CREATE IN JAVA PLEASE 1. Create a class named Taxes. 2. Create two instance variables, one variable named taxDue and the other taxBracket. Both instance variables should be double. 3. Create a constructor that has an argument type double named tb. Initialize the taxDue to zero and the taxBracet to tb. 4. Create a method named calculateTaxDue. The method should not return anything and should have two arguments, both doubles. One argument should be totalIncome and the other should be taxPaid. 5. The calculateTaxDue should multiple the totalIncome by the taxBracket and assign it to a local variable named calcTax. 6. The calculateTaxD should subtract calcTax from taxPaid and assign it to taxDue. 7. Create a method to return taxDue. 1. Create a tester class. 2. Import Scanner. 3. Add the plumbing. 4. Create a scanner object. 5. Create an array to hold five doubles and name it income. 6. Create an array to hold five doubles and name it paid. 7. Create a for loop that will run from 0 to 5 (not including 5). Note there will be five items. 8. Ask the user to input for a double representing the tax the user received. 9. Assign the answer to an element in the income array. 10. Create a while loop that will run from 0 to 5 (not including 5). Note there will be five items. 11. Ask the user to input a double representing the tax paid. 12. Assign the answer to an element in the paid array. 13. Create a totalIncome double variable and assign it the value of 0. 14. Create a totalPaid double variable and assign it the value of 0. 15. Using an enhance for loop sum the elements in the array income and assign the result to a totalIncome variable. 16. Using an enhanced for loop, sum the elements in the array paid and assign the result to the totalPaid variable. 17. Create an object of type Taxes with an argument of .20. 18. Call the calculateTaxDue method with the arguments totalIncome and totalPaid. 19. Call the get method and print the results.
CREATE IN JAVA PLEASE
1. Create a class named Taxes.
2. Create two instance variables, one variable named taxDue and the other taxBracket. Both
instance variables should be double.
3. Create a constructor that has an argument type double named tb. Initialize the taxDue to
zero and the taxBracet to tb.
4. Create a method named calculateTaxDue. The method should not return anything and
should have two arguments, both doubles. One argument should be totalIncome and the
other should be taxPaid.
5. The calculateTaxDue should multiple the totalIncome by the taxBracket and assign it to a
local variable named calcTax.
6. The calculateTaxD should subtract calcTax from taxPaid and assign it to taxDue.
7. Create a method to return taxDue.
1. Create a tester class.
2. Import Scanner.
3. Add the plumbing.
4. Create a scanner object.
5. Create an array to hold five doubles and name it income.
6. Create an array to hold five doubles and name it paid.
7. Create a for loop that will run from 0 to 5 (not including 5). Note there will be five items.
8. Ask the user to input for a double representing the tax the user received.
9. Assign the answer to an element in the income array.
10. Create a while loop that will run from 0 to 5 (not including 5). Note there will be five items.
11. Ask the user to input a double representing the tax paid.
12. Assign the answer to an element in the paid array.
13. Create a totalIncome double variable and assign it the value of 0.
14. Create a totalPaid double variable and assign it the value of 0.
15. Using an enhance for loop sum the elements in the array income and assign the result to a
totalIncome variable.
16. Using an enhanced for loop, sum the elements in the array paid and assign the result to the
totalPaid variable.
17. Create an object of type Taxes with an argument of .20.
18. Call the calculateTaxDue method with the arguments totalIncome and totalPaid.
19. Call the get method and print the results.

Step by step
Solved in 5 steps with 3 images

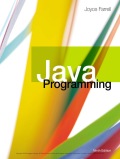
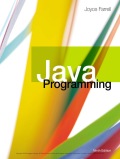