Programming Assignment: Average Miles Per GallonProblem Statement:In this assignment, you will write a program that computes fuel efficiency of a multi-leg journeyfor a vehicle. The program 1.Asksuser for the starting odometer reading (in miles). Using a while loop validates that the reading is positive, if not, prompts the user to enter a valid mileage value. 2.Next a.Asksthe user for odometer reading and amount of fuel in gallons used for the next leg ofjourney. b.Validates that the odometer reading is more than the last odometer reading and that the fuel is apositive number, if not prompts the user again till valid values are entered. c.Computes the miles per gallon (mpg) for this leg.d.Prints the mpg for this leg. 3.Asks user if there are additional legs for which the program should continue. a.If yes, repeats step 2 b.Else prints the average mpg of the entire journey by dividing the total miles travelled over all the legs by the total fuel consumed in all the legs. (It is NOT the average of all the mpgs from the different legs) NOTE: •To help you plan your code, a high level program outlinefor this problem is included. You can structureyour code based on this pseudo code. •Make sure your program works with multiple legs (not just 2 or 3). •The final average MPG should be calculated as (total miles travelled during all the legs of the journey)/ (total fuel consumed by all the legs of the journey)It is NOT calculated as the average of all the individual MPGs. •At the end, sample runof the program areshown. You should test your program with theinputs shown in the samples and check that your calculations are correct.Save your program to a file with a name of the format first_last_AvgMPG.py. Submit this file. Pseudocode: Print greetingPrompt the userfor starting odometer readingUse a while loop to validate thatstarting odometer readingis a positive number. Initialize variables: last odometer reading, current odometer reading, leg number,total fuel, moreInputWhile moreInput == ‘y’ Prompt the user for new odometer reading and fuel consumed If fuel is positive and new odometer reading > last odometer reading: Calculate MPG for this legusing mpg = (new odometer –last odometer) / fuel Print MPG for this legUpdate last odometer reading, total fuel, leg number Ask if user wants to continue(save user response in moreInput) ElsePrint message saying fuel should be positive and new odometer reading should be greater than last odometer reading (see the first sample run below) Print number of legsCalculate average MPG over entire journey(= Total miles travelled / total fuel consumed) Print average MPG over entire journey Print Bye message.
Programming Assignment: Average Miles Per GallonProblem Statement:In this assignment, you will write a program that computes fuel efficiency of a multi-leg journeyfor a vehicle. The program 1.Asksuser for the starting odometer reading (in miles). Using a while loop validates that the reading is positive, if not, prompts the user to enter a valid mileage value. 2.Next a.Asksthe user for odometer reading and amount of fuel in gallons used for the next leg ofjourney. b.Validates that the odometer reading is more than the last odometer reading and that the fuel is apositive number, if not prompts the user again till valid values are entered. c.Computes the miles per gallon (mpg) for this leg.d.Prints the mpg for this leg. 3.Asks user if there are additional legs for which the program should continue. a.If yes, repeats step 2 b.Else prints the average mpg of the entire journey by dividing the total miles travelled over all the legs by the total fuel consumed in all the legs. (It is NOT the average of all the mpgs from the different legs) NOTE: •To help you plan your code, a high level program outlinefor this problem is included. You can structureyour code based on this pseudo code. •Make sure your program works with multiple legs (not just 2 or 3). •The final average MPG should be calculated as (total miles travelled during all the legs of the journey)/ (total fuel consumed by all the legs of the journey)It is NOT calculated as the average of all the individual MPGs. •At the end, sample runof the program areshown. You should test your program with theinputs shown in the samples and check that your calculations are correct.Save your program to a file with a name of the format first_last_AvgMPG.py. Submit this file. Pseudocode: Print greetingPrompt the userfor starting odometer readingUse a while loop to validate thatstarting odometer readingis a positive number. Initialize variables: last odometer reading, current odometer reading, leg number,total fuel, moreInputWhile moreInput == ‘y’ Prompt the user for new odometer reading and fuel consumed If fuel is positive and new odometer reading > last odometer reading: Calculate MPG for this legusing mpg = (new odometer –last odometer) / fuel Print MPG for this legUpdate last odometer reading, total fuel, leg number Ask if user wants to continue(save user response in moreInput) ElsePrint message saying fuel should be positive and new odometer reading should be greater than last odometer reading (see the first sample run below) Print number of legsCalculate average MPG over entire journey(= Total miles travelled / total fuel consumed) Print average MPG over entire journey Print Bye message.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter5: Repetition Statements
Section5.5: A Closer Look: Loop Programming Techniques
Problem 14E
Related questions
Question
I'm trying to get my code to function as described and have only met two of the requirements. I can not seem to get my previous user inputs to save.
this should be a simple script saving user input previously entered in the while loop.
please do respond in cursive*****
what would you change in the script I provided? should I move my break statement?
here are the requirements ( I am also attaching my code and the desired outcome/example) *please look at both
Programming Assignment: Average Miles Per GallonProblem Statement:In this assignment, you will write a program that computes fuel efficiency of a multi-leg journeyfor a vehicle. The program
1.Asksuser for the starting odometer reading (in miles). Using a while loop validates that the reading is positive, if not, prompts the user to enter a valid mileage value.
2.Next
a.Asksthe user for odometer reading and amount of fuel in gallons used for the next leg ofjourney.
b.Validates that the odometer reading is more than the last odometer reading and that the fuel is apositive number, if not prompts the user again till valid values are entered.
c.Computes the miles per gallon (mpg) for this leg.d.Prints the mpg for this leg.
3.Asks user if there are additional legs for which the program should continue.
a.If yes, repeats step 2
b.Else prints the average mpg of the entire journey by dividing the total miles travelled over all the legs by the total fuel consumed in all the legs. (It is NOT the average of all the mpgs from the different legs)
NOTE:
•To help you plan your code, a high level program outlinefor this problem is included. You can structureyour code based on this pseudo code.
•Make sure your program works with multiple legs (not just 2 or 3).
•The final average MPG should be calculated as (total miles travelled during all the legs of the journey)/ (total fuel consumed by all the legs of the journey)It is NOT calculated as the average of all the individual MPGs.
•At the end, sample runof the program areshown. You should test your program with theinputs shown in the samples and check that your calculations are correct.Save your program to a file with a name of the format first_last_AvgMPG.py. Submit this file.
Pseudocode:
Print greetingPrompt the userfor starting odometer readingUse a while loop to validate thatstarting odometer readingis a positive number.
Initialize variables:
last odometer reading, current odometer reading, leg number,total fuel,
moreInputWhile moreInput == ‘y’
Prompt the user for new odometer reading and fuel consumed
If fuel is positive and new odometer reading > last odometer reading:
Calculate MPG for this legusing mpg = (new odometer –last odometer) / fuel
Print MPG for this legUpdate last odometer reading, total fuel, leg number
Ask if user wants to continue(save user response in moreInput)
ElsePrint message saying fuel should be positive and new odometer reading should be greater than last odometer reading (see the first sample run below)
Print number of legsCalculate average MPG over entire journey(= Total miles travelled / total fuel consumed)
Print average MPG over entire journey
Print Bye message.

Transcribed Image Text:*mpg ave 2.py - C:\Users\steph\OneDrive\Desktop\mpg ave 2.py (3.9.4)*
g ave
File Edit Format Run Options Window Help
#greeting banner
print ("Welcome to the Average MPG Calculator.")
print ()
#set varibles
dash="-"* 60
another_trip="Y"
odom=int (input ("Please enter the starting odometer reading in miles: "))
print ()
print (dash)
#another_trip =input ("continue (y/n) ? ") loop
while another_trip.lower ()
#odoml=int (input ("Please enter the new odometer reading in miles for leg: "))
"y":
==
#gallons=float (input ("Please enter the fuel consumed in gallons for leg: "))
#validate varibles in while loop
while True:
odoml=int (input ("Please enter the new odometer reading in miles for leg: "))
gallons=float (input ("Please enter the fuel consumed in gallons for leg: "))
#while ((odoml > odom ) and (gallons > 0) ) :
#odoml=int (input ("Please enter the new odometer reading in miles for leg #1: "))
#gallons=float (input ("Please enter the fuel consumed in gallons for leg #1: "))
mpg=float ( (odoml - odom) /gallons)
total miles= (odom + odoml)
if ((odoml > odom ) and (gallons > 0)) :
leg=0
leg+=1
print ("MPG for leg #",leg, "="
break
else:
print ("Fuel consumed needs to be positive and new odometer reading need to be higher than", odom)
#ask to continue
#another_trip -input ("continue (y/n)? ")
another_trip=input ("Continue (y/n) ? ")
if (another_trip.lower () !="y"):
print ("total legs of trip:", leg)
print ("average MPG:", mpg)

Transcribed Image Text:Sample 1 with some invalid inputs
a Python 3.8.1 Shell
Eile Edit Shell Debug Options Window Help
Welcome to the Average MPG Calculator
Please enter the starting odometer reading in miles: 10000
Please enter new odometer reading in miles for leg #1: 10060
Please enter fuel consumed in gallons for leg #1: 2.5
MPG for leg # 1 = 24.0
Continue (y/n) ? y
Please enter new odometer reading in miles for leg #2: 10020
Please enter fuel consumed in gallons for leg #2: 2.0
Fuel consumed needs to positive and new odometer reading needs to be higher than 10060.
Please enter new odometer reading in miles for leg #2: 10090
Please enter fuel consumed in gallons for leg #2: -1
Fuel consumed needs to positive and new odometer reading needs to be higher than 10060.
Please enter new odometer reading in miles for leg #2: 10090
Please enter fuel consumed in gallons for leg #2: 2.o
MPG for leg # 2 = 15.0
Continue (y/n) ? n
Total number of legs in the journey: 2
Final average MPG for the entire journey: 20.0
Bye!
>>>
Ln: 61 Col: 4
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
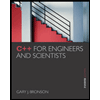
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
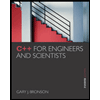
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr