Create a DeleteDuplicatesclass which consists of a deleteDuplicates method which takes the nums list as its parameter. Implement the deleteDuplicates method: Check if the input list is null or empty, and return an empty list if so. Initialize a pointer i to keep track of the unique elements. Use a for loop to Iterate through the list using another pointer j which starts at j=1 and goes until j=nums.size(); If the current element at j is not equal to the previous element at i using !nums.get(i).equals(nums.get(j), increment i and set the current element at j to the new position at i using nums.set(i, nums.get(j)). After the for loop ends, return the sublist from the beginning to i + 1, as this will contain all unique elements. Call the deleteDuplicates method with the sample list as argument: Call the deleteDuplicates method and pass the nums list as argument Store the result in a variable result Print the result to the console. Input: [1, 1, 2, 3, 3, 3, 4, 5, 5] Output: [1, 2, 3, 4, 5] ======================================================================================= import java.util.ArrayList; import java.util.List; public class DeleteDuplicates { /* * This method deletes duplicates from a sorted ArrayList. * Nums is the sorted ArrayList with duplicates. * Return the sorted ArrayList without duplicates */ public static List deleteDuplicates(List nums) { // Check if the list is null or empty, and return an empty list if so if () { } // Initialize a pointer 'i' to keep track of the unique elements int i = 0; // Iterate through the list using another pointer 'j' for () { // If the current element at 'j' is not equal to the previous element at 'i', // increment 'i' and set the current element at 'j' to the new position at 'i' if () { } } // Return the sublist from the beginning to 'i + 1', as this will contain all unique elements return nums.subList(0, i + 1); } public static void main(String[] args) { // Initialize a sample sorted ArrayList with duplicate values List nums = new ArrayList<>(); nums.add(1); nums.add(1); nums.add(2); nums.add(3); nums.add(3); nums.add(3); nums.add(4); nums.add(5); nums.add(5); // Call the deleteDuplicates method with the sample list as argument List result = deleteDuplicates(nums); // Print the result to the console System.out.println(result); } }
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
-
Create a DeleteDuplicatesclass which consists of a deleteDuplicates method which takes the nums list as its parameter.
-
Implement the deleteDuplicates method:
-
Check if the input list is null or empty, and return an empty list if so.
-
Initialize a pointer i to keep track of the unique elements.
-
Use a for loop to Iterate through the list using another pointer j which starts at j=1 and goes until j=nums.size();
-
If the current element at j is not equal to the previous element at i using !nums.get(i).equals(nums.get(j), increment i and set the current element at j to the new position at i using nums.set(i, nums.get(j)).
-
After the for loop ends, return the sublist from the beginning to i + 1, as this will contain all unique elements.
-
-
Call the deleteDuplicates method with the sample list as argument:
-
Call the deleteDuplicates method and pass the nums list as argument
-
Store the result in a variable result
-
-
Print the result to the console.
Input: [1, 1, 2, 3, 3, 3, 4, 5, 5]
Output: [1, 2, 3, 4, 5]
=======================================================================================

Step by step
Solved in 4 steps with 2 images

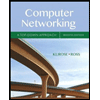
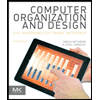
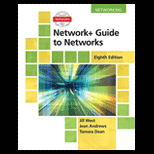
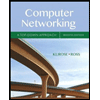
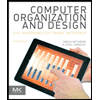
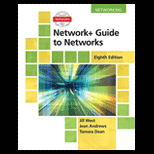
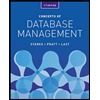
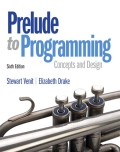
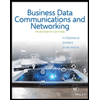