In C++ Create a new project named lab9_1 . You will need to implement a Course class. Here is its UML diagram: Course - department : string - course_num : string - section : int - num_students : int - is_full : bool + Course() + Course(string, string, int, int) + setDepartment(string) : void + setNumber(string) : void + setSection(int) : void + setStudents(int) : void + getDepartment() const : string + getNumber() const : string + getSection() const : int + getStudents() const : int + print() const : void Create a sample file to read from: CSS 2A 1111 35 Additional information: The Course class has two constructors. Make sure you have default values for your default constructor. Each course maxes out at 40 students. Therefore, you need to make sure that there aren’t more than 40 students in a Course. You can choose how you handle situations where more than 40 students are added. Additionally, you should automatically set is_full to false or true, based on the number of students. If you’d like to include additional methods than those found in the UML diagram, feel free to do so. That’s just the minimum amount required, but helper/utility methods would be okay. You will also make use of the const keyword to indicate which methods are not modifying the object. You will read in the information from the file, and declare a Course object to store this data. Then you will print a summary of the course. Then, modify st_enrolled to have 40 student (just use setStudents() method), and then print the summary once more. Your main function may start out: Course myclass; string dep, c_num; int sec, num_stus; ifstream fin("file.txt"); if(fin.fail()) { cout << "File path is bad\n"; exit(1); } fin >> dep >> c_num >> sec >> num_stus; fin.close(); myclass.setDepartment(dep); myclass.setNumber(c_num); //and continue Sample run: ----------------------------- Course: CSS 2A, Section: 1111 Enrolled: 35, Status: Open ----------------------------- Another sample run (notice since class is full, Status is set to closed): ----------------------------- Course: CSS 2A, Section: 1111 Enrolled: 40, Status: Closed -----------------------------
In C++
Create a new project named lab9_1 . You will need to implement a Course class. Here is its UML diagram:
Course |
- department : string - course_num : string - section : int - num_students : int - is_full : bool |
+ Course() + Course(string, string, int, int) + setDepartment(string) : void + setNumber(string) : void + setSection(int) : void + setStudents(int) : void + getDepartment() const : string + getNumber() const : string + getSection() const : int + getStudents() const : int + print() const : void |
Create a sample file to read from:
CSS 2A 1111 35
Additional information:
-
The Course class has two constructors. Make sure you have default values for your default constructor.
-
Each course maxes out at 40 students. Therefore, you need to make sure that there aren’t more than 40 students in a Course. You can choose how you handle situations where more than 40 students are added. Additionally, you should automatically set is_full to false or true, based on the number of students.
-
If you’d like to include additional methods than those found in the UML diagram, feel free to do so. That’s just the minimum amount required, but helper/utility methods would be okay.
-
You will also make use of the const keyword to indicate which methods are not modifying the object.
-
You will read in the information from the file, and declare a Course object to store this data. Then you will print a summary of the course. Then, modify st_enrolled to have 40 student (just use setStudents() method), and then print the summary once more.
Your main function may start out:
Course myclass;
string dep, c_num;
int sec, num_stus;
ifstream fin("file.txt");
if(fin.fail())
{
cout << "File path is bad\n";
exit(1);
}
fin >> dep >> c_num >> sec >> num_stus;
fin.close();
myclass.setDepartment(dep);
myclass.setNumber(c_num);
//and continue
Sample run:
-----------------------------
Course: CSS 2A, Section: 1111
Enrolled: 35, Status: Open
-----------------------------
Another sample run (notice since class is full, Status is set to closed):
-----------------------------
Course: CSS 2A, Section: 1111
Enrolled: 40, Status: Closed
-----------------------------

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

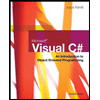
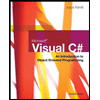