1. Write a program to fill in a two-dimensional array of boolean values by setting a[i][j] to 1 if the greatest common divisor of i and j is 1. Else if the greatest common divisor is not 1, then set a[i][j] to 0. Sample Input: ./a.out 6 Sample Output: 000000 011111 010101 011011 010101 1110
1. Write a program to fill in a two-dimensional array of boolean values by setting a[i][j] to 1 if the greatest common divisor of i and j is 1. Else if the greatest common divisor is not 1, then set a[i][j] to 0. Sample Input: ./a.out 6 Sample Output: 000000 011111 010101 011011 010101 1110
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
What is the code in C++?
![1. Write a program to fill in a two-dimensional array of boolean values by
setting a[i][j] to 1 if the greatest common divisor of i and j is 1.
Else if the greatest common divisor is not 1, then set a[i][j] to 0.
Sample Input:
/a.out 6
Sample Output:
000000
011111
010101
011011
010101
011110](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F722c0934-aff7-4600-989e-1d198e6697ab%2Fcfc69b93-9b0e-4518-814f-248722474b63%2F5m4hchp_processed.jpeg&w=3840&q=75)
Transcribed Image Text:1. Write a program to fill in a two-dimensional array of boolean values by
setting a[i][j] to 1 if the greatest common divisor of i and j is 1.
Else if the greatest common divisor is not 1, then set a[i][j] to 0.
Sample Input:
/a.out 6
Sample Output:
000000
011111
010101
011011
010101
011110
Expert Solution

Step 1: Required Code in C++.
Required Code in C++.
#include <iostream>
using namespace std;
// Function to return greatest common divisor of x and y
int getGCD(int x, int y) {
if (x == 0)
return y;
return getGCD(y % x, x);
}
int main(int argc, char** argv) {
// If valid argument is not provided
if (argc != 2) {
cout << "Usage: ./a.out <size of 2D array>\n";
return 1;
}
// Convert size of 2d array to an integer
int n = stoi(argv[1]);
// Create 2D array of size nxn
bool** mat = new bool*[n]; // Create an array
for (int i = 0; i < n; i++) {
mat[i] = new bool[n];
for (int j = 0; j < n; j++) {
// If GCD of i and j is 1 then set corresponding value in 2d array to 1
if (getGCD(i, j) == 1)
mat[i][j] = 1;
else
mat[i][j] = 0;
cout << mat[i][j] << " ";
}
cout << endl;
}
// Release memory allocated to 2D array
for (int i = 0; i < n; i++)
delete[] mat[i];
delete[] mat;
return 0;
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
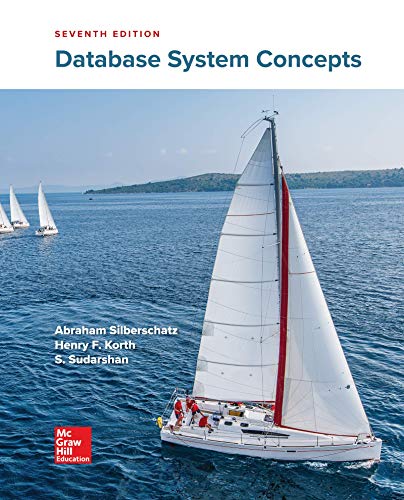
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
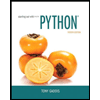
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
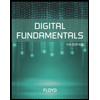
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
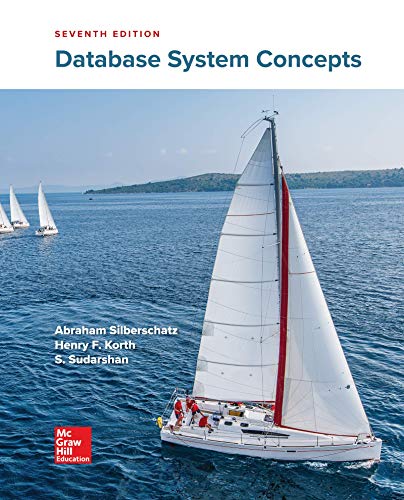
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
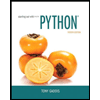
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
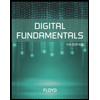
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
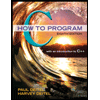
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
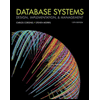
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
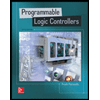
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education