C#: • Using System.Threading • Create a Baby class • It should have variables: time (int) and name (String). • Write a constructor which takes in a name o Set the name variable in the object with the name you were passed. o Create a Random object o Set the time variable to your Random Object's .Next(5000) Create a method Run() with no parameters o Print out “My name is X I am going to sleep for Yms" X should be the objects name, should be the time you randomly chose. o Call Thread.Sleep(time); o Print out "My name is X, I am awake, feed me!!!" o You will need to wrap the contents of your run method in a try/catch block, catching ThreadInterruptedException. • In your main class's main method, do the following: o Create 5 babies as follows: Baby b1 = new Baby(“Noah"); • Repeat this for b2, b3, b4, b5. They should be named Noah, Olivia, Liam, Emma, Amelia. o Create 5 threads as follows: Thread tl = new Thread(b1.Run); Repeat this for t2, t3, t4 and t5. o Start all 5 babies with a statement like “tl.Start();" Sample Output: My name is Noah and I am going to sleep for 3398ms My name is Olivia and I am going to sleep for 1687ms My name is Amelia and I am going to sleep for 448ms My name is Emma and I am going to sleep for 4792ms My name is Liam and I am going to sleep for 2671ms My name is Amelia and I'm awake,feed me!!! My name is Olivia and I'm awake,feed me!!! My name is Liam and I'm awake,feed me!!! My name is Noah and I'm awake, feed me!!! My name is Emma and I'm awake, feed me!!!
C#: • Using System.Threading • Create a Baby class • It should have variables: time (int) and name (String). • Write a constructor which takes in a name o Set the name variable in the object with the name you were passed. o Create a Random object o Set the time variable to your Random Object's .Next(5000) Create a method Run() with no parameters o Print out “My name is X I am going to sleep for Yms" X should be the objects name, should be the time you randomly chose. o Call Thread.Sleep(time); o Print out "My name is X, I am awake, feed me!!!" o You will need to wrap the contents of your run method in a try/catch block, catching ThreadInterruptedException. • In your main class's main method, do the following: o Create 5 babies as follows: Baby b1 = new Baby(“Noah"); • Repeat this for b2, b3, b4, b5. They should be named Noah, Olivia, Liam, Emma, Amelia. o Create 5 threads as follows: Thread tl = new Thread(b1.Run); Repeat this for t2, t3, t4 and t5. o Start all 5 babies with a statement like “tl.Start();" Sample Output: My name is Noah and I am going to sleep for 3398ms My name is Olivia and I am going to sleep for 1687ms My name is Amelia and I am going to sleep for 448ms My name is Emma and I am going to sleep for 4792ms My name is Liam and I am going to sleep for 2671ms My name is Amelia and I'm awake,feed me!!! My name is Olivia and I'm awake,feed me!!! My name is Liam and I'm awake,feed me!!! My name is Noah and I'm awake, feed me!!! My name is Emma and I'm awake, feed me!!!
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 14RQ
Related questions
Question

Transcribed Image Text:C#:
• Using System.Threading
• Create a Baby class
• It should have variables: time (int) and name (String).
Write a constructor which takes in a name
o Set the name variable in the object with the name you were passed.
o Create a Random object
o Set the time variable to your Random Object's .Next(5000)
• Create a method Run() with no parameters
o Print out “My name is X I am going to sleep for Yms" X should be the objects name,
should be the time you randomly chose.
o Call Thread.Sleep(time);
o Print out "My name is X, I am awake, feed me!!"
o You will need to wrap the contents of your run method in a try/catch block, catching
Y
ThreadInterruptedException.
In your main class's main method, do the following:
o Create 5 babies as follows:
· Baby bl = new Baby(“Noah");
Repeat this for b2, b3, b4, b5. They should be named Noah, Olivia, Liam, Emma,
Amelia.
o Create 5 threads as follows:
1 Thread tl = new Thread(b1.Run);
Repeat this for t2, t3, t4 and t5.
o Start all 5 babies with a statement like “tl.Start();"
Sample Output:
My name is Noah and I am going to sleep for 3398ms
My name is Olivia and I am going to sleep for 1687ms
My name is Amelia and I am going to sleep for 448ms
My name is Emma and I am going to sleep for 4792ms
My name is Liam and I am going to sleep for 2671ms
My name is Amelia and I'm awake,feed me!!!
My name is Olivia and I'm awake,feed me!!!
My name is Liam and I'm awake,feed me!!!
My name is Noah and I'm awake,feed me!!!
My name is Emma and I'm awake,feed me!!!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
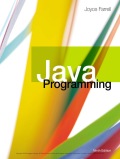
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
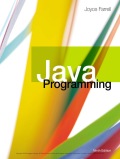
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT