Finding the Minimum of a Vector Write a function to return the index of the minimum value in a vector, or -1 if the vector doesn't have any elements. In this problem you will implement the IndexOfMinimumElement function in minimum.cc and then call that function from main.cc. You do not need to edit minimum.h. Index of minimum value with std::vector Complete IndexOfMinimumElement in minimum.cc. This function takes a std::vector containing doubles as input and should return the index of the smallest value in the vector, or -1 if there are no elements. Complete main.cc Your main function asks the user how many elements they want, construct a vector, then prompts the user for each element and fills in the vector with values. Your only task in main is to call the IndexOfMinimumElement function declared in minimum.h, and print out the minimum element's index. Here's an example of how this might look: How many elements? 3 Element 0: 7 Element 1: -4 Element 2: 2 The minimum value in your vector is at index 1 main.cc file #include #include int main() { // First, we ask the user what size vector they want to create. int size = 0; std::cout << "How many elements? "; std::cin >> size; // Construct a vector of doubles with the given size. // This initializes all the elements in the vector to the // default value of double: 0. std::vector numbers(size); for (int i = 0; i < size; i++) { // In every iteration of this for loop, we prompt the user // to input the element at index i, then we put value // into the vector at index i. double value = 0; std::cout << "Element " << i << ": "; std::cin >> value; numbers.at(i) = value; } // ==================== YOUR CODE HERE ==================== // Call the IndexOfMinimumElement function and pass in // the `numbers` vector as input, and print out the index. // Don't forget to #include "minimum.h" so you can // make a call to the IndexOfMinimumElement declared there. // ======================================================== std::cout << "The minimum value in your vector is at index "; } minimum.cc file #include "minimum.h" int IndexOfMinimumElement(std::vector &input) { // ==================== YOUR CODE HERE ==================== // Find the index of the smallest element in the input // vector, and return it. If the input vector is empty, // return -1. // ======================================================== return -1; } minimum.h file #include // Returns the index of the smallest element in the vector, // or returns -1 if the vector is empty. int IndexOfMinimumElement(std::vector &input);
Finding the Minimum of a Vector
Write a function to return the index of the minimum value in a vector, or -1 if the vector doesn't have any elements. In this problem you will implement the IndexOfMinimumElement function in minimum.cc and then call that function from main.cc.
You do not need to edit minimum.h.
Index of minimum value with std::vector
Complete IndexOfMinimumElement in minimum.cc. This function takes a std::vector containing doubles as input and should return the index of the smallest value in the vector, or -1 if there are no elements.
Complete main.cc
Your main function asks the user how many elements they want, construct a vector, then prompts the user for each element and fills in the vector with values.
Your only task in main is to call the IndexOfMinimumElement function declared in minimum.h, and print out the minimum element's index. Here's an example of how this might look:
#include <iostream> | |
#include <vector> | |
int main() { | |
// First, we ask the user what size vector they want to create. | |
int size = 0; | |
std::cout << "How many elements? "; | |
std::cin >> size; | |
// Construct a vector of doubles with the given size. | |
// This initializes all the elements in the vector to the | |
// default value of double: 0. | |
std::vector<double> numbers(size); | |
for (int i = 0; i < size; i++) { | |
// In every iteration of this for loop, we prompt the user | |
// to input the element at index i, then we put value | |
// into the vector at index i. | |
double value = 0; | |
std::cout << "Element " << i << ": "; | |
std::cin >> value; | |
numbers.at(i) = value; | |
} | |
// ==================== YOUR CODE HERE ==================== | |
// Call the IndexOfMinimumElement function and pass in | |
// the `numbers` vector as input, and print out the index. | |
// Don't forget to #include "minimum.h" so you can | |
// make a call to the IndexOfMinimumElement declared there. | |
// ======================================================== | |
std::cout << "The minimum value in your vector is at index "; | |
} |
minimum.cc file
#include "minimum.h" | |
int IndexOfMinimumElement(std::vector<double> &input) { | |
// ==================== YOUR CODE HERE ==================== | |
// Find the index of the smallest element in the input | |
// vector, and return it. If the input vector is empty, | |
// return -1. | |
// ======================================================== | |
return -1; | |
} |
minimum.h file
#include <vector> | |
// Returns the index of the smallest element in the vector, | |
// or returns -1 if the vector is empty. | |
int IndexOfMinimumElement(std::vector<double> &input); |

Here's the updated code for minimum.cc:
----------------------------------------------------------------------------------------------------------------------------------
#include "minimum.h"
int IndexOfMinimumElement(std::vector<double> &input) {
if (input.empty()) {
return -1; // return -1 if the input vector is empty
}
int minIndex = 0;
double minValue = input[0];
for (int i = 1; i < input.size(); i++) {
if (input[i] < minValue) {
minValue = input[i];
minIndex = i;
}
}
return minIndex;
}
-----------------------------------------------------------------------------------------------------------------------------------
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

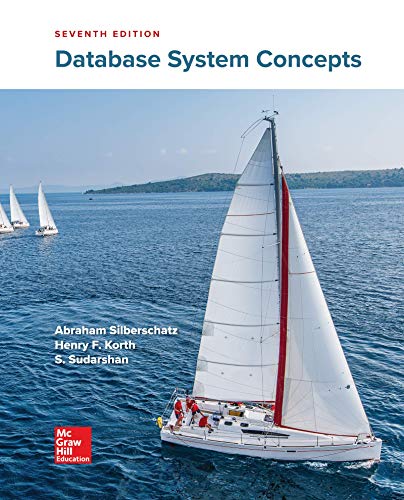
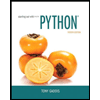
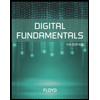
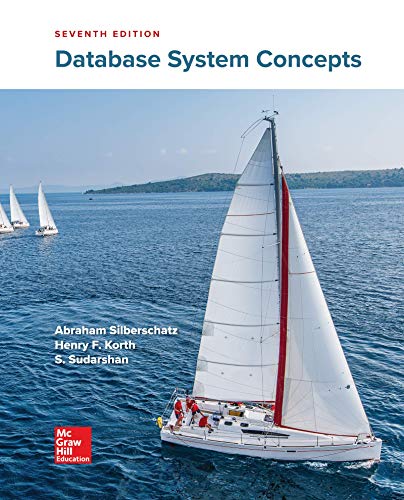
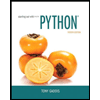
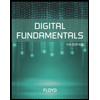
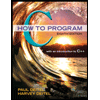
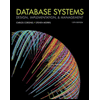
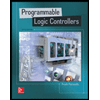