LAB 6.2 Introduction to Pass by Value Retrieve program newproverb.cpp from the Lab 6.1 folder. The code is as follows: // This program will allow the user to input from the keyboard // whether the last word to the following proverb should be party or country: // "Now is the time for all good men to come to the aid of their ___" // Inputting a 1 will use the word party. Any other number will use the word country. // PLACE YOUR NAME HERE #include #include using namespace std; // Fill in the prototype of the function writeProverb. int main() { int wordCode; cout << "Given the phrase:" << endl; cout << "Now is the time for all good men to come to the aid of their ___" << endl; cout << "Input a 1 if you want the sentence to be finished with party" << endl; cout << "Input any other number for the word country" << endl; cout << "Please input your choice now" << endl; cin >> wordCode; cout << endl; writeProverb(wordCode); return 0; } // ****************************************************************************** // writeProverb // // task: This function prints a proverb. The function takes a number // from the call. If that number is a 1 it prints "Now is the time // for all good men to come to the aid of their party." // Otherwise, it prints "Now is the time for all good men // to come to the aid of their country." // data in: code for ending word of proverb (integer) // data out: no actual parameter altered // // ***************************************************************************** void writeProverb(int number) { // Fill in the body of the function to accomplish what is described above } Exercise 1: Some people know this proverb as “Now is the time for all good men to come to the aid of their country” while others heard it as “Now is the time for all good men to come to the aid of their party.” This program will allow the user to choose which way they want it printed. Fill in the blanks of the program to accomplish what is described in the program comments. What happens if you inadvertently enter a float such as -3.97? Exercise 2: Change the program so that an input of 1 from the user will print “party” at the end, a 2 will print “country” and any other number will be invalid so that the user will need to enter a new choice. sample run: Given the phrase: Now is the time for all good men to come to the aid of their___ input a 1 if you want the sentence to be finished with party input a 2 if you want the sentence to be finished with county please input your choice now 4 i'm sorry but that is an incorrect choice; Please input a 1 or 2 2 Now is the time for all good men to come to the aid of their country Exercise 3: Change the previous program so the user may input the word to end the phrase. The string holding the user’s input word will be passed to the proverb function instead of passing a number to it. Notice that this change requires you to change the proverb function heading and the prototype as well as the call to the function. Sample Run: Given the phrase: Now is the time for all good men to come to the aid of their Please input the word you would like to have finish the proverb family Now is the time for all good men to come to the aid of their family
LAB 6.2 Introduction to Pass by Value
Retrieve
// This program will allow the user to input from the keyboard
// whether the last word to the following proverb should be party or country:
// "Now is the time for all good men to come to the aid of their ___"
// Inputting a 1 will use the word party. Any other number will use the word country.
// PLACE YOUR NAME HERE
#include <iostream>
#include <string>
using namespace std;
// Fill in the prototype of the function writeProverb.
int main()
{
int wordCode;
cout << "Given the phrase:" << endl;
cout << "Now is the time for all good men to come to the aid of their ___"
<< endl;
cout << "Input a 1 if you want the sentence to be finished with party"
<< endl;
cout << "Input any other number for the word country" << endl;
cout << "Please input your choice now" << endl;
cin >> wordCode;
cout << endl;
writeProverb(wordCode);
return 0;
}
// ******************************************************************************
// writeProverb
//
// task: This function prints a proverb. The function takes a number
// from the call. If that number is a 1 it prints "Now is the time
// for all good men to come to the aid of their party."
// Otherwise, it prints "Now is the time for all good men
// to come to the aid of their country."
// data in: code for ending word of proverb (integer)
// data out: no actual parameter altered
//
// *****************************************************************************
void writeProverb(int number)
{
// Fill in the body of the function to accomplish what is described above
}
Exercise 1: Some people know this proverb as “Now is the time for all good
men to come to the aid of their country” while others heard it as “Now is
the time for all good men to come to the aid of their party.” This program
will allow the user to choose which way they want it printed. Fill in the
blanks of the program to accomplish what is described in the program
comments. What happens if you inadvertently enter a float such as -3.97?
Exercise 2: Change the program so that an input of 1 from the user will print
“party” at the end, a 2 will print “country” and any other number will be
invalid so that the user will need to enter a new choice.
sample run:
Given the phrase:
Now is the time for all good men to come to the aid of their___
input a 1 if you want the sentence to be finished with party
input a 2 if you want the sentence to be finished with county
please input your choice now
4
i'm sorry but that is an incorrect choice; Please input a 1 or 2
2
Now is the time for all good men to come to the aid of their country
Exercise 3: Change the previous program so the user may input the word to
end the phrase. The string holding the user’s input word will be passed to the
proverb function instead of passing a number to it. Notice that this change
requires you to change the proverb function heading and the prototype as
well as the call to the function.
Sample Run:
Given the phrase:
Now is the time for all good men to come to the aid of their
Please input the word you would like to have finish the proverb
family
Now is the time for all good men to come to the aid of their family

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

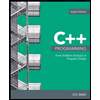
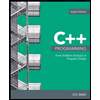