Create an abstract class named Book. Include a String field for the book’s title and a double field for the book’s price. Within the class, include a constructor that requires the book title, and add two get methods—one that returns the title and one that returns the price. Include an abstract method named setPrice(). Create two child classes of Book: Fiction and NonFiction. Each must include a setPrice() method that sets the price for all Fiction Books to $24.99 and for all NonFiction Books to $37.99. Write a constructor for each subclass, and include a call to setPrice() within each. Write an application demonstrating that you can create both a Fiction and a NonFiction Book, and display their fields. Code I was given - public abstract class Book { // attributes // constructor // get and set methods } public class BookArray { public static void main(String[] args) { // your code here } } public class Fiction { // constructor // setPrice() } public class NonFiction { // constructor // setPrice() } public class UseBook { public static void main(String[] args) { Fiction aNovel = new Fiction("Huckelberry Finn"); NonFiction aManual = new NonFiction("Elements of Style"); System.out.println("Fiction " + aNovel.getTitle() + " costs $" + aNovel.getPrice()); System.out.println("Non-Fiction " + aManual.getTitle() + " costs $" + aManual.getPrice()); } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
This is the question -
Create an abstract class named Book. Include a String field for the book’s title and a double field for the book’s price. Within the class, include a constructor that requires the book title, and add two get methods—one that returns the title and one that returns the price. Include an abstract method named setPrice().
Create two child classes of Book: Fiction and NonFiction. Each must include a setPrice() method that sets the price for all Fiction Books to $24.99 and for all NonFiction Books to $37.99. Write a constructor for each subclass, and include a call to setPrice() within each. Write an application demonstrating that you can create both a Fiction and a NonFiction Book, and display their fields.
Code I was given -

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

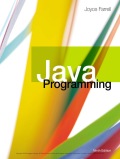
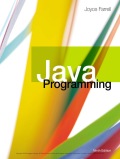