Implement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable • Constructor • Accessor and Update methods 2.) Define SLinkedList Class a. Instance Variables: # Node head # Node tail # int size b. Constructor c. Methods # int getSize() //Return the number of nodes of the list. # boolean isEmpty() //Return true if the list is empty, and false otherwise. # int getFirst() //Return the value of the first node of the list. # int getLast()/ /Return the value of the Last node of the list. # Node getHead()/ /Return the head # setHead(Node h)//Set the head # Node getTail()/ /Return the tail # setTail(Node t)//Set the tail # addFirst(E e) //add a new element to the front of the list # addLast(E e) // add new element to the end of the list # E removeFirst()//Return the value of the first node of the list # display()/ /print out values of all the nodes of the list # Node search(E key)//check if a given k is in the list, return found nodes or null # Node update(E key, E e)//update the value of a given k to new value, return updated node
Implement a Single linked list to store a set of Integer numbers (no duplicate)
• Instance variable
• Constructor
• Accessor and Update methods
2.) Define SLinkedList Class
a. Instance Variables:
# Node head
# Node tail
# int size
b. Constructor
c. Methods
# int getSize() //Return the number of nodes of the list.
# boolean isEmpty() //Return true if the list is empty, and false otherwise.
# int getFirst() //Return the value of the first node of the list.
# int getLast()/ /Return the value of the Last node of the list.
# Node getHead()/ /Return the head
# setHead(Node h)//Set the head
# Node getTail()/ /Return the tail
# setTail(Node t)//Set the tail
# addFirst(E e) //add a new element to the front of the list
# addLast(E e) // add new element to the end of the list
# E removeFirst()//Return the value of the first node of the list
# display()/ /print out values of all the nodes of the list
# Node search(E key)//check if a given k is in the list, return found nodes or null
# Node update(E key, E e)//update the value of a given k to new value, return updated node

Step by step
Solved in 4 steps with 1 images

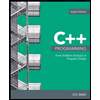
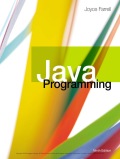
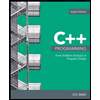
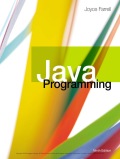