I am getting this error message. Below is my code in C++: #include #include using namespace std; int main() { int store; int totalPlayers = 5; char Menuoption; vector playerJerseyNumber; vector playerRating; for (int x = 1; x <= totalPlayers; x++) { cout << "\n"; cout << "Enter player " << x << "'s jersey number: "; cin >> store; if ((store >= 0) && (store <= 99)) { playerJerseyNumber.push_back(store); } cout << "Enter player " << x << "'s rating: "; cin >> store; if ((store >= 0) && (store <= 9)) { playerRating.push_back(store); } } cout << "\n"; cout << "ROSTER"; cout << "\n"; for (int x = 0; x < totalPlayers; x++) cout << "\nPlayer " << (x + 1) << " -- " << "Jersey number: " << playerJerseyNumber.at(x) << ", playerRating: " << playerRating.at(x)<< "\n"; while (true) { cout << "\nMENU"; cout << "\n"; cout << "a - Add player\n"; cout << "d - Remove player\n"; cout << "u - Update player rating\n"; cout << "r - Output players above a rating\n"; cout << "o - Output roster\n"; cout << "q - Quit\n"; cout << "Choose an option: "; cin >> Menuoption; switch (Menuoption) { case 'a': cout << "Enter another player's jersey number: "; cin >> store; if ((store >= 0) && (store <= 99)) { playerJerseyNumber.push_back(store); } cout << "Enter another player's playerRating: "; cin >> store; if ((store >= 0) && (store <= 9)) { playerRating.push_back(store); } break; case 'd': cout << "Enter a jersey number: "; cin >> store; for (int x = 0; x < playerJerseyNumber.size(); x++) { if (playerJerseyNumber.at(x) == store) { playerJerseyNumber.erase(playerJerseyNumber.begin() + x); playerRating.erase(playerRating.begin() + x); break; } } break; case 'u': cout << "Enter a jersey number: "; cin >> store; for (int x = 0; x < playerJerseyNumber.size(); x++) { if (playerJerseyNumber.at(x) == store) { cout << "Enter a new playerRating " << "for player: "; cin >> store; playerRating.at(x) = store; break; } } break; case 'r': cout << "Enter a rating: "; cin >> store; cout << "\n"; cout << "ABOVE" << store; for (int x = 0; x < playerJerseyNumber.size(); x++) { if (playerRating.at(x) > store) { cout << "\n"; cout << "\nPlayer " << (x + 1) << " -- " << "Jersey number: " << playerJerseyNumber.at(x) << ", rating: " << playerRating.at(x) << "\n"; } } break; case 'o': cout << "\nROSTER"; for (int x = 0; x < playerJerseyNumber.size(); x++) { cout << "\nPlayer " << (x + 1) << " -- " << "Jersey number: " << playerJerseyNumber.at(x) << ", rating: " << playerRating.at(x) << "\n"; } break; case 'q': return 0; } } }
I am getting this error message. Below is my code in C++:
#include <iostream>
#include <
using namespace std;
int main() {
int store;
int totalPlayers = 5;
char Menuoption;
vector<int> playerJerseyNumber;
vector<int> playerRating;
for (int x = 1; x <= totalPlayers; x++)
{
cout << "\n";
cout << "Enter player " << x << "'s jersey number: ";
cin >> store;
if ((store >= 0) && (store <= 99))
{
playerJerseyNumber.push_back(store);
}
cout << "Enter player " << x << "'s rating: ";
cin >> store;
if ((store >= 0) && (store <= 9))
{
playerRating.push_back(store);
}
}
cout << "\n";
cout << "ROSTER";
cout << "\n";
for (int x = 0; x < totalPlayers; x++)
cout << "\nPlayer " << (x + 1) << " -- " << "Jersey number: " << playerJerseyNumber.at(x) << ", playerRating: " << playerRating.at(x)<< "\n";
while (true)
{
cout << "\nMENU";
cout << "\n";
cout << "a - Add player\n";
cout << "d - Remove player\n";
cout << "u - Update player rating\n";
cout << "r - Output players above a rating\n";
cout << "o - Output roster\n";
cout << "q - Quit\n";
cout << "Choose an option: ";
cin >> Menuoption;
switch (Menuoption)
{
case 'a':
cout << "Enter another player's jersey number: ";
cin >> store;
if ((store >= 0) && (store <= 99))
{
playerJerseyNumber.push_back(store);
}
cout << "Enter another player's playerRating: ";
cin >> store;
if ((store >= 0) && (store <= 9))
{
playerRating.push_back(store);
}
break;
case 'd':
cout << "Enter a jersey number: ";
cin >> store;
for (int x = 0; x < playerJerseyNumber.size(); x++)
{
if (playerJerseyNumber.at(x) == store)
{
playerJerseyNumber.erase(playerJerseyNumber.begin() + x);
playerRating.erase(playerRating.begin() + x);
break;
}
} break;
case 'u':
cout << "Enter a jersey number: ";
cin >> store;
for (int x = 0; x < playerJerseyNumber.size(); x++)
{
if (playerJerseyNumber.at(x) == store)
{
cout << "Enter a new playerRating " << "for player: ";
cin >> store;
playerRating.at(x) = store;
break;
}
} break;
case 'r':
cout << "Enter a rating: ";
cin >> store;
cout << "\n";
cout << "ABOVE" << store;
for (int x = 0; x < playerJerseyNumber.size(); x++)
{
if (playerRating.at(x) > store)
{
cout << "\n";
cout << "\nPlayer " << (x + 1) << " -- " << "Jersey number: " << playerJerseyNumber.at(x)
<< ", rating: " << playerRating.at(x) << "\n";
}
}
break;
case 'o':
cout << "\nROSTER";
for (int x = 0; x < playerJerseyNumber.size(); x++)
{
cout << "\nPlayer " << (x + 1) << " -- " << "Jersey number: " << playerJerseyNumber.at(x)
<< ", rating: " << playerRating.at(x) << "\n";
}
break;
case 'q':
return 0;
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

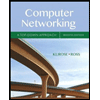
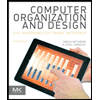
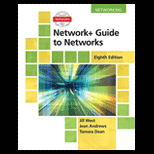
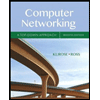
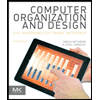
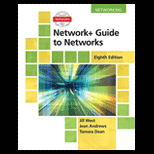
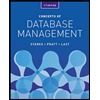
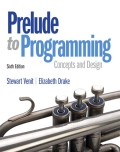
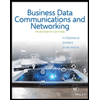