inference
.py
keyboard_arrow_up
School
University of California, Berkeley *
*We aren’t endorsed by this school
Course
188
Subject
Computer Science
Date
Apr 26, 2024
Type
py
Pages
13
Uploaded by ElderMongoose4017 on coursehero.com
# inference.py
# ------------
# Licensing Information: You are free to use or extend these projects for
# educational purposes provided that (1) you do not distribute or publish
# solutions, (2) you retain this notice, and (3) you provide clear
# attribution to UC Berkeley, including a link to http://ai.berkeley.edu.
# # Attribution Information: The Pacman AI projects were developed at UC Berkeley.
# The core projects and autograders were primarily created by John DeNero
# (denero@cs.berkeley.edu) and Dan Klein (klein@cs.berkeley.edu).
# Student side autograding was added by Brad Miller, Nick Hay, and
# Pieter Abbeel (pabbeel@cs.berkeley.edu).
import random
import itertools
from typing import List, Dict, Tuple
import busters
import game
import bayesNet as bn
from bayesNet import normalize
import hunters
from util import manhattanDistance, raiseNotDefined
from factorOperations import joinFactorsByVariableWithCallTracking, joinFactors
from factorOperations import eliminateWithCallTracking
########### ########### ###########
########### QUESTION 1 ###########
########### ########### ###########
def constructBayesNet(gameState: hunters.GameState):
"""
Construct an empty Bayes net according to the structure given in Figure 1
of the project description.
You *must* name all variables using the constants in this function.
In this method, you should:
- populate `variables` with the Bayes Net nodes
- populate `edges` with every edge in the Bayes Net. we will represent each
edge as a tuple `(from, to)`.
- set each `variableDomainsDict[var] = values`, where `values` is a list
of the possible assignments to `var`.
- each agent position is a tuple (x, y) where x and y are 0-indexed
- each observed distance is a noisy Manhattan distance:
it's non-negative and |obs - true| <= MAX_NOISE
- this uses slightly simplified mechanics vs the ones used later for simplicity
"""
# constants to use
PAC = "Pacman"
GHOST0 = "Ghost0"
GHOST1 = "Ghost1"
OBS0 = "Observation0"
OBS1 = "Observation1"
X_RANGE = gameState.getWalls().width
Y_RANGE = gameState.getWalls().height
MAX_NOISE = 7
variables = []
edges = []
variableDomainsDict = {}
"*** YOUR CODE HERE ***"
# raiseNotDefined()
variables = [PAC, GHOST0, GHOST1, OBS0, OBS1] edges = [(GHOST0, OBS0), (PAC, OBS0), (PAC, OBS1), (GHOST1, OBS1)]
variableDomainsDict[PAC]= [(x,y) for x in range(X_RANGE) for y in range(Y_RANGE)]
variableDomainsDict[GHOST0] = [(x,y) for x in range(X_RANGE) for y in range(Y_RANGE)]
variableDomainsDict[GHOST1]= [(x,y) for x in range(X_RANGE) for y in range(Y_RANGE)]
variableDomainsDict[OBS0] = range(X_RANGE+Y_RANGE + MAX_NOISE - 1)
variableDomainsDict[OBS1]= range(X_RANGE+Y_RANGE + MAX_NOISE - 1)
"*** END YOUR CODE HERE ***"
net = bn.constructEmptyBayesNet(variables, edges, variableDomainsDict)
return net
def inferenceByEnumeration(bayesNet: bn, queryVariables: List[str], evidenceDict: Dict):
"""
An inference by enumeration implementation provided as reference.
This function performs a probabilistic inference query that
returns the factor:
P(queryVariables | evidenceDict)
bayesNet: The Bayes Net on which we are making a query.
queryVariables: A list of the variables which are unconditioned in
the inference query.
evidenceDict: An assignment dict {variable : value} for the
variables which are presented as evidence
(conditioned) in the inference query. """
callTrackingList = []
joinFactorsByVariable = joinFactorsByVariableWithCallTracking(callTrackingList)
eliminate = eliminateWithCallTracking(callTrackingList)
# initialize return variables and the variables to eliminate
evidenceVariablesSet = set(evidenceDict.keys())
queryVariablesSet = set(queryVariables)
eliminationVariables = (bayesNet.variablesSet() - evidenceVariablesSet) - queryVariablesSet
# grab all factors where we know the evidence variables (to reduce the size of the tables)
currentFactorsList = bayesNet.getAllCPTsWithEvidence(evidenceDict)
# join all factors by variable
for joinVariable in bayesNet.variablesSet():
currentFactorsList, joinedFactor = joinFactorsByVariable(currentFactorsList, joinVariable)
currentFactorsList.append(joinedFactor)
# currentFactorsList should contain the connected components of the graph now as factors, must join the connected components
fullJoint = joinFactors(currentFactorsList)
# marginalize all variables that aren't query or evidence
incrementallyMarginalizedJoint = fullJoint
for eliminationVariable in eliminationVariables:
incrementallyMarginalizedJoint = eliminate(incrementallyMarginalizedJoint, eliminationVariable)
fullJointOverQueryAndEvidence = incrementallyMarginalizedJoint
# normalize so that the probability sums to one
# the input factor contains only the query variables and the evidence variables, # both as unconditioned variables
queryConditionedOnEvidence = normalize(fullJointOverQueryAndEvidence)
# now the factor is conditioned on the evidence variables
# the order is join on all variables, then eliminate on all elimination variables
return queryConditionedOnEvidence
########### ########### ###########
########### QUESTION 4 ###########
########### ########### ###########
def inferenceByVariableEliminationWithCallTracking(callTrackingList=None):
def inferenceByVariableElimination(bayesNet: bn, queryVariables: List[str], evidenceDict: Dict, eliminationOrder: List[str]):
"""
This function should perform a probabilistic inference query that
returns the factor:
P(queryVariables | evidenceDict)
It should perform inference by interleaving joining on a variable
and eliminating that variable, in the order of variables according
to eliminationOrder. See inferenceByEnumeration for an example on
how to use these functions.
You need to use joinFactorsByVariable to join all of the factors that contain a variable in order for the autograder to recognize that you performed the correct interleaving of joins and eliminates.
If a factor that you are about to eliminate a variable from has only one unconditioned variable, you should not eliminate it and instead just discard the factor. This is since the result of the eliminate would be 1 (you marginalize all of the unconditioned variables), but it is not a valid factor. So this simplifies using the result of eliminate.
The sum of the probabilities should sum to one (so that it is a true conditional probability, conditioned on the evidence).
bayesNet: The Bayes Net on which we are making a query.
queryVariables: A list of the variables which are unconditioned
in the inference query.
evidenceDict: An assignment dict {variable : value} for the
variables which are presented as evidence
(conditioned) in the inference query. eliminationOrder: The order to eliminate the variables in.
Hint: BayesNet.getAllCPTsWithEvidence will return all the Conditional Probability Tables even if an empty dict (or None) is passed in for evidenceDict. In this case it will not specialize any variable domains in the CPTs.
Useful functions:
BayesNet.getAllCPTsWithEvidence
normalize
eliminate
joinFactorsByVariable
joinFactors
"""
# this is for autograding -- don't modify
joinFactorsByVariable = joinFactorsByVariableWithCallTracking(callTrackingList)
eliminate = eliminateWithCallTracking(callTrackingList)
if eliminationOrder is None: # set an arbitrary elimination order if None given
eliminationVariables = bayesNet.variablesSet() - set(queryVariables) -\
set(evidenceDict.keys())
eliminationOrder = sorted(list(eliminationVariables))
"*** YOUR CODE HERE ***"
# raiseNotDefined()
currentFactorsList = bayesNet.getAllCPTsWithEvidence(evidenceDict)
for var in eliminationOrder:
currentFactorsList, joinedFactor = joinFactorsByVariable(currentFactorsList, var)
length = len(joinedFactor.unconditionedVariables())
if length > 1:
currentFactorsList.append(eliminate(joinedFactor, var))
joined = joinFactors(currentFactorsList)
return normalize(joined)
"*** END YOUR CODE HERE ***"
return inferenceByVariableElimination
inferenceByVariableElimination = inferenceByVariableEliminationWithCallTracking()
def sampleFromFactorRandomSource(randomSource=None):
if randomSource is None:
randomSource = random.Random()
def sampleFromFactor(factor, conditionedAssignments=None):
"""
Sample an assignment for unconditioned variables in factor with
probability equal to the probability in the row of factor
corresponding to that assignment.
factor: The factor to sample from.
conditionedAssignments: A dict of assignments for all conditioned
variables in the factor. Can only be None
if there are no conditioned variables in
factor, otherwise must be nonzero.
Useful for inferenceByLikelihoodWeightingSampling
Returns an assignmentDict that contains the conditionedAssignments but also a random assignment of the unconditioned variables given their probability.
"""
if conditionedAssignments is None and len(factor.conditionedVariables()) > 0:
raise ValueError("Conditioned assignments must be provided since \n" +
"this factor has conditionedVariables: " + "\n" +
str(factor.conditionedVariables()))
elif conditionedAssignments is not None:
conditionedVariables = set([var for var in conditionedAssignments.keys()])
if not conditionedVariables.issuperset(set(factor.conditionedVariables())):
raise ValueError("Factor's conditioned variables need to be a subset of the \n"
+ "conditioned assignments passed in. \n" + \
"conditionedVariables: " + str(conditionedVariables) + "\n" +
"factor.conditionedVariables: " + str(set(factor.conditionedVariables())))
# Reduce the domains of the variables that have been
# conditioned upon for this factor newVariableDomainsDict = factor.variableDomainsDict()
for (var, assignment) in conditionedAssignments.items():
newVariableDomainsDict[var] = [assignment]
# Get the (hopefully) smaller conditional probability table
# for this variable CPT = factor.specializeVariableDomains(newVariableDomainsDict)
else:
CPT = factor
# Get the probability of each row of the table (along with the
# assignmentDict that it corresponds to)
assignmentDicts = sorted([assignmentDict for assignmentDict in CPT.getAllPossibleAssignmentDicts()])
assignmentDictProbabilities = [CPT.getProbability(assignmentDict) for assignmentDict in assignmentDicts]
# calculate total probability in the factor and index each row by the # cumulative sum of probability up to and including that row
currentProbability = 0.0
probabilityRange = []
for i in range(len(assignmentDicts)):
currentProbability += assignmentDictProbabilities[i]
probabilityRange.append(currentProbability)
totalProbability = probabilityRange[-1]
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
user stories
I need 5 user stories about Online Crime Management for my project
For example, user stories might look like that:As a police officer, i would like to be able to check for past records with an ID number(like a passport), so that I don’t have to use the name of the person
arrow_forward
Extreme Sports Web Site (Extreme Sports Web Site)
Extreme sports include activities that are considered to have a high degree of intrinsic risk, such as BMX racing, skate boarding, mountain biking, ice climbing, and base jumping, among others. As a lifelong enthusiast, you started selling hats and t-shirts with extreme sports themes, personalities, and inspiring sayings to raise money for charity. As a consequence, you have had the pleasure of travelling to many exotic locations across the globe over the last two years in order to sell your very successful goods at a variety of events and contests. When it comes to selling your goods to extreme sports enthusiasts all over the world, you're considering launching a Web site.
What difficulties could you encounter while attempting to distribute your goods to consumers in another country?
arrow_forward
– Ethical HackingLab #2 – Legal and Ethical
Overview
Write up a case that pertains to one of the laws mentioned in the course content or another law that is relevant to the cybersecurity landscape. Make sure to include your references. You should use what you learned about Google Hacking to find actual court documents that detail the case, the charges levied, and results of the case. Finding pertinent primary sources of information in this field can be quite a challenge.
Pick a case that has occurred in the last 7 years and summarize the main points of the case.
Explain which laws were cited in the case and go into detail about why they were applied. You may need to take a closer look at the law in question to describe this.
Explain the verdict of the case and your opinion of the ruling based on the law.
In your opinion, does the law need reform or will it still apply in the future. Why or why
arrow_forward
Add a research method
Research MethodsHere you should describe the methodologies that you are employed in your project and the sources that you used. Basic to most projects will be online searches for materials such as web pages, articles, videos, and blogs. Social media such as Twitter or Facebook may play a role. Personal interviews with important people knowledgeable in your topic may be included. In some cases, it may be a good idea to do a survey. Include information on matchups between your sources and the stakeholders that you have identified and whose viewpoints are represented. Describe specifically how the researchmethods tie in with how you are advancing your project.
arrow_forward
Computer Graphics:
Using Unreal Engine or any CGI Software provide two rendered objects in which at least five(5) of the following techniques are realized.
Sphere , Materials , Point Lighting , Spot Lighting , Directional Lighting , Ambient Lighting , Recursive Depth , Soft Shadow , Transparent Object , Depth of Field , Motion Blur , Texture Mapping and 3D Transformation
There is no restriction on what techniques you choose to demonstrate, nor on the scene you choose to develop, but you must submit the following.
A still rendered image, provided as a *.jpg or *.png file
A five to ten (5-10) second animation clip, provided as an *.mpg or suitable video formatted file.
arrow_forward
Please assist... thumbs up guaranteed if the solution is correct. thanks
arrow_forward
Job portal system: This system allows the students to search and apply for jobs etc. online. The manager can use the system for posting and deleting job offers etc.
1.Create your own case study (problem statement) for the above application
arrow_forward
comments appreciated
arrow_forward
Explain the concept of accessibility in user interface design. What are some best practices for designing interfaces that are inclusive and accessible to users with disabilities?
arrow_forward
Job portal system: This system allows the students to search and apply for jobs etc. online. The manager can use the system for posting and deleting job offers etc.
Create your own case study (problem statement) for the above application and
Draw the use case diagram
arrow_forward
How-tos for Microsoft Word The paper's name. As a connection, you might provide a hyperlink to an external file.
arrow_forward
Login and Registration form using GUI Swing
arrow_forward
no plagiarism should be unique
arrow_forward
Mountain Rescue Teams
Climbing mountains is a popular leisure activity in Great Britain that is enjoyed by a very large number of people every year. However, this hobby can be dangerous, especially when participants have not prepared adequately. Every year some people have to be rescued while in the mountains in Great Britain.
These rescues are conducted by teams of volunteers affiliated to the Mountaineering Trust. The teams search on foot. They navigate using maps prepared by Ordnance Survey, the official map-making body of the British government. Ordnance Survey maps have grids that allow anywhere in Great Britain to be located to the nearest 100 meters using a grid reference.
If necessary, the teams request assistance from the Royal Air Force (RAF), which provides search and rescue helicopters from its bases across Great Britain.
The DatabaseThe location, name, and height of every mountain is stored. The location is taken to be the grid reference of the summit and serves as a…
arrow_forward
Excel is a program that is used to
prepare a
text document
database
O spreadsheets
slide presentations
arrow_forward
Please explain ur thought process. Starting the code like shown
arrow_forward
Use Case Modeling
Instant messaging is an online chat that offers real-time text transmission over the Internet. The system contains the following requirements:
A user can:
Set profile including change profile picture, change name, and change status.
Manage account through either change privacy settings, change SIM number, or delete account
Send broadcast message by selecting a list of contacts
Create a group including choose a group name, choose the contacts to add, and choose a group picture.
Chat with a contact including send text, send an attachment, send contact, and send location.
Mute a conversation. Choose the duration to mute either one week, one month, or one year.
Make a voice/video call with a contact.
Search for a contact either through contacts chats or through contacts list
Invite friend
Model the use case diagram for the above system.
Write the use case narrative of the “Send Location” use case
Use-Case Name:
Use-Case ID:…
arrow_forward
Instruction: Identify if the following is UNIQUE IDENTIFIER or NOT.
Instructor ID
arrow_forward
Alert dont submit AI generated answer.
Python please solve all question.
arrow_forward
Requirements:
Should use continue.
Java Programming.
arrow_forward
Databases Write the assignment on a white paper
arrow_forward
Alert: Don't submit AI generated answer, and give proper explanation and step by step solution.
arrow_forward
Consequently, users may opt to submit data through the command line as opposed to the GUI.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
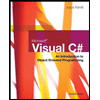
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Microsoft Windows 10 Comprehensive 2019
Computer Science
ISBN:9780357392607
Author:FREUND
Publisher:Cengage
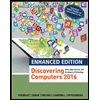
Enhanced Discovering Computers 2017 (Shelly Cashm...
Computer Science
ISBN:9781305657458
Author:Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. Campbell
Publisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
Related Questions
- user stories I need 5 user stories about Online Crime Management for my project For example, user stories might look like that:As a police officer, i would like to be able to check for past records with an ID number(like a passport), so that I don’t have to use the name of the personarrow_forwardExtreme Sports Web Site (Extreme Sports Web Site) Extreme sports include activities that are considered to have a high degree of intrinsic risk, such as BMX racing, skate boarding, mountain biking, ice climbing, and base jumping, among others. As a lifelong enthusiast, you started selling hats and t-shirts with extreme sports themes, personalities, and inspiring sayings to raise money for charity. As a consequence, you have had the pleasure of travelling to many exotic locations across the globe over the last two years in order to sell your very successful goods at a variety of events and contests. When it comes to selling your goods to extreme sports enthusiasts all over the world, you're considering launching a Web site. What difficulties could you encounter while attempting to distribute your goods to consumers in another country?arrow_forward– Ethical HackingLab #2 – Legal and Ethical Overview Write up a case that pertains to one of the laws mentioned in the course content or another law that is relevant to the cybersecurity landscape. Make sure to include your references. You should use what you learned about Google Hacking to find actual court documents that detail the case, the charges levied, and results of the case. Finding pertinent primary sources of information in this field can be quite a challenge. Pick a case that has occurred in the last 7 years and summarize the main points of the case. Explain which laws were cited in the case and go into detail about why they were applied. You may need to take a closer look at the law in question to describe this. Explain the verdict of the case and your opinion of the ruling based on the law. In your opinion, does the law need reform or will it still apply in the future. Why or whyarrow_forward
- Add a research method Research MethodsHere you should describe the methodologies that you are employed in your project and the sources that you used. Basic to most projects will be online searches for materials such as web pages, articles, videos, and blogs. Social media such as Twitter or Facebook may play a role. Personal interviews with important people knowledgeable in your topic may be included. In some cases, it may be a good idea to do a survey. Include information on matchups between your sources and the stakeholders that you have identified and whose viewpoints are represented. Describe specifically how the researchmethods tie in with how you are advancing your project.arrow_forwardComputer Graphics: Using Unreal Engine or any CGI Software provide two rendered objects in which at least five(5) of the following techniques are realized. Sphere , Materials , Point Lighting , Spot Lighting , Directional Lighting , Ambient Lighting , Recursive Depth , Soft Shadow , Transparent Object , Depth of Field , Motion Blur , Texture Mapping and 3D Transformation There is no restriction on what techniques you choose to demonstrate, nor on the scene you choose to develop, but you must submit the following. A still rendered image, provided as a *.jpg or *.png file A five to ten (5-10) second animation clip, provided as an *.mpg or suitable video formatted file.arrow_forwardPlease assist... thumbs up guaranteed if the solution is correct. thanksarrow_forward
- Job portal system: This system allows the students to search and apply for jobs etc. online. The manager can use the system for posting and deleting job offers etc. 1.Create your own case study (problem statement) for the above applicationarrow_forwardcomments appreciatedarrow_forwardExplain the concept of accessibility in user interface design. What are some best practices for designing interfaces that are inclusive and accessible to users with disabilities?arrow_forward
- Job portal system: This system allows the students to search and apply for jobs etc. online. The manager can use the system for posting and deleting job offers etc. Create your own case study (problem statement) for the above application and Draw the use case diagramarrow_forwardHow-tos for Microsoft Word The paper's name. As a connection, you might provide a hyperlink to an external file.arrow_forwardLogin and Registration form using GUI Swingarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Microsoft Windows 10 Comprehensive 2019Computer ScienceISBN:9780357392607Author:FREUNDPublisher:CengageEnhanced Discovering Computers 2017 (Shelly Cashm...Computer ScienceISBN:9781305657458Author:Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. CampbellPublisher:Cengage Learning
- Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
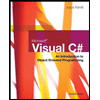
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Microsoft Windows 10 Comprehensive 2019
Computer Science
ISBN:9780357392607
Author:FREUND
Publisher:Cengage
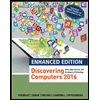
Enhanced Discovering Computers 2017 (Shelly Cashm...
Computer Science
ISBN:9781305657458
Author:Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. Campbell
Publisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage