Poker Game Use the functions developed in exercise 7.24 in the book to write a program that deals two five-card poker hands, evaluates each, and determines which is the better hand. Create functions that: a. Determine whether the hand contains a pair. b. Determine whether the hand contains two pairs. c. Determine whether the hand contains three of a kind (e.g., three jacks). d. Determine whether the hand contains four of a kind (e.g., four aces). e. Determine whether the hand contains a flush (i.e., all five cards of the same suit). f. Determine whether the hand contains a straight (i.e., five cards of consecutive face values). I have attached the code at the for exercise 7.24 however I do recommend that you read through the chapter in order to better understand why the algorithm is written up that way.
Poker Game Use the functions developed in exercise 7.24 in the book to write a program that deals two five-card poker hands, evaluates each, and determines which is the better hand. Create functions that: a. Determine whether the hand contains a pair. b. Determine whether the hand contains two pairs. c. Determine whether the hand contains three of a kind (e.g., three jacks). d. Determine whether the hand contains four of a kind (e.g., four aces). e. Determine whether the hand contains a flush (i.e., all five cards of the same suit). f. Determine whether the hand contains a straight (i.e., five cards of consecutive face values). I have attached the code at the for exercise 7.24 however I do recommend that you read through the chapter in order to better understand why the algorithm is written up that way.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
The below code is the code that it’s mentioned in the question!
Please write your in C language, and put it in text so i can copy it!
Thanks

Transcribed Image Text:Poker Game
Use the functions developed in exercise 7.24 in the book to write a program that deals two
five-card poker hands, evaluates each, and determines which is the better hand.
Create functions that:
a. Determine whether the hand contains a pair.
b. Determine whether the hand contains two pairs.
c. Determine whether the hand contains three of a kind (e.g., three jacks).
d. Determine whether the hand contains four of a kind (e.g., four aces).
e. Determine whether the hand contains a flush (i.e., all five cards of the same suit).
f. Determine whether the hand contains a straight (i.e., five cards of consecutive face
values).
I have attached the code at the for exercise 7.24 however I do recommend that you read through
the chapter in order to better understand why the algorithm is written up that way.
![#include <stdio.h>
#include <stdlib.h>
8
9
10 #include <time.h>
#define SUITS 4
11
12
#define FACES 13
13 #define CARDS 52
1 // prototypes
15 void shuffle(unsigned int wDeck [) [FACES]); // shuffling modifies wDeck
16 void deal (unsigned int weck[] [FACES], const char *wFace[],
17 const char *wSuit[]); // dealing doesn't modify the arrays
18 int main(void){
19 // initialize deck array
20 unsigned int deck [ SUITS] [FACES] = {0};
srand (time (NULL)); // seed random-number generator
shuffle(deck); // shuffle the deck
// initialize suit array
24
19 77
21
A Implicit conversion loses integer precision: 'time_t' (aka 'long') to 'unsig
23
const char *suit[SUITS] =
{"Hearts", "Diamonds", "Cclubs", "Spades"};
26 // initialize face array
const char *face[FACES]
28 {"Ace", "Deuce", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Jack", "Queen", "King"};
29 deal (deck, face, suit); // deal the deck
27
%3D
30 }
31
32
33 // shuffle cards in deck
34 void shuffle(unsigned int wDeck[][FACES]){
35 // for each of the cards, choose slot of deck randomly
for (size_t card = 1; card <= CARDS; ++card) {
size_t row; // row number
size_t column; // column number
// choose new random location until unoccupied slot found
36
37
38
do {
row = rand () % SUITS;
column = rand () % FACES;
40
41
42
43
} while(wDeck[ row] [column] != 0);
44 // place card number in chosen slot of deck
wDeck[row][column]= card;
}
45
AImplicit conversion loses integer precision: 'size_t' (aka 'unsigned long') to 'unsig
46
47 }
// deal cards in deck
48
49
void deal (unsigned int wDeck [] [FACES], const char *wFace[], const char *wSuit[]){
50
//deal each of the cards
for (size_t card = 1; card <= CARDS; ++card) {
51
52
// loop through rows of wDeck
for (size_t row = 0; row < SUITS; ++row) {
53
54 // loop through columns of wDeck for current row
55
for (size_t column = 0; column < FACES; ++column) {
56 // if slot contains current card, display card
57
if (wDeck[row][column] == card) { printf("%5s of %-8s%c", wFace[column], wSuit[row],card % 2 == 0 ? '\n': '\t'); // 2-column format](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F82c30bff-0aeb-441d-8230-5a24750639a5%2F99b1f404-2e95-4090-9dbc-59ab98b3fb59%2F4fhknpk_processed.jpeg&w=3840&q=75)
Transcribed Image Text:#include <stdio.h>
#include <stdlib.h>
8
9
10 #include <time.h>
#define SUITS 4
11
12
#define FACES 13
13 #define CARDS 52
1 // prototypes
15 void shuffle(unsigned int wDeck [) [FACES]); // shuffling modifies wDeck
16 void deal (unsigned int weck[] [FACES], const char *wFace[],
17 const char *wSuit[]); // dealing doesn't modify the arrays
18 int main(void){
19 // initialize deck array
20 unsigned int deck [ SUITS] [FACES] = {0};
srand (time (NULL)); // seed random-number generator
shuffle(deck); // shuffle the deck
// initialize suit array
24
19 77
21
A Implicit conversion loses integer precision: 'time_t' (aka 'long') to 'unsig
23
const char *suit[SUITS] =
{"Hearts", "Diamonds", "Cclubs", "Spades"};
26 // initialize face array
const char *face[FACES]
28 {"Ace", "Deuce", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Jack", "Queen", "King"};
29 deal (deck, face, suit); // deal the deck
27
%3D
30 }
31
32
33 // shuffle cards in deck
34 void shuffle(unsigned int wDeck[][FACES]){
35 // for each of the cards, choose slot of deck randomly
for (size_t card = 1; card <= CARDS; ++card) {
size_t row; // row number
size_t column; // column number
// choose new random location until unoccupied slot found
36
37
38
do {
row = rand () % SUITS;
column = rand () % FACES;
40
41
42
43
} while(wDeck[ row] [column] != 0);
44 // place card number in chosen slot of deck
wDeck[row][column]= card;
}
45
AImplicit conversion loses integer precision: 'size_t' (aka 'unsigned long') to 'unsig
46
47 }
// deal cards in deck
48
49
void deal (unsigned int wDeck [] [FACES], const char *wFace[], const char *wSuit[]){
50
//deal each of the cards
for (size_t card = 1; card <= CARDS; ++card) {
51
52
// loop through rows of wDeck
for (size_t row = 0; row < SUITS; ++row) {
53
54 // loop through columns of wDeck for current row
55
for (size_t column = 0; column < FACES; ++column) {
56 // if slot contains current card, display card
57
if (wDeck[row][column] == card) { printf("%5s of %-8s%c", wFace[column], wSuit[row],card % 2 == 0 ? '\n': '\t'); // 2-column format
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 10 images

Recommended textbooks for you
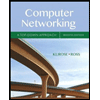
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
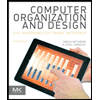
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
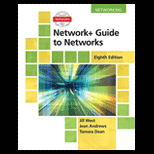
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
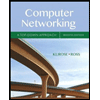
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
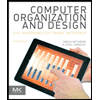
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
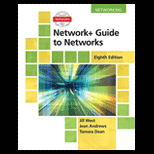
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
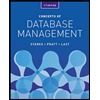
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
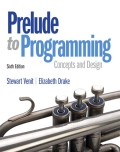
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
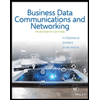
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY