Write a socket server program named "RockPaperScissorsServer", which communicates with players (clients). Server firstly receives a name for the player. Let’s assume 1 is Rock, 2 is Paper and 3 is Scissors. Next Server receives any of the 3 number from the user, generate a random number between 1 to 3 itself and then apply the Rock Paper Scissors game logic (which is paper beats rock, rock beats scissors, scissors beats paper), Then it will tell the player if the player or server has won. Write another client program to communicate with the server.
Topic: Data Communication & Network
Write a socket server program named "RockPaperScissorsServer", which communicates with players (clients). Server firstly receives a name for the player. Let’s assume 1 is Rock, 2 is Paper and 3 is Scissors. Next Server receives any of the 3 number from the user, generate a random number between 1 to 3 itself and then apply the Rock Paper Scissors game logic (which is paper beats rock, rock beats scissors, scissors beats paper), Then it will tell the player if the player or server has won. Write another client program to communicate with the server.
Kindly make the server in such a way the any time any player can leave and another player can join.
Use Java to write the code.
*** Reference code: Creating a simple Server ***
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.io.PrintWriter; import java.net.ServerSocket; import java.net.Socket;
import java.util.Scanner;
public class Server1 {
private static ServerSocket server = null; private static
Socket socket = null; private static final int port =
8080;
public static void main(String[] args) {
//Create IO Objects BufferedReader in = null; PrintWriter out = null; Scanner consoleInput = new Scanner(System.in);
//Start Server
try { System.out.println("Server is starting ..."); server = new ServerSocket(port); System.out.println("Server has started");
} catch (IOException e) { System.out.println("Can not listen to port: " + port); System.exit(-1);
}
while(true) { //Create Socket
try { socket = server.accept(); System.out.println("Client has been connected\n"); } catch
(IOException e) {
System.out.println("Communication Error with client"); System.exit(-1); }
try { in = new BufferedReader( new InputStreamReader( socket.getInputStream() ) );
out = new PrintWriter(socket.getOutputStream(), true);
out.println("NSU CSE338 LAB Server"); System.out.println("Client Name: " + in.readLine());
while(socket.isConnected()) { System.out.print("Server: "); out.println(consoleInput.nextLine()); System.out.print("Client: "); System.out.println(in.readLine()); }
} catch (IOException e) { System.out.print("Client Left"); consoleInput.close(); } }
}
}
*** Reference code: Creating a Client ***
import java.io.BufferedReader; import java.io.DataOutputStream; import java.io.IOException; import java.io.InputStreamReader; import java.io.PrintWriter; import java.net.Socket;
import java.util.Scanner;
public class Client1 {
public static Socket socket = null;
public static void main(String[] args) {
try { socket = new Socket("localhost", 8080);
System.out.println("Connected to Server\n" + "Socket: " + socket.getInetAddress() + ":" + socket.getPort() + "\n" ); } catch (IOException e) { System.out.print("Connection to network can not be stablished"); }
BufferedReader in = null; PrintWriter out = null; Scanner consoleInput = new Scanner(System.in);
try {
in = new BufferedReader( new InputStreamReader(
socket.getInputStream() ));
out = new PrintWriter(socket.getOutputStream(), true);
System.out.println("Server: " + in.readLine()); System.out.print("Client: your name_ ");
out.println(consoleInput.nextLine());
while(true) { System.out.print("Server: "); System.out.println(in.readLine()); System.out.print("Client: "); out.println(consoleInput.nextLine()); }
} catch (IOException e) {
e.printStackTrace();
} } }

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

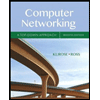
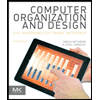
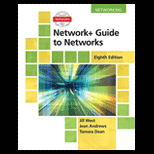
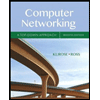
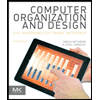
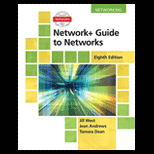
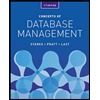
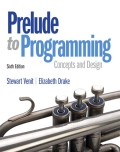
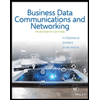