So for my project program in Python I'm suppose to code a BankApp in which the user is suppose to input their username and password to access their balance details and withdraw or deposit money. The program works though there is a small issue. See when testing I notice a problem with the withdraw and deposit function, see if I put a negative value rather than saying "invalid" and looping back to the menu it will do the opposite which I'll provide a screenshot to explain it better. Can you fix and explain how you did it(also show a sceenshot on where you fix/added code)&(UserInfo will also be provided for code to work)-Thank you. Code def read_user_information(filename): usernames = [] passwords = [] balances = [] try: with open(filename, "r") as file: lines = file.readlines() for line in lines[2:]: data = line.strip().split() if len(data) == 3: usernames.append(data[0]) passwords.append(data[1]) balances.append(float(data[2])) except FileNotFoundError: print("User information file not found. A new file will be created.") return usernames, passwords, balances def write_user_information(filename, usernames, passwords, balances): with open(filename, "w") as file: file.write("userName passWord Balance\n") file.write("========================\n") for i in range(len(usernames)): file.write(f"{usernames[i]:<12} {passwords[i]:<12} {balances[i]:<}\n") def login(usernames, passwords): username_input = input("Enter username: ") password_input = input("Enter password: ") for i, username in enumerate(usernames): if username == username_input and passwords[i] == password_input: return i print("Invalid username or password. Please try again.") return None def deposit(balances, user_index): try: amount = float(input("Enter the amount to deposit: ")) balances[user_index] += amount print(f"Deposit successful. New balance: ${balances[user_index]:.2f}") except ValueError: print("Invalid amount entered. Deposit failed.") def withdraw(balances, user_index): try: amount = float(input("Enter the amount to withdraw: ")) if amount > balances[user_index]: print("Insufficient funds. Withdrawal failed.") else: balances[user_index] -= amount print(f"Withdrawal successful. New balance: ${balances[user_index]:.2f}") except ValueError: print("Invalid amount entered. Withdrawal failed.") def show_balance(balances, user_index): print(f"Current balance: ${balances[user_index]:.2f}") def add_new_user(usernames, passwords, balances): new_username = input("Enter new username: ") new_password = input("Enter new password: ") try: new_balance = float(input("Enter initial balance: ")) usernames.append(new_username) passwords.append(new_password) balances.append(new_balance) print(f"New user {new_username} added with balance: ${new_balance:.2f}") except ValueError: print("Invalid balance entered. User addition failed.") def main(): filename = "UserInformation.txt" usernames, passwords, balances = read_user_information(filename) user_index = None while user_index is None: user_index = login(usernames, passwords) while True: print("\nMenu:") print("D - Deposit Money") print("W - Withdraw Money") print("B - Display Balance") print("C - Change User") print("A - Add New Client") print("E - Exit") choice = input("Enter your choice: ").upper() if choice == 'D': deposit(balances, user_index) elif choice == 'W': withdraw(balances, user_index) elif choice == 'B': show_balance(balances, user_index) elif choice == 'C': user_index = None while user_index is None: user_index = login(usernames, passwords) elif choice == 'A': add_new_user(usernames, passwords, balances) elif choice == 'E': write_user_information(filename, usernames, passwords, balances) print("Exiting program. User information updated.") break else: print("Invalid option. Please try again.") if __name__ == "__main__": main()
So for my project program in Python I'm suppose to code a BankApp in which the user is suppose to input their username and password to access their balance details and withdraw or deposit money. The program works though there is a small issue. See when testing I notice a problem with the withdraw and deposit function, see if I put a negative value rather than saying "invalid" and looping back to the menu it will do the opposite which I'll provide a screenshot to explain it better. Can you fix and explain how you did it(also show a sceenshot on where you fix/added code)&(UserInfo will also be provided for code to work)-Thank you.
Code
def read_user_information(filename):
usernames = []
passwords = []
balances = []
try:
with open(filename, "r") as file:
lines = file.readlines()
for line in lines[2:]:
data = line.strip().split()
if len(data) == 3:
usernames.append(data[0])
passwords.append(data[1])
balances.append(float(data[2]))
except FileNotFoundError:
print("User information file not found. A new file will be created.")
return usernames, passwords, balances
def write_user_information(filename, usernames, passwords, balances):
with open(filename, "w") as file:
file.write("userName passWord Balance\n")
file.write("========================\n")
for i in range(len(usernames)):
file.write(f"{usernames[i]:<12} {passwords[i]:<12} {balances[i]:<}\n")
def login(usernames, passwords):
username_input = input("Enter username: ")
password_input = input("Enter password: ")
for i, username in enumerate(usernames):
if username == username_input and passwords[i] == password_input:
return i
print("Invalid username or password. Please try again.")
return None
def deposit(balances, user_index):
try:
amount = float(input("Enter the amount to deposit: "))
balances[user_index] += amount
print(f"Deposit successful. New balance: ${balances[user_index]:.2f}")
except ValueError:
print("Invalid amount entered. Deposit failed.")
def withdraw(balances, user_index):
try:
amount = float(input("Enter the amount to withdraw: "))
if amount > balances[user_index]:
print("Insufficient funds. Withdrawal failed.")
else:
balances[user_index] -= amount
print(f"Withdrawal successful. New balance: ${balances[user_index]:.2f}")
except ValueError:
print("Invalid amount entered. Withdrawal failed.")
def show_balance(balances, user_index):
print(f"Current balance: ${balances[user_index]:.2f}")
def add_new_user(usernames, passwords, balances):
new_username = input("Enter new username: ")
new_password = input("Enter new password: ")
try:
new_balance = float(input("Enter initial balance: "))
usernames.append(new_username)
passwords.append(new_password)
balances.append(new_balance)
print(f"New user {new_username} added with balance: ${new_balance:.2f}")
except ValueError:
print("Invalid balance entered. User addition failed.")
def main():
filename = "UserInformation.txt"
usernames, passwords, balances = read_user_information(filename)
user_index = None
while user_index is None:
user_index = login(usernames, passwords)
while True:
print("\nMenu:")
print("D - Deposit Money")
print("W - Withdraw Money")
print("B - Display Balance")
print("C - Change User")
print("A - Add New Client")
print("E - Exit")
choice = input("Enter your choice: ").upper()
if choice == 'D':
deposit(balances, user_index)
elif choice == 'W':
withdraw(balances, user_index)
elif choice == 'B':
show_balance(balances, user_index)
elif choice == 'C':
user_index = None
while user_index is None:
user_index = login(usernames, passwords)
elif choice == 'A':
add_new_user(usernames, passwords, balances)
elif choice == 'E':
write_user_information(filename, usernames, passwords, balances)
print("Exiting program. User information updated.")
break
else:
print("Invalid option. Please try again.")
if __name__ == "__main__":
main()



Step by step
Solved in 4 steps with 3 images

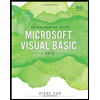
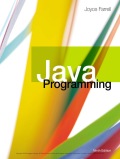
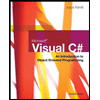
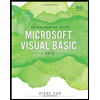
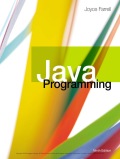
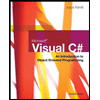