PJ 5 – Simple Calculator Please write a C++ program that can perform the following 6 operators for 2 numbers: N1 and N2. You need to prompt the user to enter a number, an operator, and another number. To simplify your program, please declare N1, N2, and result as double-floating numbers, and operator as char. Please see the sample test below to design your application program properly. This program is a simple calculator for users to enjoy. Please let your program keep running to accept more data for the next operation until the operator received is @. In other words, the operator @ is the only way to stop your program and thank the user. The O valid operators for this simple calculator are as follows: 1. + for addition of N1 and N2. Therefore, result = (N1 +N2). 2. - for subtraction of N2 from N1. Therefore, result = (N1 – N2). * for multiplication of N1 with N2. Therefore, result = (N1 * N2). 3.
PJ 5 – Simple Calculator Please write a C++ program that can perform the following 6 operators for 2 numbers: N1 and N2. You need to prompt the user to enter a number, an operator, and another number. To simplify your program, please declare N1, N2, and result as double-floating numbers, and operator as char. Please see the sample test below to design your application program properly. This program is a simple calculator for users to enjoy. Please let your program keep running to accept more data for the next operation until the operator received is @. In other words, the operator @ is the only way to stop your program and thank the user. The O valid operators for this simple calculator are as follows: 1. + for addition of N1 and N2. Therefore, result = (N1 +N2). 2. - for subtraction of N2 from N1. Therefore, result = (N1 – N2). * for multiplication of N1 with N2. Therefore, result = (N1 * N2). 3.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%

Transcribed Image Text:PJ 5 – Simple Calculator
Please write a C++ program that can perform the following 6 operators for 2 numbers: N1 and N2. You
need to prompt the user to enter a number, an operator, and another number. To simplify your program,
please declare N1, N2, and result as double-floating numbers, and operator as char. Please see the sample
test below to design your application program properly. This program is a simple calculator for users to
enjoy. Please let your program keep running to accept more data for the next operation until the operator
received is @. In other words, the operator @ is the only way to stop your program and thank the user.
6.
The
valid operators for this simple calculator are as follows:
1. + for addition of N1 and N2. Therefore, result = (N1 + N2).
- for subtraction of N2 from N1. Therefore, result = (N1 – N2).
3. * for multiplication of N1 with N2. Therefore, result = (N1 * N2).
4. / for floating-point division of N1 by N2. If N2 is 0, please say “The second number cannot be zero",
else result = (N1 / N2).
5. ^ for power N2 with base N1 (i.e., N1N2). Therefore, result = pow ( N1 , N2 ). // power function
pow(N1, N2) is a function call to compute N1 power N2.
6. % for integer division remainder (i.e., modulus) of N1 by N2. If N2 is zero, please
say “The second number cannot be zero", else result = ( int(N1) % int(N2) ).
int(N1) is a function call to convert N1 to an integer. % operator accepts only two integers.
2.
7. @ for stopping this calculator after printing a friendly thank-you message.
8. Otherwise, please say “Sorry, the operator
is not valid!". // Please show this invalid operator.
Your test case #1 must look exactly as follows including the data. You must also do test case #2 and test
case #3 with different sets of data. Each of your test case must cover all the 6 valid operators (at least once
for each) plus an invalid one, Each test case or test run must begin with a welcome message, and must end

Transcribed Image Text:Welcome to use the Simple Calculator of Dr. Simon Lin! + You must use your name!
1
Please enter your number, operator, and number > 3.3 + 5.5
Result: 3.3 + 5.5 = 8.8
2=
Please enter your number, operator, and number > 3.3
Result: 3.3 – 5.5 = -2.2
5.5
3====
Please enter your number, operator, and number > 3.3 *
Result: 3.3 * 5.5 = 18.15
5.5
4=
Please enter your number, operator, and number > 3.3 / 5.5
Result: 3.3 / 5.5 = 0.6
5=
Please enter your number, operator, and number > 3.3 ^ 5.5
Result: 3.3 ^ 5.5 = 710.93
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
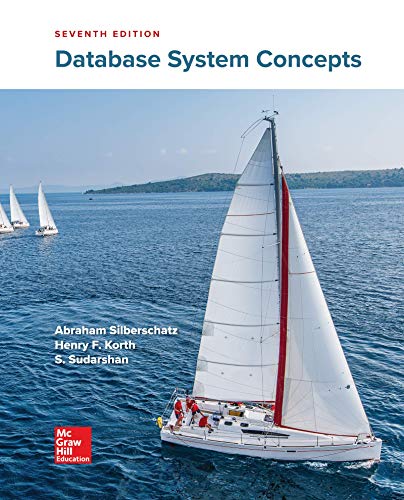
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
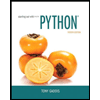
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
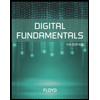
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
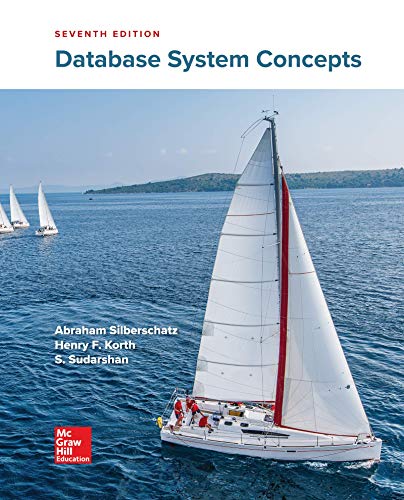
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
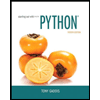
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
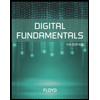
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
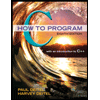
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
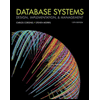
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
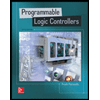
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education