We will write an Account class that represents a (simplified) bank account. A bank account keeps the name of the account holder, the account number, and the current balance. It has has the following methods: def init_(self, acc_name: str, acc_num: str, init_amt: int) – sets up an account with the speci- fied account name, number, and starting balance. def deposit(self, amt: int)-> int - adds the amt of money to the account balance and returns the new balance. If amt is zero or negative, it will raise an exception ValueError with an appropriate message. def withdraw(self, amt: int)-> bool - subtracts the amt of money from the account balance if it has sufficient funds and returns True. Otherwise, it must return False and leave the balance unchanged. This method never raises an exception. def transferTo(self, other_acc: Account, amt: int)-> bool transfers money from this account to another account. Specifically, a.transfer(b, 10) means transfer 10 from a to b. If amt is zero or negative, it will raise an exception ValueError with an appropriate message. If there is insufficient amount in the source account, abort the transfer and return False. Return True if the transfer is successful. When there is an exception, the balances must remain unchanged. def _str_(self)-> str returns the details of the account as a string. The format is as shown in the following example. Here's an example usage of the Account class. acc1 = Account('MUIC', 'A12345', 500) acc2 = Account('CS', 'A67890', 0) acc1.deposit(300) # returns 800 print (acc1) # => Name: MUIC, Acc No: A12345, Balance: 800 acc1.withdraw(450) # returns True print (acc1) # => Name: MUIC, Acc No: A12345, Balance: 350 acc1.withdraw(500) # Insufficient funds, so it returns False print (acc1) # => Name: MUIC, Acc No: A12345, Balance: 350 r = acc1.transferTo(acc2, 50) print (r) # => True print (acc1) # Name: MUIC, Acc No: A12345, Balance: 300 print (acc2) # Name: CS, Acc No: A67890, Balance: 50 r = acc2.transferTo (acc1, 5000) print (r) # => False r = acc2.transferTo (acc1, -10) # yields an exception ValueError
We will write an Account class that represents a (simplified) bank account. A bank account keeps the name of the account holder, the account number, and the current balance. It has has the following methods: def init_(self, acc_name: str, acc_num: str, init_amt: int) – sets up an account with the speci- fied account name, number, and starting balance. def deposit(self, amt: int)-> int - adds the amt of money to the account balance and returns the new balance. If amt is zero or negative, it will raise an exception ValueError with an appropriate message. def withdraw(self, amt: int)-> bool - subtracts the amt of money from the account balance if it has sufficient funds and returns True. Otherwise, it must return False and leave the balance unchanged. This method never raises an exception. def transferTo(self, other_acc: Account, amt: int)-> bool transfers money from this account to another account. Specifically, a.transfer(b, 10) means transfer 10 from a to b. If amt is zero or negative, it will raise an exception ValueError with an appropriate message. If there is insufficient amount in the source account, abort the transfer and return False. Return True if the transfer is successful. When there is an exception, the balances must remain unchanged. def _str_(self)-> str returns the details of the account as a string. The format is as shown in the following example. Here's an example usage of the Account class. acc1 = Account('MUIC', 'A12345', 500) acc2 = Account('CS', 'A67890', 0) acc1.deposit(300) # returns 800 print (acc1) # => Name: MUIC, Acc No: A12345, Balance: 800 acc1.withdraw(450) # returns True print (acc1) # => Name: MUIC, Acc No: A12345, Balance: 350 acc1.withdraw(500) # Insufficient funds, so it returns False print (acc1) # => Name: MUIC, Acc No: A12345, Balance: 350 r = acc1.transferTo(acc2, 50) print (r) # => True print (acc1) # Name: MUIC, Acc No: A12345, Balance: 300 print (acc2) # Name: CS, Acc No: A67890, Balance: 50 r = acc2.transferTo (acc1, 5000) print (r) # => False r = acc2.transferTo (acc1, -10) # yields an exception ValueError
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Python: Bank Account

Transcribed Image Text:We will write an Account class that represents a (simplified) bank account. A bank account keeps the name of
the account holder, the account number, and the current balance. It has has the following methods:
def init_(self, acc_name: str, acc_num: str, init_amt: int) sets up an account with the speci-
fied account name, number, and starting balance.
def deposit(self, amt: int)-> int - adds the amt of money to the account balance and returns the new
balance. If amt is zero or negative, it will raise an exception ValueError with an appropriate message.
def withdraw(self, amt: int)-> bool subtracts the amt of money from the account balance if it has
sufficient funds and returns True. Otherwise, it must return False and leave the balance unchanged. This
method never raises an exception.
def transferTo(self, other_acc: Account, amt: int)-> bool transfers money from this account to
another account. Specifically, a.transfer(b, 10) means transfer 10 from a to b. If amt is zero or
negative, it will raise an exception ValueError with an appropriate message. If there is insufficient
amount in the source account, abort the transfer and return False. Return True if the transfer is successful.
When there is an exception, the balances must remain unchanged.
def _str_(self)-> str – returns the details of the account as a string. The format is as shown in the
following example.
Here's an example usage of the Account class.
accl = Account('MUIC', 'A12345', 500)
acc2 = Account('CS', 'A67890', 0)
acc1.deposit(300) # returns 800
print (acc1) # => Name: MUIC, Acc No: A12345, Balance: 800
acc1.withdraw(450) # returns True
print (acc1) # => Name: MUIC, Acc No: A12345, Balance: 350
acc1.withdraw(500) # Insufficient funds, so it returns False
print (acc1) # => Name: MUIC, Acc No: A12345, Balance: 350
r = acc1.transferTo(acc2, 50)
print (r) # => True
print (acc1) # Name: MUIC, Acc No: A12345, Balance: 300
print (acc2) # Name: CS, Acc No: A67890, Balance: 50
r = acc2.transferTo(acc1, 5000)
print (r) # => False
r = acc2.transferTo(acc1, -10) # yields an exception ValueError
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
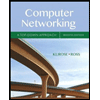
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
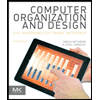
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
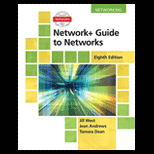
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
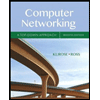
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
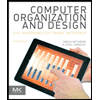
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
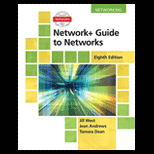
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
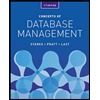
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
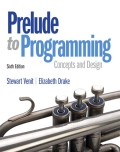
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
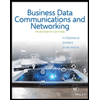
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY