Min Heap of Candy — Add and Remove
Min Heap of Candy — Add and Remove
Using the provided code (Question04.java and Candyjava), write the add and remove methods for a min heap of Candy using an array implementation. Make sure to include all provided files in your project and write your solution in the file "Question04.java". This method should preserve the properties of a min hap, and the candies are compared based on their weight. This value can be accessed through its accessor.
Solution Tests:
• Does the solution compile?
• Does the solution have your name in the comments?
• Does the solution have a high-level solution description (150-300 words) in the comments?
• Does the method preserve the properties of a Min Heap?
• Does the output match the following?
Testing the Add Method
Candy Weight: 10.0,
Candy Weight: 40.0,
Candy Weight: 20.0,
Candy Weight: 50.0,
Candy Weight: 60.0,
Candy Weight: 80.0,
Candy Weight: 30.0,
Candy Weight: 100.0,
Candy Weight: 70.0,
Candy Weight: 90.0,
Testing the Remove Method
Candy Weight: 10.0,
Candy Weight: 20.0,
Candy Weight: 30.0,
Candy Weight: 40.0,
Candy Weight: 50.0,
Candy Weight: 60.0,
Candy Weight: 70.0,
Candy Weight: 80.0,
Candy Weight: 90.0,
Candy Weight: 100.0,
![//Do not alter-
public class Question04
private Candy [] heap = new Candy [10];
public int size;//First null element
public void add(Candy aData)
{
//Write your code here
}//Do not alter
//Do not alter-
public Candy remove()
{
//Write your code here
}//Do not alter
//Write any additional helper properties or methods here
//Do not alter-
public void printBreadthorder()
{
for(Candy c : this.heap)
{
if(c != nul)
System.out.print(c+", ");
else
System.out.print("null, ");
}
System.out.println();
}
Run | Debug
public static void main(String[] args)
{
System.out.println("Testing the Add Method");
Candy [] array = {
new Candy (100.0),
new Candy (80.0),
new Candy(60.0),
new Candy(40.0),
new Candy(20.0),
new Candy(10.0),
new Candy (30.0),
new Candy(50.0),
new Candy(70.0),
new Candy (90.0)};
Question04 heap = new Question04();
for(Candy c : array)
heap.add (c);
heap.printBreadthorder();
System.out.println("Testing the Remove Method");
for(int i=0;i<array.length;i++)
System.out.print(heap.remove() +", ");
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcec9c358-132d-4e0e-a629-c9920901d8f4%2F658e44fa-d1d9-4170-a653-2388698a800e%2F70mngkl_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

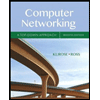
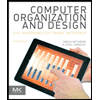
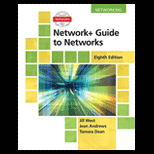
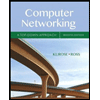
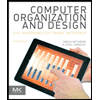
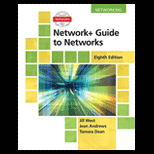
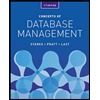
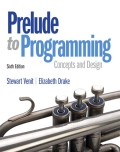
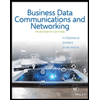