Thus is supposed to be the output of the code: When Padfoot the dog speaks he says woof, woof! When I tell him to come, he runs over! His tricks are begs, sits, rolls over. ******** When Cruickshanks the cat speaks he says meow, meow! When I tell him to come, he walks away! His tricks are purrs, licks my hand. ******** When Hedwig the bird speaks he says tweet, tweet. When I tell him to come, he flies over! His tricks are screeches, lands on my finger. ******** When Nagini the snake speaks he says hiss, hiss. When I tell him to come, he slithers around! His tricks are eats his prey whole. ******** But my code does not compile ******** #include #include #include using namespace std; // ToDo: Create child classes for 4 kinds of Pets: Dog, Cat, Bird and Snake. // HINT: You can change the Pet class to add or change data members. Since all of // of the behaviors return a string, the Pet parent class can contain strings for // each behavior and set functions that can be called from the child. // Pet class uses a type to distinguish between different kinds of pets. You still // need getType(), but it can be stored as a string in the parent or returned from // the child. class Pet { public: // Constructor, no defealt Pet(string nm, string kind); // return the name string getName() const; // return the type string getType() const; // return the string when pet speaks string speak() const; // return the string when pet comes string come() const; // return the string when pet does tricks string do_tricks() const; void setSpeak(string speak); void setCome(string come); void setDo_tricks(string tricks); private: string name; string tricks; string come_str; string speak_str; string type; static const char DOG = 'D'; static const char CAT = 'C'; static const char BIRD = 'B'; static const char SNAKE= 'S'; }; class DOG:public Pet { public: DOG(string name); }; class CAT:public Pet { public: CAT(string name); }; class BIRD:public Pet { public: BIRD(string name); }; class SNAKE:public Pet { public: SNAKE(string name); }; int main() { // ToDo: the type will no longer be needed once you modify the code // to have classes for all 4 kinds of Pets. Pet dog("Padfoot", "dog"); Pet cat("Cruickshanks", "cat"); Pet bird("Hedwig", "bird"); Pet snake("Nagini", "snake"); // ToDo: the code below should not have to change to use the new classes. cout << "When " << dog.getName() << " the " << dog.getType() << " speaks he says " << dog.speak() << endl; cout << "When I tell him to come, he " << dog.come() << endl; cout << "His tricks are " << dog.do_tricks() << endl; cout << "********\n" << endl; cout << "When " << cat.getName() << " the " << cat.getType() << " speaks he says " << cat.speak() << endl; cout << "When I tell him to come, he " << cat.come() << endl; cout << "His tricks are " << cat.do_tricks() << endl; cout << "********\n" << endl; cout << "When " << bird.getName() << " the " << bird.getType() << " speaks he says " << bird.speak() << endl; cout << "When I tell him to come, he " << bird.come() << endl; cout << "His tricks are " << bird.do_tricks() << endl; cout << "********\n" << endl; cout << "When " << snake.getName() << " the " << snake.getType() << " speaks he says " << snake.speak() << endl; cout << "When I tell him to come, he " << snake.come() << endl; cout << "His tricks are " << snake.do_tricks() << endl; cout << "********\n" << endl; return 0; } // Constructor for a pet Pet::Pet(string nm, string kind) : name(nm), type(toupper(kind[0])) { if (type != DOG && type != CAT && type != BIRD && type != SNAKE) { cout << "Illegal pet, you can't keep a " << kind << " as a Pet!"; exit(1); } } void Pet::setSpeak(string speak) { speak_str=speak; } void Pet::setCome(string come) { come_str=come; } void Pet::setDo_tricks(string do_tricks) { do_tricks=tricks; } // return the pet's name string Pet::getName() const { return name; } // return the pet's type string Pet::getType() const { return type; } // return the string when pet does speaks string Pet::speak() const { return speak_str; } // return the string when pet does comes string Pet::come() const { return come_str; } // return the string when pet does tricks string Pet::do_tricks() const { return tricks; } DOG::dog(string name):Pet(name,"dog") { setSpeak("Woof, Woof"); setCome("run over!"); setDo_tricks("rolls over."); } CAT::cat(string name):Pet(name,"cat") { setSpeak("Meow, Meow!"); setCome("he walks away!"); setDo_Trick("licks my hand."); } BIRD::bird(string name): Pet(name,"bird") { setSpeak("Tweet, Tweet!"); setCome("flies over!"); setDo_tricks("lands on my finger."); } SNAKE::snake(string name):Pet(name,"snake") { setSpeak("hiss,hiss"); setCome("slither around!"); setDo_tricks("eats his prey whole.") }
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
When Padfoot the dog speaks he says woof, woof!
When I tell him to come, he runs over!
His tricks are begs, sits, rolls over.
********
When Cruickshanks the cat speaks he says meow, meow!
When I tell him to come, he walks away!
His tricks are purrs, licks my hand.
********
When Hedwig the bird speaks he says tweet, tweet.
When I tell him to come, he flies over!
His tricks are screeches, lands on my finger.
********
When Nagini the snake speaks he says hiss, hiss.
When I tell him to come, he slithers around!
His tricks are eats his prey whole.
********

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

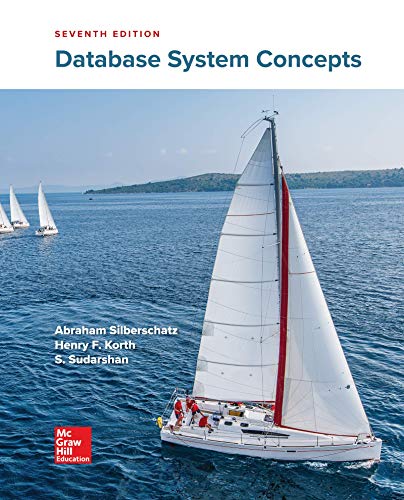
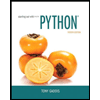
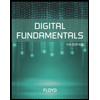
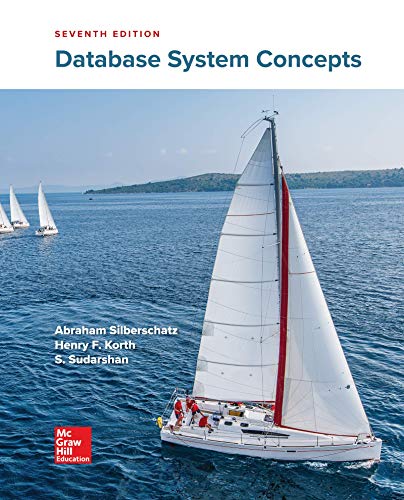
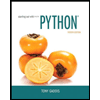
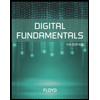
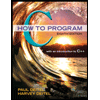
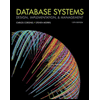
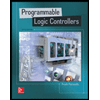