Java: Write a complete method to create an array of random integers. Method Specification The method takes in three integer parameters: the array size, the lower bound, and the upper bound. The method also takes in a boolean parameter. The method creates and returns an integer array of the specified size that contains random numbers between the lower and upper bound., using these rules: The lower bound is always inclusive. If the boolean parameter is true, the upper bound is also inclusive. If the boolean parameter is false, the upper bound is not inclusive (meaning it is exclusive). If any of the parameters are invalid, null is returned. Driver/Tester Program I have provided you with a driver/tester program that you can use to test your code before submitting. Paste your code into the placeholder at the top of the file. I strongly recommend running this program before you submit your homework. The program is designed to help you catch common errors and fix them before submitting. Run the program multiple times since random number selection is involved. Each time, review the output to see if your actual results match what was expected. A "**********TEST FAILED" message should also print if a test case fails. The driver program has some tester methods at the end. These method are used to streamline the running of multiple repeated tests. You don't need to understand, review, or modify these methods. The driver program was too long to paste so it is in two pictures. See attached images for the driver program.
Java:
Write a complete method to create an array of random integers.
Method Specification
The method takes in three integer parameters: the array size, the lower bound, and the upper bound. The method also takes in a boolean parameter.
The method creates and returns an integer array of the specified size that contains random numbers between the lower and upper bound., using these rules:
- The lower bound is always inclusive.
- If the boolean parameter is true, the upper bound is also inclusive.
- If the boolean parameter is false, the upper bound is not inclusive (meaning it is exclusive).
If any of the parameters are invalid, null is returned.
Driver/Tester Program
I have provided you with a driver/tester program that you can use to test your code before submitting. Paste your code into the placeholder at the top of the file.
I strongly recommend running this program before you submit your homework. The program is designed to help you catch common errors and fix them before submitting. Run the program multiple times since random number selection is involved. Each time, review the output to see if your actual results match what was expected. A "**********TEST FAILED" message should also print if a test case fails.
The driver program has some tester methods at the end. These method are used to streamline the running of multiple repeated tests. You don't need to understand, review, or modify these methods. The driver program was too long to paste so it is in two pictures. See attached images for the driver program.
![}
/* TESTER METHODS */
* The methods below are designed to help support the tests cases run from main. You don't
* need to use, modify, or understand these methods. You can safely ignore them. :)
public static void testGenerateRandomArrayWith InvalidParameters(int size, int low, int high, boolean inclusive, String testDescription) {
int[] array
generateRandomArray (size, low, high, inclusive);
System.out.println("\nExpected size of returned array: " + size);
String inclusiveString = inclusive? "] (inclusive)" : ") (EXCLUSIVE)";
System.out.println("Expected Range of values: (inclusive) [" + low + ",
if(array!=null) {
}
} else {
}
}
public static void testGenerateRandomArray(int size, int low, int high, boolean inclusive) {
int[] array
generateRandomArray (size, low, high, inclusive);
System.out.println("\nExpected size: " + size);
System.out.println(" Actual size: " + array.length);
if(size==0) {
+ high + inclusiveString);
System.out.println("**********TEST FAILED for invalid parameters. Returned array should be null. Test: " + test Description);
allTests Passed = false;
System.out.println("Expected result: null\n Actual result: " + array);
if(array!=null && array.length>0) {
allTests Passed = false;
System.out.print("*
}
return;
}
String inclusiveString = inclusive? "] (inclusive)" : ") (EXCLUSIVE)";
System.out.println("Expected Range: (inclusive) [" + low + ", ..., " + high + inclusiveString);
System.out.println("Actual Array: " + Arrays.toString(array));
if(array.length != size) {
}
}
}
boolean validNumbers = true;
int badNumber = -1;
for(int num: array) {
if (num < low) {
allTests Passed = false;
System.out.println("**********TEST FAILED: array is not the requested size");
validNumbers = false;
badNumber = num;
***TEST FAILED for requested size of 0. ");
if(inclusive && num > high) {
validNumbers = false;
}
if(!validNumbers) {
*/
badNumber = num;
} else if(!inclusive && num >= high) {
validNumbers = false;
badNumber = num;
allTestsPassed = false;
System.out.println("**********TEST FAILED: one or more numbers is outside of the range. One number outside the range is " + badNumber);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7024c4db-e4c4-44c2-8fab-3045baf15ef1%2F0c6158ba-c928-4a0d-9895-dfe1f40e432d%2Fvz2595b_processed.png&w=3840&q=75)
![import java.util.*;
public class Homework5BDriver {
public static int[] generateRandomArray (int size, int lower, int upper, boolean inclusive) {
// YOUR CODE HERE
return new int[] {}; // placeholder: replace with your own code when you write the method
}
public static void main(String[] args) {
System.out.println(".
System.out.println("
-TESTING generateRandomArray METHOD-
-");
*IMPORTANT NOTE: run these tests multiple times because they involve random numbers. \nThis will increase your chance of finding any bugs.");
// the parameters sent to the tester method are the same as the ones sent to the generateRandomArray method
// parameter 1: the size of the array
// parameter 2: the lower bound
// parameter 3: the upper bound
// parameter 4: whether or not the upper bound is inclusive
testGenerateRandomArray (20, 1, 10, true);
testGenerateRandomArray (20, -10, 0, true);
testGenerateRandomArray (8, -1, 1, true);
testGenerateRandomArray(100, 1, 10, false);
testGenerateRandomArray(16, 1, 5, true);
testGenerateRandomArray (15, 1, 5, false);
testGenerateRandomArray(18, 4, 5, false);
testGenerateRandomArray (12, 7, 7, true);
System.out.println("\n----
---TESTS WITH INVALID PARAMETERS---
----");
testGenerateRandomArrayWith Invalid Parameters (10, 4, 3, true, "lower bound is greater than upper bound");
testGenerateRandomArrayWithInvalid Parameters (10, 4, 4, false, "lower bound and upper bound are equal but the results should NOT be exlusive");
testGenerateRandomArrayWithInvalidParameters (10, 4, 2, false, "lower bound is greater than upper bound");
testGenerateRandomArrayWith Invalid Parameters(-1, 4, 14, true, "negative array size");
if (allTests Passed) {
} else {
}
System.out.println("\n----------Summary-
System.out.flush();
System.err.println("\n**********Summary*
}
private static boolean allTests Passed = true;
\nAll automated tests have passed. \nManually review your code for style. \nManually view the expected output to make sure it matches.");
ERROR: There is failure in at least one automated test. Review the output above for details.");](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7024c4db-e4c4-44c2-8fab-3045baf15ef1%2F0c6158ba-c928-4a0d-9895-dfe1f40e432d%2Flitqyx8_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

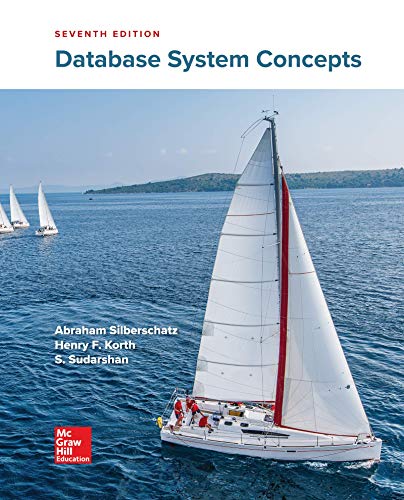
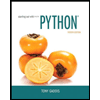
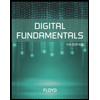
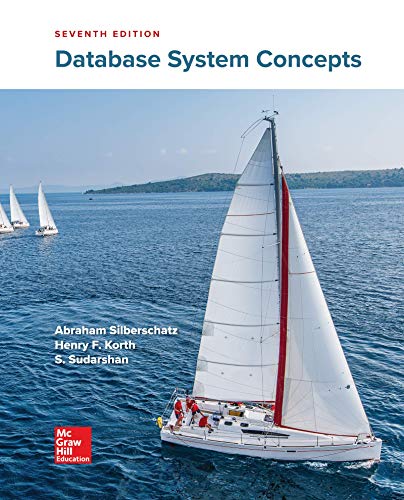
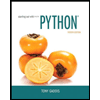
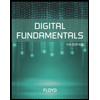
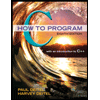
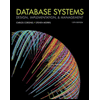
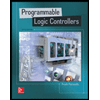