A Java program to demonstrate use of Comparable
**Students.java code**
// A Java program to demonstrate use of Comparable
import java.util.*;
// A class 'Student' that implements Comparable
class Student implements Comparable<Student>
{
private String FirstName;
private String LastName;
private int StudentID;
// Used to sort movies by year
public int compareTo(Student a)
{
return this.StudentID - a.StudentID;
}
// Constructor
public Student(String fm, String ls, int id)
{
this.FirstName = fm;
this.LastName = ls;
this.StudentID = id;
}
// Getter methods for accessing private data
public String getFirstName() { return FirstName; }
public String getLastName() { return LastName; }
public int getStudentID() { return StudentID; }
}
// Driver class
class Students
{
public static void main(String[] args)
{
ArrayList<Student> list = new ArrayList<Student>();
list.add(new Student("Amir", "Alao", 123456));
list.add(new Student("John", "Smith", 654321));
list.add(new Student("Mario", "Ellie", 198045));
list.add(new Student("James", "Harden", 198312));
list.add(new Student("LeBron", "James", 139284));
System.out.println("Student names before Sorting : ");
for (Student student: list)
{
System.out.println(student.getFirstName() + " " +
student.getLastName() + " " +
student.getStudentID());
}
System.out.println("*******************Sorting
Collections.sort(list);
System.out.println("Student names after Sorting : ");
for (Student student: list)
{
System.out.println(student.getFirstName() + " " +
student.getLastName() + " " +
student.getStudentID());
}
}
}
***ObjectMergeSorter Code***
import java.util.ArrayList;
import java.util.Collections;
public class ObjectMergeSorter {
// calls recursive sortArray method to begin merge sorting
// splits array, sorts subarrays and merges subarrays into sorted array
private static void sortArray(int[] Student, int low, int high) {
// test base case; size of array equals 1
if ((high - low) >= 1) { // if not base case
int middle1 = (low + high) / 2; // calculate middle of array
int middle2 = middle1 + 1; // calculate next element over
// split array in half; sort each half (recursive calls)
sortArray(Student, low, middle1); // first half of array
sortArray(Student, middle2, high); // second half of array
// merge two sorted arrays after split calls return
merge (Student, low, middle1, middle2, high);
}
}
// merge two sorted subarrays into one sorted subarray
private static void merge(int[] Student, int left, int middle1,
int middle2, int right) {
int leftIndex = left; // index into left subarray
int rightIndex = middle2; // index into right subarray
int combinedIndex = left; // index into temporary working array
int[] combined = new int[Student.length]; // working array
// merge arrays until reaching end of either
while (leftIndex <= middle1 && rightIndex <= right) {
// place smaller of two current elements into result
// and move to next space in arrays
if (Student[leftIndex] <= Student[rightIndex]) {
combined[combinedIndex++] = Student[leftIndex++];
}
else {
combined[combinedIndex++] = Student[rightIndex++];
}
}
// if left array is empty
if (leftIndex == middle2) {
// copy in rest of right array
while (rightIndex <= right) {
combined[combinedIndex++] = Student[rightIndex++];
}
}
else { // right array is empty
// copy in rest of left array
while (leftIndex <= middle1) {
combined[combinedIndex++] = Student[leftIndex++];
}
}
// copy values back into original array
for (int i = left; i <= right; i++) {
Student[i] = combined[i];
}
// output merged array
}
// Driver class
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

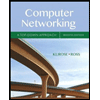
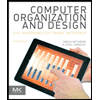
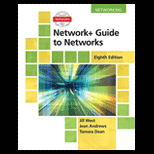
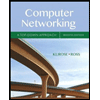
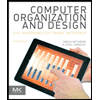
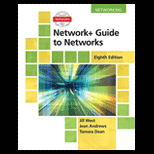
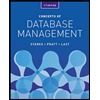
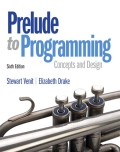
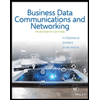