use java (ArrayList, inheritance, polymorphism, File I/O and basic Java) Student class: First, you need to design, code in Java, test and document a base class, Student. The Student class will have the following information, and all of these should be defined as Private: A first name (given name) A last name (family name/surname) Student number (ID) – an integer number (of type long) The Student class will have at least the following constructors and methods: (i) two constructors - one without any parameters (the default constructor), and one with parameters to give initial values to all the instance variables of Student. (ii) only necessary set and get methods for a valid class design. (iii) a reportGrade method, which you have nothing to report here, you can just print to the screen a message “There is no grade here.”. This method will be overridden in the respective child classes. (iv) an equals method which compares two student objects and returns true if they have the same student number (ID), otherwise it returns false. You may add other methods in the Student class as you see appropriate, however you will need to justify why those methods are required. Design, code in Java, test and document (at least) the following classes – a Student_Course class, a Student_Research class (both derived from the Student class) a Unit_Course class, Research class (both derived from the Unit class specified below) and a Client class. Assuming in this program, you allow multiple student objects to be created (i.e. arraylist of student objects). For course work students (Student_Course class): (a) Contain enrolment type. (b) Provide a reportGrade method such that will output “C” (to identify as course work student), the Name (first name and last name), student number, the unit ID, the overall mark, and the final grade of the student. For research students (Student_Research class): (a) Contain enrolment type. (b) Provide a reportGrade method such that will output “R” (to identify as research student), the Name (first name and last name), student number, the overall mark, and the final grade of the student. Unit class: (a) Enrolment type: “C” for course work enrolment and “R” for research enrolment. (b) A final grade reporting method for reporting the “NA” for not available. Course work unit (Unit_Course class): (a) Will have the information of the unit ID (of type string; e.g. ICT333), and the level of the unit (of type integer; e.g. 3 for third year) (b) There are two assignments, each marked out of a maximum of 100 marks and equally weighted. The marks for each assignment are recorded separately. (c) There is one final examination that is marked out of a maximum of 100 marks and recorded separately. (d) An overall mark (to be calculated within the class) (e) A final grade, which is a string (avoid code duplication for calculating this) final grade is on the basis of an overall mark, which is a number in the range 0 to 100 and is obtained by calculating the weighted average of the student's performance in the assessment components. The criteria for calculating the weighted average is as defined below: The two assignments together count for a total of 60% (30% each) of the final grade, and the final exam is worth 40% of the final grade. A grade is to be awarded for the students as follows: 80 or higher is an HD, 70 or less than 80 is a D, 60 or less than 70) is a C, 50 or less than 60) is a P, and below 50 is an N. Research enrolment (Research class): (a) Enrolment type as “R” (b) There is a proposal, marked out of a maximum of 100 mark. (c) There is a final dissertation, marked out of a maximum of 100 mark. (d) An overall mark (to be calculated within the class) (e) A final grade, which is a string (avoid code duplication for calculating this) The final grade based an overall mark, between (0 to 100) & is obtained by calculating weighted average of the assessment components. The criteria is as defined below: proposal is worth 40% of the final grade, & final dissertation is worth 60% of the final grade. A grade is to be awarded for the students as follows: 80 or higher is an HD, 70 or less than 80 is a D, 60 or less than 70) is a C, 50 or less than 60) is a P, and below 50 is an N. Client Class will allow entry of these data for several different student into an ArrayList and then perform some analysis and queries. provide the user a menu to perform the following**. need to load the information of the students from a CSV file (student.csv) before displaying the menu. only need 1 ArrayList & 1 menu for this. in the csv file, first item can be C or R to differentiate the entry is a Student_Course object, or a Student_Research object. You can then decide how you want other data to be listed in the csv file. ***The menu is in the image attached! Note that the program will loop around until the user selects the first option (Quit). You should use 5 coursework students and 5 research students in your test. Consider all possible enrolment types in your test.
use java (ArrayList, inheritance, polymorphism, File I/O and basic Java)
Student class:
First, you need to design, code in Java, test and document a base class, Student. The Student class will
have the following information, and all of these should be defined as Private:
-
A first name (given name)
-
A last name (family name/surname)
-
Student number (ID) – an integer number (of type long)
The Student class will have at least the following constructors and methods:
-
(i) two constructors - one without any parameters (the default constructor), and one with
parameters to give initial values to all the instance variables of Student.
-
(ii) only necessary set and get methods for a valid class design.
-
(iii) a reportGrade method, which you have nothing to report here, you can just print to the screen
a message “There is no grade here.”. This method will be overridden in the respective child
classes.
-
(iv) an equals method which compares two student objects and returns true if they have the same
student number (ID), otherwise it returns false.
You may add other methods in the Student class as you see appropriate, however you will need to justify why those methods are required.
Design, code in Java, test and document (at least) the following classes –
-
a Student_Course class, a Student_Research class (both derived from the Student class)
-
a Unit_Course class, Research class (both derived from the Unit class specified below)
-
and a Client class.
Assuming in this program, you allow multiple student objects to be created (i.e. arraylist of student objects).
For course work students (Student_Course class):
-
(a) Contain enrolment type.
-
(b) Provide a reportGrade method such that will output “C” (to identify as course work student), the Name (first name and last name), student number, the unit ID, the overall mark, and the final grade of the student.
For research students (Student_Research class):
-
(a) Contain enrolment type.
-
(b) Provide a reportGrade method such that will output “R” (to identify as research student), the Name (first name and last name), student number, the overall mark, and the final grade of the student.
Unit class:
(a) Enrolment type: “C” for course work enrolment and “R” for research enrolment.
(b) A final grade reporting method for reporting the “NA” for not available.
Course work unit (Unit_Course class):
-
(a) Will have the information of the unit ID (of type string; e.g. ICT333), and the level of the unit (of type integer; e.g. 3 for third year)
-
(b) There are two assignments, each marked out of a maximum of 100 marks and equally weighted. The marks for each assignment are recorded separately.
-
(c) There is one final examination that is marked out of a maximum of 100 marks and recorded separately.
-
(d) An overall mark (to be calculated within the class)
-
(e) A final grade, which is a string (avoid code duplication for calculating this)
final grade is on the basis of an overall mark, which is a number in the range 0 to 100 and is obtained by calculating the weighted average of the student's performance in the assessment components. The criteria for calculating the weighted average is as defined below:
The two assignments together count for a total of 60% (30% each) of the final grade, and the final exam is worth 40% of the final grade.
A grade is to be awarded for the students as follows: 80 or higher is an HD, 70 or less than 80 is a D, 60 or less than 70) is a C, 50 or less than 60) is a P, and below 50 is an N.
Research enrolment (Research class):
(a) Enrolment type as “R”
(b) There is a proposal, marked out of a maximum of 100 mark.
(c) There is a final dissertation, marked out of a maximum of 100 mark. (d) An overall mark (to be calculated within the class)
(e) A final grade, which is a string (avoid code duplication for calculating this)
The final grade based an overall mark, between (0 to 100) & is obtained by calculating weighted average of the assessment components. The criteria is as defined below:
proposal is worth 40% of the final grade, & final dissertation is worth 60% of the final grade.
A grade is to be awarded for the students as follows: 80 or higher is an HD, 70 or less than 80 is a D, 60 or less than 70) is a C, 50 or less than 60) is a P, and below 50 is an N.
Client Class
will allow entry of these data for several different student into an ArrayList and then perform some analysis and queries.
provide the user a menu to perform the following**. need to load the information of the students from a CSV file (student.csv) before displaying the menu. only need 1 ArrayList & 1 menu for this. in the csv file, first item can be C or R to differentiate the entry is a Student_Course object, or a Student_Research object. You can then decide how you want other data to be listed in the csv file.
***The menu is in the image attached!
Note that the program will loop around until the user selects the first option (Quit).
You should use 5 coursework students and 5 research students in your test. Consider all possible enrolment types in your test.


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 7 images

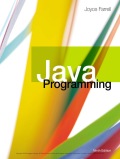
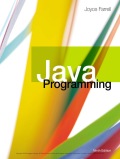