Step 1: Inspect the project The project contains HTML, CSS, and JavaScript files: ⚫ index.html contains the "New task:" label, a textbox for entering a new task, an Add button, and an empty ordered list. • styles.css adds styling to the webpage. ⚫ todo.js contains four functions that need implementing. Step 2: Add new task (4 points) To add a new task, make the following modifications: 1. In domLoaded (), register addBtnClick() as the Add button's click event handler. 2. In addBtnClick(), extract the text entered in the textbox, then call addTask() with the new task. 3. In addTask(): • Call document.createElement() to create a new element. • Set the new element's innerHTML to the following string where NEW TASK is the addTask() parameter: NEW TASK ✖ • Call document.querySelector() to find the DOM node. • Call appendChild() on the DOM node with the new element to append the to the ordered list. When the above modifications are implemented, the user should be able to type a new task, click Add, and see the new task in the list below. Each additional task added should appear at the end of the list. Clicking the * next to each task should not do anything. Step 3: Improve task entering (2 points) The user can enter tasks faster by making three improvements: 1) Clearing a newly added task from the textbox automatically, 2) Putting the focus back on the textbox after clicking Add, and 3) Allowing the user to hit Enter instead of clicking the Add button. Make the following modifications: 1. In domLoaded(), add a keyup event handler for the textbox that has an event parameter. 1. In domLoaded(), add a keyup event handler for the textbox that has an event parameter. 2. In the keyup event handler, call addBtnClick() if the event.key is "Enter". 3. In addBtnClick(): • Clear the textbox's value property by assigning an empty string after the call to addTask(). • Call the textbox method focus() to put the focus back on the textbox. When the above modifications are implemented, the user should be able to quickly type tasks and press Enter without ever having to click the Add button or manually delete the previously typed task. If the user does click Add, the focus is automatically put back on the textbox so the user doesn't have to click the textbox in order to type the next task. Step 4: Prevent empty tasks (1 point) The user can hit Enter or click Add without typing a task, but an empty task should not be added to the list. Add an if statement in addBtnClick() to prevent calling addTask() with an empty string argument. Step 5: Remove tasks (3 points) When the user clicks the done button (*) next to each task, the task should be removed from the list. Make the following modifications: 1. In addTask(): • After the existing code that appends the new list item, search the DOM for all buttons that use the done-btn class. • Register removeTask() as the last done button's click event handler. 2. In removeTask(): • Use the parentNode property to assign a variable with event.target's parent node (event.target is the done button that was clicked, and the parent is the element that contains the done button). ⚫ Call removeChild() on the element (the element's parent node) to remove the element. When the above modifications are implemented, clicking on any task's button will cause the task to immediately disappear.
Step 1: Inspect the project The project contains HTML, CSS, and JavaScript files: ⚫ index.html contains the "New task:" label, a textbox for entering a new task, an Add button, and an empty ordered list. • styles.css adds styling to the webpage. ⚫ todo.js contains four functions that need implementing. Step 2: Add new task (4 points) To add a new task, make the following modifications: 1. In domLoaded (), register addBtnClick() as the Add button's click event handler. 2. In addBtnClick(), extract the text entered in the textbox, then call addTask() with the new task. 3. In addTask(): • Call document.createElement() to create a new element. • Set the new element's innerHTML to the following string where NEW TASK is the addTask() parameter: NEW TASK ✖ • Call document.querySelector() to find the DOM node. • Call appendChild() on the DOM node with the new element to append the to the ordered list. When the above modifications are implemented, the user should be able to type a new task, click Add, and see the new task in the list below. Each additional task added should appear at the end of the list. Clicking the * next to each task should not do anything. Step 3: Improve task entering (2 points) The user can enter tasks faster by making three improvements: 1) Clearing a newly added task from the textbox automatically, 2) Putting the focus back on the textbox after clicking Add, and 3) Allowing the user to hit Enter instead of clicking the Add button. Make the following modifications: 1. In domLoaded(), add a keyup event handler for the textbox that has an event parameter. 1. In domLoaded(), add a keyup event handler for the textbox that has an event parameter. 2. In the keyup event handler, call addBtnClick() if the event.key is "Enter". 3. In addBtnClick(): • Clear the textbox's value property by assigning an empty string after the call to addTask(). • Call the textbox method focus() to put the focus back on the textbox. When the above modifications are implemented, the user should be able to quickly type tasks and press Enter without ever having to click the Add button or manually delete the previously typed task. If the user does click Add, the focus is automatically put back on the textbox so the user doesn't have to click the textbox in order to type the next task. Step 4: Prevent empty tasks (1 point) The user can hit Enter or click Add without typing a task, but an empty task should not be added to the list. Add an if statement in addBtnClick() to prevent calling addTask() with an empty string argument. Step 5: Remove tasks (3 points) When the user clicks the done button (*) next to each task, the task should be removed from the list. Make the following modifications: 1. In addTask(): • After the existing code that appends the new list item, search the DOM for all buttons that use the done-btn class. • Register removeTask() as the last done button's click event handler. 2. In removeTask(): • Use the parentNode property to assign a variable with event.target's parent node (event.target is the done button that was clicked, and the parent is the element that contains the done button). ⚫ Call removeChild() on the element (the element's parent node) to remove the element. When the above modifications are implemented, clicking on any task's button will cause the task to immediately disappear.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Question
here is html code
<!DOCTYPE html>
<html lang="en">
<head>
<title>To-Do List</title>
<linkrel="stylesheet"href="styles.css">
<scriptsrc="todo.js"defer></script>
</head>
<body>
<h1>To-Do List</h1>
<labelfor="new-task">New task:</label>
<inputid="new-task"type="text"autofocus>
<buttonid="add-btn">Add</button>
<ol>
<!-- Items are added dynamically with JavaScript -->
</ol>
</body>
</html>
this is the javascript code that has to be modified
window.addEventListener("DOMContentLoaded", domLoaded);
function domLoaded() {
// TODO: Complete the function
}
function addBtnClick() {
// TODO: Complete the function
}
function addTask(task) {
// TODO: Complete the function
}
function removeTask(event) {
// TODO: Complete the function
}

Transcribed Image Text:Step 1: Inspect the project
The project contains HTML, CSS, and JavaScript files:
⚫ index.html contains the "New task:" label, a textbox for entering a new task, an Add button, and an empty ordered list.
• styles.css adds styling to the webpage.
⚫ todo.js contains four functions that need implementing.
Step 2: Add new task (4 points)
To add a new task, make the following modifications:
1. In domLoaded (), register addBtnClick() as the Add button's click event handler.
2. In addBtnClick(), extract the text entered in the textbox, then call addTask() with the new task.
3. In addTask():
• Call document.createElement() to create a new <li> element.
• Set the new <li> element's innerHTML to the following string where NEW TASK is the addTask() parameter:
<span class="task-text">NEW TASK</span> <button class="done-btn">✖</button>
• Call document.querySelector() to find the <ol> DOM node.
• Call appendChild() on the <ol> DOM node with the new <li> element to append the <li> to the ordered list.
When the above modifications are implemented, the user should be able to type a new task, click Add, and see the new task in the list
below. Each additional task added should appear at the end of the list. Clicking the * next to each task should not do anything.
Step 3: Improve task entering (2 points)
The user can enter tasks faster by making three improvements: 1) Clearing a newly added task from the textbox automatically, 2)
Putting the focus back on the textbox after clicking Add, and 3) Allowing the user to hit Enter instead of clicking the Add button.
Make the following modifications:
1. In domLoaded(), add a keyup event handler for the textbox that has an event parameter.

Transcribed Image Text:1. In domLoaded(), add a keyup event handler for the textbox that has an event parameter.
2. In the keyup event handler, call addBtnClick() if the event.key is "Enter".
3. In addBtnClick():
• Clear the textbox's value property by assigning an empty string after the call to addTask().
• Call the textbox method focus() to put the focus back on the textbox.
When the above modifications are implemented, the user should be able to quickly type tasks and press Enter without ever having to
click the Add button or manually delete the previously typed task. If the user does click Add, the focus is automatically put back on the
textbox so the user doesn't have to click the textbox in order to type the next task.
Step 4: Prevent empty tasks (1 point)
The user can hit Enter or click Add without typing a task, but an empty task should not be added to the list. Add an if statement in
addBtnClick() to prevent calling addTask() with an empty string argument.
Step 5: Remove tasks (3 points)
When the user clicks the done button (*) next to each task, the task should be removed from the list.
Make the following modifications:
1. In addTask():
• After the existing code that appends the new list item, search the DOM for all buttons that use the done-btn class.
• Register removeTask() as the last done button's click event handler.
2. In removeTask():
• Use the parentNode property to assign a variable with event.target's parent node (event.target is the done button that was
clicked, and the parent is the <li> element that contains the done button).
⚫ Call removeChild() on the <ol> element (the <li> element's parent node) to remove the <li> element.
When the above modifications are implemented, clicking on any task's button will cause the task to immediately disappear.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
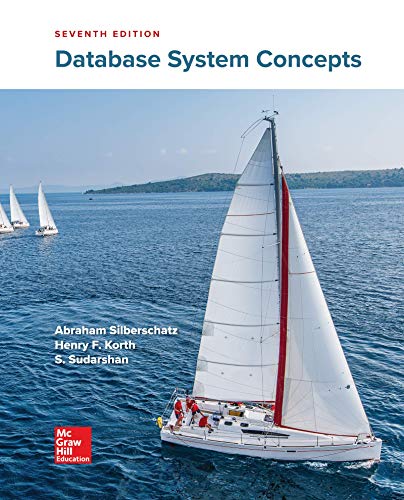
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
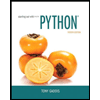
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
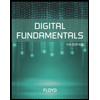
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
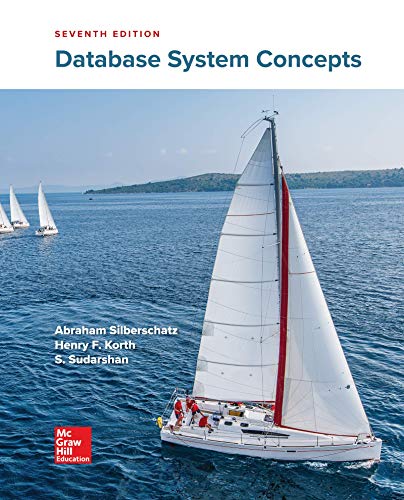
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
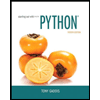
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
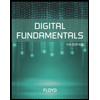
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
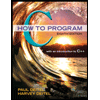
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
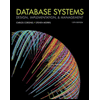
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
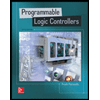
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education