data then add and remove some passengers from them.
• Try to violate the state of the objects and show that your code prevents all violations.
• Show that the other operations that happen frequently are working fine
• At the end, the whole data should be saved into a text file and this file should be saved
automatically inside the folder contains your Java project.

Step by step
Solved in 3 steps with 6 images

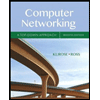
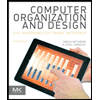
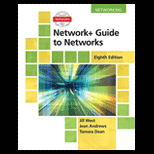
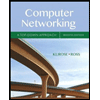
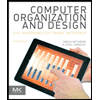
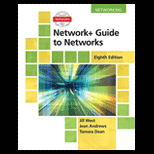
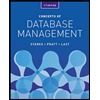
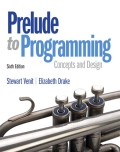
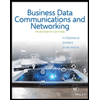