Given the menu with the following options as mentioned above, the call to print_main_menu(main_menu) will output: ======= ====== What would you like to do? L - List A - Add U - Update D Delete S Save the data R Restore data from file Q- Quit this program ========================== Program flow The expected program flow is: The main program starts with a menu of options given above Loop indefinitely (while the user didn't choose to exit): o Print the menu to the user o Get the user's choice from input() o Check if the user's choice is a valid option in the menu (is it one of the dictionary keys?). ■ If the input is a valid option, print the option that user selected ■ If not, simply continue from the top of the loop o If the user entered 'Q', break the while loop Instructions 1. Fix TODO 1: Add the options from the instructions to the menu dictionary inside the main program. 2. Fix TODO 2: Implement the "Quit" option, breaking from the while loop if the user input is an uppercase OR lowercase "Q". 3. Fix TODO 3: Check whether a provided option is a valid menu option. Each time a valid menu option is provided, the program "echoes" it back to the user as follows: print (f"You selected option (opt) to > {the_menu [opt]}.") Hints • Make sure you do not hard-code the menu options in your functions - the options need to be retrieved from the dictionary provided as
def print_main_menu(menu):
"""
Given a dictionary with the menu,
prints the keys and values as the
formatted options.
Adds additional prints for decoration
and outputs a question
"What would you like to do?"
"""
if __name__ == "__main__":
the_menu = {} # TODO 1: add the options from the instructions
opt = None
while True:
# print_main_menu(...) # TODO 1: uncomment, define the function, and call with the menu as an argument
print("::: Enter an option")
opt = input("> ")
if opt == ...: # TODO 2: make Q or q quit the program
print("Goodbye!\n")
break # exit the main `while` loop
else:
if ...: # TODO 3: check of the character stored in opt is in the_menu dictionary
print(f"You selected option {opt} to > {the_menu[opt]}.")
else:
print(f"WARNING: {opt} is an invalid option.\n")

![Given the menu with the following options as mentioned above, the call to print_main_menu(main_menu) will output:
====
====
What would you like to do?
List
A - Add
U - Update
D
Delete
S Save the data
R Restore data from file
Q- Quit this program
=====
Program flow
==============
The expected program flow is:
• The main program starts with a menu of options given above
• Loop indefinitely (while the user didn't choose to exit):
o Print the menu to the user
o Get the user's choice from input()
o
Check if the user's choice is a valid option in the menu (is it one of the dictionary keys?).
■ If the input is a valid option, print the option that user selected
■ If not, simply continue from the top of the loop
o If the user entered 'Q', break the while loop
Instructions
1. Fix TODO 1: Add the options from the instructions to the menu dictionary inside the main program.
2. Fix TODO 2: Implement the "Quit" option, breaking from the while loop if the user input is an uppercase OR lowercase "Q".
3. Fix TODO 3: Check whether a provided option is a valid menu option.
Each time a valid menu option is provided, the program "echoes" it back to the user as follows:
print (f"You selected option {opt} to > {the_menu [opt]}.")
Hints
• Make sure you do not hard-code the menu options in your functions - the options need to be retrieved from the dictionary provided as
a parameter to the function.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F979d0aba-5428-414f-a3ba-5510f0301082%2Fed69479b-12b9-482d-94f2-a7964fad9013%2Fa4jfbe_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

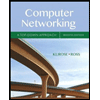
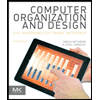
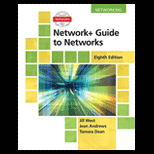
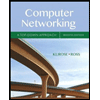
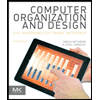
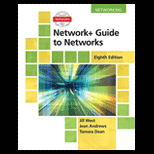
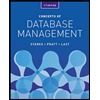
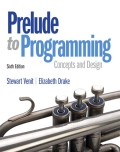
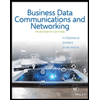