First, you need to design, code in Java, test and document a base class, Student. The Student class will have the following information, and all of these should be defined as Private: A. A first name (given name) B. A last name (family name/surname) C. Student number (ID) – an integer number (of type long) D. A date of birth (in day/month/year format – three integers) - (Create your own Date class, the Date class should be a separate class from this. Do NOT use the Date class from JAVA.) The Student class will have at least the following constructors and methods: (i) two constructors - one without any parameters (the default constructor), and one with parameters to give initial values to all the instance variables of Student. (ii) only necessary set and get methods for a valid class design. (iii) a reportGrade method, which you have nothing to report here, you can just print to the screen a message “There is no grade here.”. This method will be overridden in the respective child classes. (iv) an equals method which compares two student objects and returns true if they have the same student number (ID), otherwise it returns false. You may add other methods in the Student class as you see appropriate, however you will need to justify why those methods are required. Design, code in Java, test and document (at least) the following classes – • a UndergraduateStudent class, a GraduateStudent class (both derived from the Student class) • a UndergraduateUnit class, GraduateUnit class (both derived from the Unit class specified below) • and a Client class. Assuming in this program, you allow multiple student objects to be created (i.e. arraylist of student objects). For undergraduate students (UndergraduateStudent class): (a) Contain information of the UndergraduateUnit object (assuming that there is only one unit) (b) Provide a reportGrade method such that it will output “U” (to identify as undergraduate student), the Name (first name and last name), Student number, the unit ID, the overall mark, and the final grade of the student. For graduate students (GraduateStudent class): (a) Contain information of the GraduateUnit object (assuming that there is only one unit) (b) Provide a reportGrade method such that it will output “G” (to identify as graduate student), the Name (first name and last name), Student number, enrolment type and the final grade of the student. Unit class: (a) Enrolment type: “U” for undergraduate and “G” for graduate (b) A final grade reporting method for reporting the “NA” for not available. Undergraduate unit (UndergraduateUnit class): (a) Will have the information of the unit ID (of type string; e.g. ICT167), and the level of the unit (of type integer; e.g. 1 for first year) (b) There are two assignments, each marked out of a maximum of 100 marks and equally weighted. The marks for each assignment are recorded separately (c) There is weekly practical work. The marks for this component are recorded as a total mark obtained (marked out of a maximum of 20 marks) for all practical work demonstrated during the semester. (d) There is one final examination that is marked out of a maximum of 100 marks and recorded separately. (e) An overall mark (to be calculated within the class) (f) A final grade, which is a string (avoid code duplication for calculating this) The final grade, for undergraduate students, is to be awarded on the basis of an overall mark, which is a number in the range 0 to 100 and is obtained by calculating the weighted average of the student's performance in the assessment components. The criteria for calculating the weighted average is as defined below: The two assignments together count for a total of 50% (25% each) of the final grade, the practical work is worth 20%, and the final exam is worth 30% of the final grade. A grade is to be awarded for undergraduate students as follows: An overall mark of 80 or higher is an HD, an overall mark of 70 or higher (but less than 80) is a D, an overall mark of 60 or higher (but less than 70) is a C, an overall mark of 50 or higher (but less than 60) is a P, and an overall mark below 50 is an N. Graduate unit (GraduateUnit class): (a) Enrolment type of the graduate student; whether the student is a Master or PhD student (of type string; e.g. Master or PhD) (b) The number of years used to complete the thesis (of type integer, e.g. 3 for taking three years to complete). (c) A final grade, which is a character (C for completed; N for not completed; S for suspended)
First, you need to design, code in Java, test and document a base class, Student. The Student class will
have the following information, and all of these should be defined as Private:
A. A first name (given name)
B. A last name (family name/surname)
C. Student number (ID) – an integer number (of type long)
D. A date of birth (in day/month/year format – three integers) - (Create your own Date class, the
Date class should be a separate class from this. Do NOT use the Date class from JAVA.)
The Student class will have at least the following constructors and methods:
(i) two constructors - one without any parameters (the default constructor), and one with
parameters to give initial values to all the instance variables of Student.
(ii) only necessary set and get methods for a valid class design.
(iii) a reportGrade method, which you have nothing to report here, you can just print to the screen
a message “There is no grade here.”. This method will be overridden in the respective child
classes.
(iv) an equals method which compares two student objects and returns true if they have the same
student number (ID), otherwise it returns false.
You may add other methods in the Student class as you see appropriate, however you will need to
justify why those methods are required.
Design, code in Java, test and document (at least) the following classes –
• a UndergraduateStudent class, a GraduateStudent class (both derived from the Student class)
• a UndergraduateUnit class, GraduateUnit class (both derived from the Unit class specified
below)
• and a Client class.
Assuming in this program, you allow multiple student objects to be created (i.e. arraylist of student
objects).
For undergraduate students (UndergraduateStudent class):
(a) Contain information of the UndergraduateUnit object (assuming that there is only one unit)
(b) Provide a reportGrade method such that it will output “U” (to identify as undergraduate student),
the Name (first name and last name), Student number, the unit ID, the overall mark, and the final
grade of the student.
For graduate students (GraduateStudent class):
(a) Contain information of the GraduateUnit object (assuming that there is only one unit)
(b) Provide a reportGrade method such that it will output “G” (to identify as graduate student), the
Name (first name and last name), Student number, enrolment type and the final grade of the
student.
Unit class:
(a) Enrolment type: “U” for undergraduate and “G” for graduate
(b) A final grade reporting method for reporting the “NA” for not available.
Undergraduate unit (UndergraduateUnit class):
(a) Will have the information of the unit ID (of type string; e.g. ICT167), and the level of the unit (of
type integer; e.g. 1 for first year)
(b) There are two assignments, each marked out of a maximum of 100 marks and equally weighted.
The marks for each assignment are recorded separately
(c) There is weekly practical work. The marks for this component are recorded as a total mark
obtained (marked out of a maximum of 20 marks) for all practical work demonstrated during the
semester.
(d) There is one final examination that is marked out of a maximum of 100 marks and recorded
separately.
(e) An overall mark (to be calculated within the class)
(f) A final grade, which is a string (avoid code duplication for calculating this)
The final grade, for undergraduate students, is to be awarded on the basis of an overall mark, which is a
number in the range 0 to 100 and is obtained by calculating the weighted average of the student's
performance in the assessment components. The criteria for calculating the weighted average is as
defined below:
The two assignments together count for a total of 50% (25% each) of the final grade, the
practical work is worth 20%, and the final exam is worth 30% of the final grade.
A grade is to be awarded for undergraduate students as follows: An overall mark of 80 or higher is an
HD, an overall mark of 70 or higher (but less than 80) is a D, an overall mark of 60 or higher (but less
than 70) is a C, an overall mark of 50 or higher (but less than 60) is a P, and an overall mark below 50
is an N.
Graduate unit (GraduateUnit class):
(a) Enrolment type of the graduate student; whether the student is a Master or PhD student (of type
string; e.g. Master or PhD)
(b) The number of years used to complete the thesis (of type integer, e.g. 3 for taking three years to
complete).
(c) A final grade, which is a character (C for completed; N for not completed; S for suspended)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

First, you need to design, code in Java, test and document a base class, Student. The Student class will
have the following information, and all of these should be defined as Private:
-
A first name (given name)
-
A last name (family name/surname)
-
Student number (ID) – an integer number (of type long)
The Student class will have at least the following constructors and methods:
-
(i) two constructors - one without any parameters (the default constructor), and one with
parameters to give initial values to all the instance variables of Student.
-
(ii) only necessary set and get methods for a valid class design.
-
(iii) a reportGrade method, which you have nothing to report here, you can just print to the screen
a message “There is no grade here.”. This method will be overridden in the respective child
classes.
-
(iv) an equals method which compares two student objects and returns true if they have the same
student number (ID), otherwise it returns false.
You may add other methods in the Student class as you see appropriate, however you will need to justify why those methods are required.
ICT167 Assignment 2 2 TJ 2023
Design, code in Java, test and document (at least) the following classes –
-
a Student_Course class, a Student_Research class (both derived from the Student class)
-
a Unit_Course class, Research class (both derived from the Unit class specified below)
-
and a Client class.
Assuming in this program, you allow multiple student objects to be created (i.e. arraylist of student objects).
For course work students (Student_Course class):
-
(a) Contain enrolment type.
-
(b) Provide a reportGrade method such that it will output “C” (to identify as course work student), the Name (first name and last name), student number, the unit ID, the overall mark, and the final grade of the student.
For research students (Student_Research class):
-
(a) Contain enrolment type.
-
(b) Provide a reportGrade method such that it will output “R” (to identify as research student), the Name (first name and last name), student number, the overall mark, and the final grade of the student.
Unit class:
(a) Enrolment type: “C” for course work enrolment and “R” for research enrolment. (b) A final grade reporting method for reporting the “NA” for not available.
Course work unit (Unit_Course class):
-
(a) Will have the information of the unit ID (of type string; e.g. ICT333), and the level of the unit (of type integer; e.g. 3 for third year)
-
(b) There are two assignments, each marked out of a maximum of 100 marks and equally weighted. The marks for each assignment are recorded separately.
-
(c) There is one final examination that is marked out of a maximum of 100 marks and recorded separately.
-
(d) An overall mark (to be calculated within the class)
-
(e) A final grade, which is a string (avoid code duplication for calculating this)
The final grade is to be awarded on the basis of an overall mark, which is a number in the range 0 to 100 and is obtained by calculating the weighted average of the student's performance in the assessment components. The criteria for calculating the weighted average is as defined below:
The two assignments together count for a total of 60% (30% each) of the final grade, and the final exam is worth 40% of the final grade.
A grade is to be awarded for the students as follows: An overall mark of 80 or higher is an HD, an overall mark of 70 or higher (but less than 80) is a D, an overall mark of 60 or higher (but less than 70) is a C, an overall mark of 50 or higher (but less than 60) is a P, and an overall mark below 50 is an N.
Research enrolment (Research class):
(a) Enrolment type as “R”
(b) There is a proposal, marked out of a maximum of 100 mark.
(c) There is a final dissertation, marked out of a maximum of 100 mark. (d) An overall mark (to be calculated within the class)
ICT167 Assignment 2 3 TJ 2023 (e) A final grade, which is a string (avoid code duplication for calculating this)
The final grade is to be awarded on the basis of an overall mark, which is a number in the range 0 to 100 and is obtained by calculating the weighted average of the student's performance in the assessment components. The criteria for calculating the weighted average is as defined below:
The proposal is worth 40% of the final grade, and the final dissertation is worth 60% of the final grade.
A grade is to be awarded for the students as follows: An overall mark of 80 or higher is an HD, an overall mark of 70 or higher (but less than 80) is a D, an overall mark of 60 or higher (but less than 70) is a C, an overall mark of 50 or higher (but less than 60) is a P, and an overall mark below 50 is an N.
Client class:
The client program will allow entry of these data for several different student into an ArrayList and
then perform some analysis and queries.
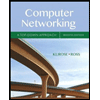
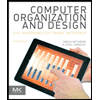
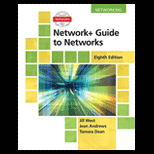
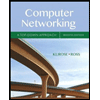
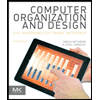
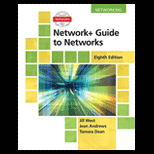
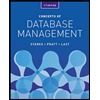
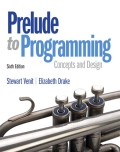
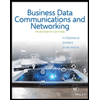