Please solve the following problem in C++ and do not use vectors or while(true) make sure there is a condition in the while loop. Make the solution simple and do not use too advanced concepts. Given a hotel with 30 floors numbered 1-30 and up to 100 rooms per floor so that the lower 2 digits are the room number and upper digits are the floor number (e.g. room 1899 is the 99th room on the 18th floor), take reservation inputs that consist of pairs integers that represent the number of nights and a room number (each on a separate line) and terminated by "0 0", and output: (a) the average duration (number of nights) of a reservation (b) the floor number (1-30) that had the most reservations. If there is a tie for the floor with the most reservations, you may output ANY one of the floors that tied. For error checking of input values, ignore any input that has an illegal room number (i.e. above 3099 or below 100) or an input that has a negative number of nights for the reservation. Instead, count how many of these errors occur and output those values. Also output:
Please solve the following problem in C++ and do not use
Given a hotel with 30 floors numbered 1-30 and up to 100 rooms per floor so that the lower 2 digits are the room number and upper digits are the floor number (e.g. room 1899 is the 99th room on the 18th floor), take reservation inputs that consist of pairs integers that represent the number of nights and a room number (each on a separate line) and terminated by "0 0", and output:
(a) the average duration (number of nights) of a reservation
(b) the floor number (1-30) that had the most reservations. If there is a tie for the floor with the most reservations, you may output ANY one of the floors that tied.
For error checking of input values, ignore any input that has an illegal room number (i.e. above 3099 or below 100) or an input that has a negative number of nights for the reservation. Instead, count how many of these errors occur and output those values. Also output:
(c) the number of invalid room numbers entered
(d) the number of negative reservation durations.
Inputs from the user will contain at least one valid entry so that there will always be a floor with the most reservations and a non-zero average duration.
Use printResults() to output the answers.
An example input and output sequence is shown below.
Sample input:
101 2
3099 3
2054 -1
3100 -1
99 2
-546 3
3064 2
0 0
In the above there are only 3 valid input lines (the first 2 and the last). There are 3 lines with invalid room numbers (3100, 99, -546) and 2 lines with negative night durations. Notice that one input line can contain both and invalid room number AND a negative night duration. 2.3333 is the average number of nights per reservation for the valid inputs. And floor 30 is the floor with the most reservations.
The sample output:
Average nights per reservation: 2.33333
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

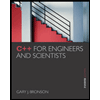
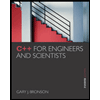