my code # Initialize the required CS credits earned earned_credit_hours = 0 # Print the header print("--------------------------------") print(" UIC CS Track") print("--------------------------------\n") # QUESTION 1: Are you a CS major? major_answer = input("QUESTION 1\nAre you a CS major? (Yes or No): ").upper() if major_answer == "YES": # QUESTION 2: Have you taken ENGR100? engr100_answer = input("\nQUESTION 2\nHave you taken ENGR100? (Yes or No): ").upper() # QUESTION 3: Have you taken CS111 and CS112? cs111_answer = input("\nQUESTION 3\nHave you taken CS111? (Yes or No): ").upper() # Check if CS111 is taken if cs111_answer == "YES": earned_credit_hours += 3 print("\nQUESTION 4") cs141_answer = input("Have you taken CS141? (Yes or No): ").upper() if cs141_answer == "YES": earned_credit_hours += 3 print("\nQUESTION 5") cs151_answer = input("Have you taken CS151? (Yes or No): ").upper() if cs151_answer == "YES": earned_credit_hours += 3 print("\nQUESTION 6") cs211_answer = input("Have you taken CS211? (Yes or No): ").upper() if cs211_answer == "YES": earned_credit_hours += 3 print("\nQUESTION 7") cs251_answer = input("Have you taken CS251? (Yes or No): ").upper() if cs251_answer == "YES": earned_credit_hours += 4 print("Have you taken CS277? (Yes or No): ", end='') cs277_answer = input().strip().upper() if cs277_answer == "YES": earned_credit_hours += 3 print("Have you taken CS377? (Yes or No): ", end='') cs377_answer = input().strip().upper() if cs377_answer == "YES": earned_credit_hours += 3 print("Have you taken CS401? (Yes or No): ", end='') cs401_answer = input().strip().upper() if cs401_answer == "YES": earned_credit_hours += 3 print("\nQUESTION 8") cs261_answer = input("Have you taken CS261? (Yes or No): ").upper() if cs261_answer == "YES": earned_credit_hours += 4 # Print the summary for CS majors print("\n--------------------------------") print(" Summary") print("--------------------------------\n") print("You are a CS major!") if engr100_answer == "NO": print("Do not forget to take ENGR100!") print("\nRequired CS credits earned:", earned_credit_hours, end=".\n") else: # Print the summary for non-CS majors print("\n--------------------------------") print(" Summary") print("--------------------------------\n") print("Sadly, you are not a CS major.") # Goodbye message print("\nThank you for using App!") print("Closing app...") Do this In a file called main.py, implement a program that asks if the user has taken any of the "Level 2-3" CS classes in addition to the questions from the previous milestone, and then prints a short summary based on the user's replies. The program should have up to 11 input prompts the string values of the user's answers. Firstly, copy and paste your code from the previous milestone. Your program should continue what you did in the previous milestone by asking up to eight additional questions. As you can see in the diagram below, "Level 2" contains CS141 and CS151 while "Level 3" contains CS211, CS251, CS261, CS277, CS377 and CS401. Secondly, here's functionality you need to implement: If the user has not taken any of "Level 1" CS courses, the program skips to the summary. Else, your program should ask if the user has taken CS141 and CS151. If the user has not taken all "Level 2" CS courses the program skips to the summary. Else, your program should ask if the user has taken CS211, CS251 etc. If the user has taken CS251, the program should ask if the user has taken CS277. If yes, the program should also ask if the user has taken CS377. Regardless of the replies on CS277 and CS377 questions, the program should ask about CS401 as well. This part is different from anything you did before.
my code
In a file called main.py, implement a program that asks if the user has taken any of the "Level 2-3" CS classes in addition to the questions from the previous milestone, and then prints a short summary based on the user's replies. The program should have up to 11 input prompts the string values of the user's answers.
Firstly, copy and paste your code from the previous milestone. Your program should continue what you did in the previous milestone by asking up to eight additional questions. As you can see in the diagram below, "Level 2" contains CS141 and CS151 while "Level 3" contains CS211, CS251, CS261, CS277, CS377 and CS401.
Secondly, here's functionality you need to implement:
- If the user has not taken any of "Level 1" CS courses, the program skips to the summary. Else, your program should ask if the user has taken CS141 and CS151.
- If the user has not taken all "Level 2" CS courses the program skips to the summary. Else, your program should ask if the user has taken CS211, CS251 etc.
- If the user has taken CS251, the program should ask if the user has taken CS277. If yes, the program should also ask if the user has taken CS377. Regardless of the replies on CS277 and CS377 questions, the program should ask about CS401 as well. This part is different from anything you did before.


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

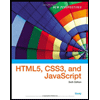
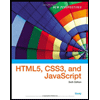