Code using c++ 4. Bored Person by CodeChum Admin Help! Our people in this tech universe we created are starting to get really, really boreed! They're complaining because there's internet connection but they don't have mobile phones. We need to create one for them ASAP or else they will go crazy!! Instructions: In the code editor, you are provided with the definition of a struct Person. This struct needs an integer value for its age, character value for its gender, and a struct Phone for its phone. Furthermore, you are provided with a displayPerson() function which accepts a struct Person as its parameter. Your task is to define a new struct called Phone. This Phone would need 3 properties: char type - which can either be 'I' for iPhone or 'A' for Android int ram - the number of RAM this phone has int storage - the number of storage this phone has Then, using this struct Phone you defined, create a Phone in the main() and ask the user for its type, ram, and storage. Next, create a struct Person and ask the user for its age and gender and then set its phone to the Phone you just created earlier. Finally, call the displayPerson() function and pass the Person. Input 1. The type of the Phone 2. The RAM of the Phone 3. The storage of the Phone 4. The age of the Person 5. The gender of the Person Output Enter·Phone's·type:·I Enter·Phone's·RAM:·2 Enter·Phone's·storage:·124 Enter·Person's·age:·24 Enter·Person's·gender:·M PERSON·DETAILS: Age:·24 Gender:·Male Phone:·iPhone·(2GB,·124GB)
Code using c++ 4. Bored Person by CodeChum Admin Help! Our people in this tech universe we created are starting to get really, really boreed! They're complaining because there's internet connection but they don't have mobile phones. We need to create one for them ASAP or else they will go crazy!! Instructions: In the code editor, you are provided with the definition of a struct Person. This struct needs an integer value for its age, character value for its gender, and a struct Phone for its phone. Furthermore, you are provided with a displayPerson() function which accepts a struct Person as its parameter. Your task is to define a new struct called Phone. This Phone would need 3 properties: char type - which can either be 'I' for iPhone or 'A' for Android int ram - the number of RAM this phone has int storage - the number of storage this phone has Then, using this struct Phone you defined, create a Phone in the main() and ask the user for its type, ram, and storage. Next, create a struct Person and ask the user for its age and gender and then set its phone to the Phone you just created earlier. Finally, call the displayPerson() function and pass the Person. Input 1. The type of the Phone 2. The RAM of the Phone 3. The storage of the Phone 4. The age of the Person 5. The gender of the Person Output Enter·Phone's·type:·I Enter·Phone's·RAM:·2 Enter·Phone's·storage:·124 Enter·Person's·age:·24 Enter·Person's·gender:·M PERSON·DETAILS: Age:·24 Gender:·Male Phone:·iPhone·(2GB,·124GB)
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter12: Adding Functionality To Your Classes
Section12.2: Providing Class Conversion Capabilities
Problem 2E
Related questions
Question
Code using c++
4. Bored Person
by CodeChum Admin
Help! Our people in this tech universe we created are starting to get really, really boreed!
They're complaining because there's internet connection but they don't have mobile phones. We need to create one for them ASAP or else they will go crazy!!
Instructions:
- In the code editor, you are provided with the definition of a struct Person. This struct needs an integer value for its age, character value for its gender, and a struct Phone for its phone. Furthermore, you are provided with a displayPerson() function which accepts a struct Person as its parameter.
- Your task is to define a new struct called Phone. This Phone would need 3 properties:
- char type - which can either be 'I' for iPhone or 'A' for Android
- int ram - the number of RAM this phone has
- int storage - the number of storage this phone has
- Then, using this struct Phone you defined, create a Phone in the main() and ask the user for its type, ram, and storage.
- Next, create a struct Person and ask the user for its age and gender and then set its phone to the Phone you just created earlier.
- Finally, call the displayPerson() function and pass the Person.
Input
1. The type of the Phone
2. The RAM of the Phone
3. The storage of the Phone
4. The age of the Person
5. The gender of the Person
Output
Enter·Phone's·type:·I
Enter·Phone's·RAM:·2
Enter·Phone's·storage:·124
Enter·Person's·age:·24
Enter·Person's·gender:·M
PERSON·DETAILS:
Age:·24
Gender:·Male
Phone:·iPhone·(2GB,·124GB)

Transcribed Image Text:3. int storage - the number of storage this phone has
>
+
+ C++
main.cpp
3. Then, using this struct Phone you defined, create a Phone in the main() and ask the
1 #include <iostream>
user for its type, ram, and storage.
2 using namespace std;
4. Next, create a struct Person and ask the user for its age and gender and then set its
3
phone to the Phone you just created earlier.
5. Finally, call the displayPerson () function and pass the Person.
4 typedef struct {
int age;
char gender;
Phone phone;
6
7
Input
8 } Person;
1. The type of the Phone
10 void displayPerson(Person);
11
12 int main(void) {
13
2. The RAM of the Phone
3. The storage of the Phone
14
15
return 0;
4. The age of the Person
16 }
17
5. The gender of the Person
18 void displayPerson(Person p) {
cout <« "PERSON DETAILS:" « endl;
cout << "Age:
cout << "Gender: ";
if(p.gender == 'M') {
cout <« "Male";
} else {
cout <« "Female";
}
cout << endl;
19
Output
<« p.age << endl;
20
21
22
Enter Phone's type: I
Enter Phone's RAM: 2
23
24
Enter Phone's storage: 124
25
26
27
Enter Person's age: 24
28
Enter Person's gender: M
cout << "Phone: ";
if(p.phone.type == 'I') {
cout <« "iPhone";
} else {
cout <« "Android";
}
cout <« " (" « p.phone.ram << "GB, " < p.phone.storage <« "GB)";
29
30
PERSON DETAILS:
31
Age: 24
32
33
Gender: Male
34
Phone: iPhone (2GB, 124GB)
35
36 }
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
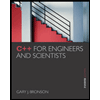
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
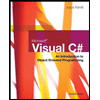
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
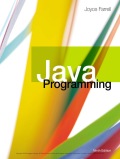
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
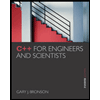
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
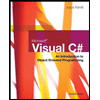
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
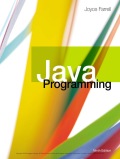
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT