▪ Include the following information as comments at the top of your program (file name, your name, today's date, short description of the program) ▪ A prime number is a number that is only evenly divisible by itself and 1. ▪ For example: The number 5 is prime because it can only be evenly divided by 1 and 5. The number 6, however, is not prime because it can be divided evenly by 1, 2, 3, and 6. ▪ Write a program that performs the following tasks: ▪ Write the definition of a Boolean function isPrime that takes an input number and returns True if the number is prime and False otherwise. . In the main function, ▪ get user input - an integer number (n). ▪ Find all the numbers that are prime - from 1 up to the number input by the user, by calling the function isPrime for each number. ▪ Keep track of the count of the numbers that are prime numbers in a accumulator variable, . Finally, display the total number of prime numbers from 1 to n ▪ Your output should match the given sample runs. ▪ Note: isPrime is a Boolean Function. Follow the syntax for using a Boolean Function. Sample Run 1: Please enter an integer number: 200 There are 46 prime numbers from 1 to 200.
▪ Include the following information as comments at the top of your program (file name, your name, today's date, short description of the program) ▪ A prime number is a number that is only evenly divisible by itself and 1. ▪ For example: The number 5 is prime because it can only be evenly divided by 1 and 5. The number 6, however, is not prime because it can be divided evenly by 1, 2, 3, and 6. ▪ Write a program that performs the following tasks: ▪ Write the definition of a Boolean function isPrime that takes an input number and returns True if the number is prime and False otherwise. . In the main function, ▪ get user input - an integer number (n). ▪ Find all the numbers that are prime - from 1 up to the number input by the user, by calling the function isPrime for each number. ▪ Keep track of the count of the numbers that are prime numbers in a accumulator variable, . Finally, display the total number of prime numbers from 1 to n ▪ Your output should match the given sample runs. ▪ Note: isPrime is a Boolean Function. Follow the syntax for using a Boolean Function. Sample Run 1: Please enter an integer number: 200 There are 46 prime numbers from 1 to 200.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter5: Repetition Statements
Section: Chapter Questions
Problem 7PP
Related questions
Question
Hello. I'm using functions in my python code but I'm not sure what boolean function I should be using for prime numbers

Transcribed Image Text:▪ Include the following information as comments at the top of your program (file name, your name, today's date, short description of the program)
A prime number is a number that is only evenly divisible by itself and 1.
▪ For example: The number 5 is prime because it can only be evenly divided by 1 and 5. The number 6, however, is not prime because it can be divided evenly
by 1, 2, 3, and 6.
▪ Write a program that performs the following tasks:
Write the definition of a Boolean function isPrime that takes an input number and returns True if the number is prime and False otherwise.
▪ In the main function,
get user input - an integer number (n).
▪ Find all the numbers that are prime - from 1 up to the number input by the user, by calling the function isPrime for each number.
■
Keep track of the count of the numbers that are prime numbers in a accumulator variable,
■
Finally, display the total number of prime numbers from 1 to n
Your output should match the given sample runs.
Note: isPrime is a Boolean Function. Follow the syntax for using a Boolean Function.
Sample Run 1:
■
Please enter an integer number: 200
There are 46 prime numbers from 1 to 200.

Transcribed Image Text:1 # Rahma Seid
NT
2 # CSCI 1170-001
3 # 10/24/22
4
# CLA16
5
# The purpose of this program is to find the prime number, after the user inputs an integer
6
7 def IsPrime (integer):
8
9
10
11
12
13
14
15
16
3556NTO
if integer % 2 == 0:
return True
else:
return False
IsPrime(n)
min <= 1
max => 200
17
18 def main():
19
20
21
n = int(input("Please enter an integer number: "))
if IsPrime(n):
print(n, 'is prime.')
22
23
24
25
26 main()
else:
print(n, 'isn't prime.')
print (f" There are {IsPrime} prime numbers from {min} to {max}.")
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
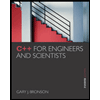
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
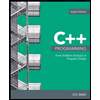
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
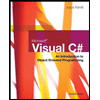
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
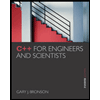
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
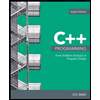
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
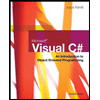
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage