Project, with two attributes; projectNo (int) & location (string). Add the following: a parameterized constructor that initializes both fields getters toString() Supervisor, with two attributes; jobID (int) & name (string). A supervisor also has a list of projects. Add the following: a parameterized constructor that initializes jobId and name fields addProject(Project) method that adds the project object to the list of projects (if not null) terminateAllProjectsIn(String) method that searches for projects located in the passed location from the projects list, and if found, the method should remove these objects from the list and print a message “XX Project(s) terminated”. If the supervisor does not have projects in this location, your method should print the message “No current projects in ----------------” (----- means the passed location) ** toString method that returns the doctor details and the details of his/her patients. ** NOTE: you cannot iterate the list and remove its elements at the same time. To do so, you will need to create a temporary list; that is a copy of the original: ArrayList tmp = (ArrayList) projects.clone(); Iterate the temporary list and perform the changes on the original. Copy the class ProjectTest to the same package and make sure it runs and gives this output. App.java: public class ProjectTest { public static void main(String[] args) { Supervisor sup1 = new Supervisor(1, "Ali"); sup1.addProject(new Project(11, "Doha")); sup1.addProject(new Project(22, "Khor")); sup1.addProject(new Project(33, "Wakra")); sup1.addProject(new Project(44, "doha")); sup1.addProject(new Project(55, "DOHA")); System.out.println(sup1); System.out.println("_______________________\n"); sup1.terminateAllProjectsIn("Doha"); System.out.println("_______________________\n"); System.out.println(sup1); System.out.println("_______________________\n"); sup1.terminateAllProjectsIn("thumama"); System.out.println("_______________________\n"); System.out.println(sup1);
Given the class App below, write the following classes such that the main class (App) runs with no errors, and gives the output indicated by the screenshot below.
You will need to create the classes:
- Project, with two attributes; projectNo (int) & location (string). Add the following:
- a parameterized constructor that initializes both fields
- getters
- toString()
- Supervisor, with two attributes; jobID (int) & name (string). A supervisor also has a list of projects. Add the following:
- a parameterized constructor that initializes jobId and name fields
- addProject(Project) method that adds the project object to the list of projects (if not null)
- terminateAllProjectsIn(String) method that searches for projects located in the passed location from the projects list, and if found, the method should remove these objects from the list and print a message “XX Project(s) terminated”. If the supervisor does not have projects in this location, your method should print the message “No current projects in ----------------” (----- means the passed location) **
- toString method that returns the doctor details and the details of his/her patients.
** NOTE: you cannot iterate the list and remove its elements at the same time. To do so, you will need to create a temporary list; that is a copy of the original:
ArrayList<Project> tmp = (ArrayList<Project>) projects.clone();
Iterate the temporary list and perform the changes on the original.
Copy the class ProjectTest to the same package and make sure it runs and gives this output.
App.java:
public class ProjectTest {
public static void main(String[] args) {
Supervisor sup1 = new Supervisor(1, "Ali");
sup1.addProject(new Project(11, "Doha"));
sup1.addProject(new Project(22, "Khor"));
sup1.addProject(new Project(33, "Wakra"));
sup1.addProject(new Project(44, "doha"));
sup1.addProject(new Project(55, "DOHA"));
System.out.println(sup1);
System.out.println("_______________________\n");
sup1.terminateAllProjectsIn("Doha");
System.out.println("_______________________\n");
System.out.println(sup1);
System.out.println("_______________________\n");
sup1.terminateAllProjectsIn("thumama");
System.out.println("_______________________\n");
System.out.println(sup1);
}
}

Step by step
Solved in 2 steps with 1 images

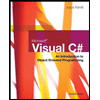
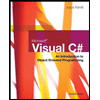