clude // size should not be negative typedef unsigne
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 2CP
Related questions
Question
Try to do it asap give output screenshots
Complete the code, where it says "TO DO"
code:
#ifndef __SLLIST_H__
#define __SLLIST_H__
#include "Node.h"
#include <cassert>
// size should not be negative
typedef unsigned long size_t;
namespace ds {
template <typename ItemType> class TestDriver; // for autograding; please ignore
/** Singly linked list. */
template <typename ItemType>
class SLList {
friend class TestDriver<ItemType>; // for autograding; please ignore
private:
/** Pointer pointing to the sentinel node. */
Node<ItemType> *sentinel;
/** Stores the current size of the list. */
size_t count;
public:
/** Construct a new SLList object. */
SLList() {
sentinel = new Node<ItemType>(ItemType(), nullptr);
count = 0;
}
/** Add x at the beginning of the list. */
void addFirst(ItemType x) {
count += 1;
sentinel->next = new Node<ItemType>(x, sentinel->next);
}
/** Return the first element. */
ItemType &getFirst() const {
assert(sentinel->next != nullptr);
return sentinel->next->item;
}
/** Return the number of elements in list. */
size_t size() const { return count; }
/** Append the list with x. */
void addLast(ItemType x) {
count += 1;
Node<ItemType> *p = sentinel;
while (p->next != nullptr) {
p = p->next;
}
p->next = new Node<ItemType>(x, nullptr);
}
SLList(const SLList<ItemType> &other);
~SLList();
ItemType &get(int i) const;
ItemType removeFirst();
ItemType removeLast();
};
/** Copy constructor. */
template <typename ItemType>
SLList<ItemType>::SLList(const SLList<ItemType> &other) {
// TODO: create a list that is identical to `other`
}
/** Destroy the SLList object. */
template <typename ItemType>
SLList<ItemType>::~SLList() {
// TODO:
}
/** Return the i-th item in list. */
template <typename ItemType>
ItemType &SLList<ItemType>::get(int i) const {
// TODO:
}
/** Delete and return the first item of the list. */
template <typename ItemType>
ItemType SLList<ItemType>::removeFirst() {
// TODO:
}
/** Delete and return the last item of the list. */
template <typename ItemType>
ItemType SLList<ItemType>::removeLast() {
// TODO:
}
} // namespace ds
#endif // __SLLIST_H__
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
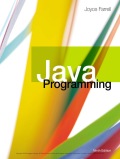
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
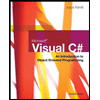
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
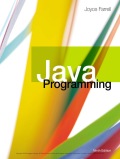
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
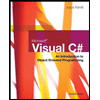
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,