Write a program that reads the contents of a text file (Kennedy.txt). The program should create a dictionary in which the key-value pairs are described as follows: • Key. The keys are the individual words found in the file. • Values. Each value is a list that contains the line numbers in the file where the word (the key) is found. Once the dictionary is built, the program should create another text file, known as a word index, listing the contents of the dictionary.
Write a program that reads the contents of a text file (Kennedy.txt). The program should create a dictionary in which the key-value pairs are described as follows: • Key. The keys are the individual words found in the file. • Values. Each value is a list that contains the line numbers in the file where the word (the key) is found. Once the dictionary is built, the program should create another text file, known as a word index, listing the contents of the dictionary.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
There are many parts to this question and I'm in dire need of assistance.
please help in python
![In [103]:
#main function takes the input and output file and creates the word index
def main(infile, outfile, word_count_dict):
|| || ||
# test function
# create the index and word dictionary
>>> word_count_dict = {'we': 1, 'observe': 1, 'today': 1,
'not': 1, 'a': 3, 'victory': 1,
'of': 2, 'party': 1, 'but': 1,
'celebration': 1, 'freedom': 1,
'symbolizing': 1, 'an': 1, 'end': 1,
'as': 4, 'well': 2, 'beginning': 1,
'signifying': 1, 'renewal': 1, 'change': 1}
>>> infile = "kennedy.txt"; outfile = "index.txt"
>>> main(infile, outfile, word_count_dict)
Index saved to: index.txt
>>> with open (outfile) as f: print(f.read())
a: 1 2 4
an: 3
as: 4 5 6
beginning: 4
but: 2
celebration: 2
change: 6
end: 3
freedom: 3
not: 1
observe: 1
of: 2 3
party: 2
renewal: 5
signifying: 5
symbolizing: 3
today: 1
victory: 1
we: 1
well: 4 5
<BLANKLINE >
#read from the input text file and get a list of lines, set Error if problem reading
line_list, Error = read_to_list(infile)
if not Error:
# Get a dictionary holding the words and their line numbers.
word_dict = get_word_dict(line_list)
# Write the index file.
write_index_file(word_dict, outfile)
# Create the word list and word count List from the word_count_dict
word_list, word_count = word_count_dict_to_lists(word_count_dict)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F101176c3-68b2-418c-b51f-7bef8aae27d5%2F3e1a113c-7f9e-421b-b3a6-17354a198a06%2Fd8khlar_processed.png&w=3840&q=75)
Transcribed Image Text:In [103]:
#main function takes the input and output file and creates the word index
def main(infile, outfile, word_count_dict):
|| || ||
# test function
# create the index and word dictionary
>>> word_count_dict = {'we': 1, 'observe': 1, 'today': 1,
'not': 1, 'a': 3, 'victory': 1,
'of': 2, 'party': 1, 'but': 1,
'celebration': 1, 'freedom': 1,
'symbolizing': 1, 'an': 1, 'end': 1,
'as': 4, 'well': 2, 'beginning': 1,
'signifying': 1, 'renewal': 1, 'change': 1}
>>> infile = "kennedy.txt"; outfile = "index.txt"
>>> main(infile, outfile, word_count_dict)
Index saved to: index.txt
>>> with open (outfile) as f: print(f.read())
a: 1 2 4
an: 3
as: 4 5 6
beginning: 4
but: 2
celebration: 2
change: 6
end: 3
freedom: 3
not: 1
observe: 1
of: 2 3
party: 2
renewal: 5
signifying: 5
symbolizing: 3
today: 1
victory: 1
we: 1
well: 4 5
<BLANKLINE >
#read from the input text file and get a list of lines, set Error if problem reading
line_list, Error = read_to_list(infile)
if not Error:
# Get a dictionary holding the words and their line numbers.
word_dict = get_word_dict(line_list)
# Write the index file.
write_index_file(word_dict, outfile)
# Create the word list and word count List from the word_count_dict
word_list, word_count = word_count_dict_to_lists(word_count_dict)

Transcribed Image Text:1. Word Index and word count plot
Write a program that reads the contents of a text file (Kennedy.txt). The program should create a dictionary in which the key-value pairs are described as
follows:
• Key. The keys are the individual words found in the file.
• Values. Each value is a list that contains the line numbers in the file where the word (the key) is found.
Once the dictionary is built, the program should create another text file, known as a word index, listing the contents of the dictionary.
Finally take a word count dictionary and plot a bar chart of the word counts.
Algorithm Steps for the main() function:
1. Read the file's contents into a list
function read_to_list(infile) that returns line_list
2. create a dictionary holding the words and their line numbers
function get_word_dict(line_list) that returns word_dict
3. Write the index file
function write_index_file(word_dict,outfile)
4. Get the word count dictionary (word_count_dict is given) and return a word_list and word_count list
function word_count_dict_to_lists(word_count_dict) returns word_list and word_count_list
5. Plot the word count frequency in a horizontal bar plot
function plot_word_count (word_list, word_count, title,x_label,y_label)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
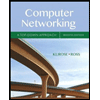
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
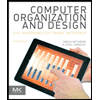
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
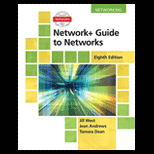
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
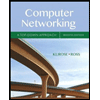
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
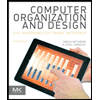
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
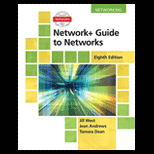
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
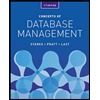
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
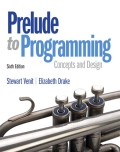
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
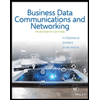
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY