As a starting point, use the files fraction.h, fraction.cpp, testfraction.cpp below: // simple class for fractions #ifndef FRACTION_H_ #define FRACTION_H_ class Fraction {
As a starting point, use the files fraction.h, fraction.cpp, testfraction.cpp below:
// simple class for fractions
#ifndef FRACTION_H_
#define FRACTION_H_
class Fraction {
private: // internal implementation is hidden
int num; // numerator
int den; // denominator
int gcd(int a, int b); // calculates the gcd of a and b
int lcm(int a, int b);
public:
Fraction(); // empty constructor
Fraction(int, int = 1); // constructor taking values for fractions and
// integers. Denominator by default is 1
void print(); // prints it to the screen
};
#endif /* FRACTION_H_ */
#include <iostream>
#include "fraction.h"
Fraction::Fraction()
{
num = 1;
den = 1;
}
Fraction::Fraction(int n, int d)
{
int tmp_gcd = gcd(n, d);
num = n / tmp_gcd;
den = d / tmp_gcd;
}
int Fraction::gcd(int a, int b)
{
int tmp_gcd = 1;
// Implement GCD of two numbers;
return tmp_gcd;
}
int Fraction::lcm(int a, int b)
{
return a * b / gcd(a, b);
}
void Fraction::print()
{
std::cout << num << "/" << den << std::endl;
}
#include <iostream>
#include "fraction.h"
using namespace std;
int main(void)
{
Fraction a(4, 2);
Fraction b(17, 11);
Fraction c(5);
a.print();
b.print();
c.print();
}


Step by step
Solved in 5 steps with 4 images

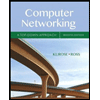
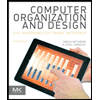
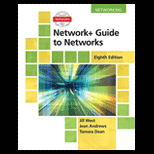
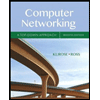
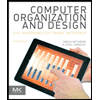
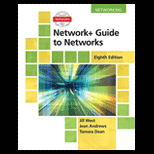
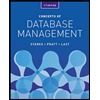
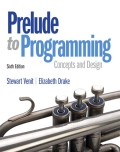
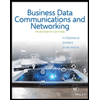