Homework Assignment-1 Topics: Functions, Dictionary, Import Problem description: Write a python application to simulate an online clothing system. The purpose of this assignment is to gain experience in Python dictionary structure, create basic Python functions and import a module. Design solution: The template file contains a list of clothes item sold, each item in the list contains the item id, name of the item and the prices. The program will generate an items_dict where each key is the item id of the cloth and the values will be a list of item name and price information. A customer will be presented with a main menu with 4 different options: display all items, add an item to cart, checkout and exit the program. A customer can buy an item by entering the ID of the item. This program implements a dictionary called cart, where each key is the item id and the values are the list of name, price and quantity that user chooses to purchase. The program keeps repeating by displaying the main menu to user until user hits the quit button. If user hits the checkout option, the program will display all the items in the cart and display the total amount purchased. Implementation: 1. This program will require to use two .py files: utility and application files. utility file contains all the helper functions. The application file contains the main functionality of the program. One helpful example can be: how to import a module. 2. This program requires to implement two dictionaries: items_dict and cart. items_dict will be populated from the given list of clothes at the beginning of the program. Each key will be the item id and the value will be a list of item name and price. cart dictionary will be a subset of items_dict that contains all the items user wants to purchase. The structure will be same as items_dict except the value list will contain name, price and quantity of the item. 3. The application file will contain a main function. main function implements the user menu and calls all the functions from the utility file. Hint: How to import a function from one file to another? 4. Utility file will contain the following function definitions. All these function call will be made from application file. a. build_dict(items): Returns items_dict from items list. items list is provided in the template file. This function is defined in the utility file and will be called from the application file at the beginning of the program. b. checkout(cart): Returns the total amount purchased using the user cart. This function will be called when user chooses to checkout for option 3. cart contains all the items user is purchasing. checkout function will get the price and quantity of an item from the cart dictionary and returns the total amount by multiplying price with quantity. Hint: price of an item is the -2 index and quantity at -1 index from the cart’s value list. c. display_all_items(d): Takes any dictionary d and display all the items in the dictionary. This function will be called for menu option 1 and option 3. Hint: How to print a dictionary? d. add_to_cart(item_id, cart, items_dict): Add an item to cart from the items_dict if item id exists in the items_dict. For menu option 2, user will provide the item id. If id exists then item will be added to the cart. Upon successful addition the function will return True otherwise returns False.
Homework Assignment-1
Topics: Functions, Dictionary, Import
Problem description: Write a python application to simulate an online clothing system. The
purpose of this assignment is to gain experience in Python dictionary structure, create basic
Python functions and import a module.
Design solution: The template file contains a list of clothes item sold, each item in the list
contains the item id, name of the item and the prices. The
where each key is the item id of the cloth and the values will be a list of item name and price
information. A customer will be presented with a main menu with 4 different options: display
all items, add an item to cart, checkout and exit the program. A customer can buy an item by
entering the ID of the item. This program implements a dictionary called cart, where each key is
the item id and the values are the list of name, price and quantity that user chooses to
purchase. The program keeps repeating by displaying the main menu to user until user hits the
quit button. If user hits the checkout option, the program will display all the items in the cart
and display the total amount purchased.
Implementation:
1. This program will require to use two .py files: utility and application files. utility file
contains all the helper functions. The application file contains the main functionality of
the program. One helpful example can be: how to import a module.
2. This program requires to implement two dictionaries: items_dict and cart. items_dict
will be populated from the given list of clothes at the beginning of the program. Each
key will be the item id and the value will be a list of item name and price. cart dictionary
will be a subset of items_dict that contains all the items user wants to purchase. The
structure will be same as items_dict except the value list will contain name, price and
quantity of the item.
3. The application file will contain a main function. main function implements the user
menu and calls all the functions from the utility file. Hint: How to import a function from
one file to another?
4. Utility file will contain the following function definitions. All these function call will be
made from application file.
a. build_dict(items): Returns items_dict from items list. items list is provided in the
template file. This function is defined in the utility file and will be called from the
application file at the beginning of the program.
b. checkout(cart): Returns the total amount purchased using the user cart. This
function will be called when user chooses to checkout for option 3. cart contains
all the items user is purchasing. checkout function will get the price and quantity
of an item from the cart dictionary and returns the total amount by multiplying
price with quantity. Hint: price of an item is the -2 index and quantity at -1 index
from the cart’s value list.
c. display_all_items(d): Takes any dictionary d and display all the items in the
dictionary. This function will be called for menu option 1 and option 3. Hint: How
to print a dictionary?
d. add_to_cart(item_id, cart, items_dict): Add an item to cart from the items_dict
if item id exists in the items_dict. For menu option 2, user will provide the item
id. If id exists then item will be added to the cart. Upon successful addition the
function will return True otherwise returns False.



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

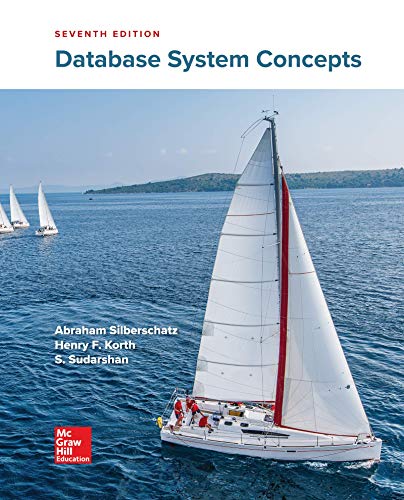
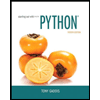
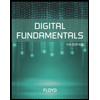
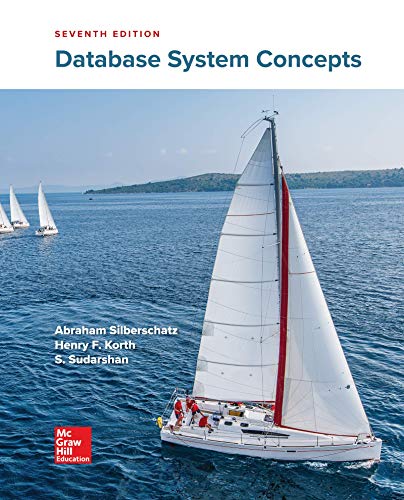
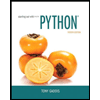
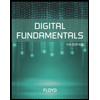
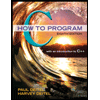
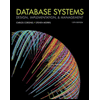
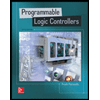