Create a Python class that represents a standard playing card. Playing cards (in America, at least) have rank and suit. The suits of a deck of playing cards are Hearts, Spades, Clubs, and Diamonds. Cards of each suit range in rank from Ace (1), 2, 3, 4, 5, 6, 7, 8, 9, 10, Jack (11), Queen (12) and King (13) Additionally, when playing Blackjack, the values of each card is usually the same as its rank except that face cards (Jack, King, Queen) have a value of 10, and the Ace has a value of 1 (“Aces low”). ***Implement a class representing a playing card, and name it PlayingCard. These class methods are required: 1. __init__(self, rank, suit): This is your class initializer. - self is required, of course. Make sure you understand its purpose in classes. - rank is an int value that maps to the rank of a playing card. This number ranges from 1-13 (Ace, 2, 3, …, King.) - suit is a single character "d", "c", "h", "s" respectifully representing each suit a card could possibly have (Diamonds, Clubs, Hearts, or Spades) 2. get_rank(self): - When called on an instance of your PlayingCard class, it will return the rank of that particular card as an integer from 1-13 (Ace, 2, 3, …, King.) 3. get_suit(self): - When called on an instance of your PlayingCard class, it will return the suit of that particular card as single-character string of either "d", "c", "h", "s", where the letters represent Diamonds, Clubs, Hearts, or Spades, respectfully. 4. bj_value(self): - When called on an instance of your PlayingCard class, it will return the Blackjack value of that particular card as an integer. 5. __repr__(self): - You will be introduced to another dunder name. This one, when defined inside your class, allows any instance of your class to properly describe itself when you attempt to, say, print an object representing an instance of your class PlayingCard. Basically, it’s a technique to convert a non-printing object into a string. 6. main(): - function that tests your PlayingCard class by creating n instances of randomly generated cards and then asking each instance of these cards to print their information and their Blackjack value. Note: You will print an instance of you PlayingCard object directly, making indirect (thus, proper) use of the __repr__ method!
Create a Python class that represents a standard playing card.
Playing cards (in America, at least) have rank and suit.
- The suits of a deck of playing cards are Hearts, Spades, Clubs, and Diamonds.
- Cards of each suit range in rank from Ace (1), 2, 3, 4, 5, 6, 7, 8, 9, 10, Jack (11), Queen (12) and King (13)
Additionally, when playing Blackjack, the values of each card is usually the same as its rank except that face cards (Jack, King, Queen) have a value of 10, and the Ace has a value of 1 (“Aces low”).
***Implement a class representing a playing card, and name it PlayingCard. These class methods are required:
1. __init__(self, rank, suit): This is your class initializer.
- self is required, of course. Make sure you understand its purpose in classes.
- rank is an int value that maps to the rank of a playing card. This number ranges from 1-13 (Ace, 2, 3, …, King.)
- suit is a single character "d", "c", "h", "s" respectifully representing each suit a card could possibly have (Diamonds, Clubs, Hearts, or Spades)
2. get_rank(self):
- When called on an instance of your PlayingCard class, it will return the rank of that particular card as an integer from 1-13 (Ace, 2, 3, …, King.)
3. get_suit(self):
- When called on an instance of your PlayingCard class, it will return the suit of that particular card as single-character string of either "d", "c", "h", "s", where the letters represent Diamonds, Clubs, Hearts, or Spades, respectfully.
4. bj_value(self):
- When called on an instance of your PlayingCard class, it will return the Blackjack value of that particular card as an integer.
5. __repr__(self):
- You will be introduced to another dunder name. This
one, when defined inside your class, allows any instance of your class to properly describe itself when you attempt to, say, print an object representing an instance of your class PlayingCard. Basically, it’s a technique to convert a non-printing object into a string.
6. main():
- function that tests your PlayingCard class by creating n instances of randomly generated cards and then asking each instance of these cards to print their information and their Blackjack value.
Note: You will print an instance of you PlayingCard object directly, making indirect (thus, proper) use of the __repr__ method!

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

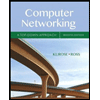
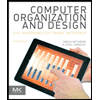
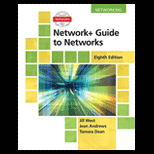
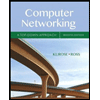
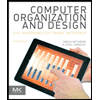
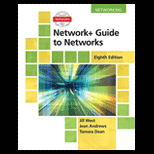
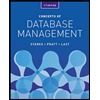
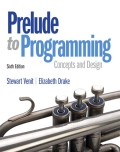
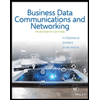