I need an explanation for this program. class Directory { Node head,tail; Directory() { head=null; tail=null; } public void insert(Node node) // method that insers node into list if phone number is new { Node temp=head; while(temp!=null && !temp.phone_number.equals(node.phone_number)) temp=temp.next; if(temp!=null) { System.out.println("Entered phone number already exists"); return; } if(head==null) { head=node; tail=node; } else { tail.next=node; tail=node; } System.out.println("Inserted Successfully"); } public void remove(String ph_no) // method that removes node with given phone number { Node prev=null; Node node=head; while(node!=null && !node.phone_number.equals(ph_no)) { prev=node; node=node.next; } if(node==null) System.out.println("Phone number not found"); else if(prev==null) { head=head.next; if(head==null) tail=null; System.out.println("Deleted Successfully"); } else { prev.first_name=node.first_name; prev.last_name=node.last_name; prev.phone_number=node.phone_number; prev.city=node.city; prev.gender=node.gender; prev.address=node.address; prev.email=node.email; prev.next=node.next; System.out.println("Deleted Successfully"); } } public void update(String first_name,String phone_number) // method that updates the phone number of person if exists { boolean found=false; Node node=head; while(node!=null) { if(node.first_name.equals(first_name)) { node.phone_number=phone_number; found=true; } node=node.next; } if(!found) System.out.println("No person found with given first name"); else System.out.println("Updated Successfully"); } public void display() // method that displays data in directory { if(head==null) { System.out.println("Empty directory"); return; } System.out.println("People are as follows: "); Node node=head; while(node!=null) { System.out.println(node.toString()); node=node.next; } } public void search(String first_name) // method that searches for person with first name { Node node=head; boolean found=false; while(node!=null) { if(node.first_name.equals(first_name)) { System.out.println(node.toString()); found=true; } node=node.next; } if(!found) System.out.println("No person found with given first name"); } public void sort() // sorts data in linked lists according to first name { Node node1=head; while(node1!=null) { Node node2=node1.next; while(node2!=null) { if(node1.first_name.compareTo(node2.first_name)>0) { swap(node1,node2); } node2=node2.next; } node1=node1.next; } System.out.println("Sorted Successfully"); } public void swap(Node n1,Node n2) { String temp=""; temp=n1.first_name; n1.first_name=n2.first_name; n2.first_name=temp; temp=n1.last_name; n1.last_name=n2.last_name; n2.last_name=temp; temp=n1.phone_number; n1.phone_number=n2.phone_number; n2.phone_number=temp; temp=n1.city; n1.city=n2.city; n2.city=temp; temp=n1.address; n1.address=n2.address; n2.address=temp; temp=n1.email; n1.email=n2.email; n2.email=temp; char t=n1.gender; n1.gender=n2.gender; n2.gender=t; } } // prototype of person details class Node { String first_name,last_name,phone_number,city,address,email; char gender; Node next=null; Node() { } Node(String first_name,String last_name,String phone_number,String city,String address,char gender,String email) { this.first_name=first_name; this.last_name=last_name; this.phone_number=phone_number; this.city=city; this.address=address; this.gender=gender; this.email=email; } public String toString() { return "\nFirst Name: "+this.first_name+"\nLast Name: "+this.last_name+"\nPhone Number: "+this.phone_number+"\nCity: "+this.city+ "\nAddress: "+this.address+"\nGender: "+this.gender+"\nEmail: "+this.email+"\n"; } }
I need an explanation for this program. class Directory { Node head,tail; Directory() { head=null; tail=null; } public void insert(Node node) // method that insers node into list if phone number is new { Node temp=head; while(temp!=null && !temp.phone_number.equals(node.phone_number)) temp=temp.next; if(temp!=null) { System.out.println("Entered phone number already exists"); return; } if(head==null) { head=node; tail=node; } else { tail.next=node; tail=node; } System.out.println("Inserted Successfully"); } public void remove(String ph_no) // method that removes node with given phone number { Node prev=null; Node node=head; while(node!=null && !node.phone_number.equals(ph_no)) { prev=node; node=node.next; } if(node==null) System.out.println("Phone number not found"); else if(prev==null) { head=head.next; if(head==null) tail=null; System.out.println("Deleted Successfully"); } else { prev.first_name=node.first_name; prev.last_name=node.last_name; prev.phone_number=node.phone_number; prev.city=node.city; prev.gender=node.gender; prev.address=node.address; prev.email=node.email; prev.next=node.next; System.out.println("Deleted Successfully"); } } public void update(String first_name,String phone_number) // method that updates the phone number of person if exists { boolean found=false; Node node=head; while(node!=null) { if(node.first_name.equals(first_name)) { node.phone_number=phone_number; found=true; } node=node.next; } if(!found) System.out.println("No person found with given first name"); else System.out.println("Updated Successfully"); } public void display() // method that displays data in directory { if(head==null) { System.out.println("Empty directory"); return; } System.out.println("People are as follows: "); Node node=head; while(node!=null) { System.out.println(node.toString()); node=node.next; } } public void search(String first_name) // method that searches for person with first name { Node node=head; boolean found=false; while(node!=null) { if(node.first_name.equals(first_name)) { System.out.println(node.toString()); found=true; } node=node.next; } if(!found) System.out.println("No person found with given first name"); } public void sort() // sorts data in linked lists according to first name { Node node1=head; while(node1!=null) { Node node2=node1.next; while(node2!=null) { if(node1.first_name.compareTo(node2.first_name)>0) { swap(node1,node2); } node2=node2.next; } node1=node1.next; } System.out.println("Sorted Successfully"); } public void swap(Node n1,Node n2) { String temp=""; temp=n1.first_name; n1.first_name=n2.first_name; n2.first_name=temp; temp=n1.last_name; n1.last_name=n2.last_name; n2.last_name=temp; temp=n1.phone_number; n1.phone_number=n2.phone_number; n2.phone_number=temp; temp=n1.city; n1.city=n2.city; n2.city=temp; temp=n1.address; n1.address=n2.address; n2.address=temp; temp=n1.email; n1.email=n2.email; n2.email=temp; char t=n1.gender; n1.gender=n2.gender; n2.gender=t; } } // prototype of person details class Node { String first_name,last_name,phone_number,city,address,email; char gender; Node next=null; Node() { } Node(String first_name,String last_name,String phone_number,String city,String address,char gender,String email) { this.first_name=first_name; this.last_name=last_name; this.phone_number=phone_number; this.city=city; this.address=address; this.gender=gender; this.email=email; } public String toString() { return "\nFirst Name: "+this.first_name+"\nLast Name: "+this.last_name+"\nPhone Number: "+this.phone_number+"\nCity: "+this.city+ "\nAddress: "+this.address+"\nGender: "+this.gender+"\nEmail: "+this.email+"\n"; } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
I need an explanation for this program.
class Directory
{
Node head,tail;
Directory()
{
head=null;
tail=null;
}
public void insert(Node node) // method that insers node into list if phone number is new
{
Node temp=head;
while(temp!=null && !temp.phone_number.equals(node.phone_number))
temp=temp.next;
if(temp!=null)
{
System.out.println("Entered phone number already exists");
return;
}
if(head==null)
{
head=node;
tail=node;
}
else
{
tail.next=node;
tail=node;
}
System.out.println("Inserted Successfully");
}
public void remove(String ph_no) // method that removes node with given phone number
{
Node prev=null;
Node node=head;
while(node!=null && !node.phone_number.equals(ph_no))
{
prev=node;
node=node.next;
}
if(node==null)
System.out.println("Phone number not found");
else if(prev==null)
{
head=head.next;
if(head==null)
tail=null;
System.out.println("Deleted Successfully");
}
else
{
prev.first_name=node.first_name;
prev.last_name=node.last_name;
prev.phone_number=node.phone_number;
prev.city=node.city;
prev.gender=node.gender;
prev.address=node.address;
prev.email=node.email;
prev.next=node.next;
System.out.println("Deleted Successfully");
}
}
public void update(String first_name,String phone_number) // method that updates the phone number of person if exists
{
boolean found=false;
Node node=head;
while(node!=null)
{
if(node.first_name.equals(first_name))
{
node.phone_number=phone_number;
found=true;
}
node=node.next;
}
if(!found)
System.out.println("No person found with given first name");
else
System.out.println("Updated Successfully");
}
public void display() // method that displays data in directory
{
if(head==null)
{
System.out.println("Empty directory");
return;
}
System.out.println("People are as follows: ");
Node node=head;
while(node!=null)
{
System.out.println(node.toString());
node=node.next;
}
}
public void search(String first_name) // method that searches for person with first name
{
Node node=head;
boolean found=false;
while(node!=null)
{
if(node.first_name.equals(first_name))
{
System.out.println(node.toString());
found=true;
}
node=node.next;
}
if(!found)
System.out.println("No person found with given first name");
}
public void sort() // sorts data in linked lists according to first name
{
Node node1=head;
while(node1!=null)
{
Node node2=node1.next;
while(node2!=null)
{
if(node1.first_name.compareTo(node2.first_name)>0)
{
swap(node1,node2);
}
node2=node2.next;
}
node1=node1.next;
}
System.out.println("Sorted Successfully");
}
public void swap(Node n1,Node n2)
{
String temp="";
temp=n1.first_name;
n1.first_name=n2.first_name;
n2.first_name=temp;
temp=n1.last_name;
n1.last_name=n2.last_name;
n2.last_name=temp;
temp=n1.phone_number;
n1.phone_number=n2.phone_number;
n2.phone_number=temp;
temp=n1.city;
n1.city=n2.city;
n2.city=temp;
temp=n1.address;
n1.address=n2.address;
n2.address=temp;
temp=n1.email;
n1.email=n2.email;
n2.email=temp;
char t=n1.gender;
n1.gender=n2.gender;
n2.gender=t;
}
}
// prototype of person details
class Node
{
String first_name,last_name,phone_number,city,address,email;
char gender;
Node next=null;
Node() { }
Node(String first_name,String last_name,String phone_number,String city,String address,char gender,String email)
{
this.first_name=first_name;
this.last_name=last_name;
this.phone_number=phone_number;
this.city=city;
this.address=address;
this.gender=gender;
this.email=email;
}
public String toString()
{
return "\nFirst Name: "+this.first_name+"\nLast Name: "+this.last_name+"\nPhone Number: "+this.phone_number+"\nCity: "+this.city+
"\nAddress: "+this.address+"\nGender: "+this.gender+"\nEmail: "+this.email+"\n";
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Recommended textbooks for you
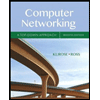
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
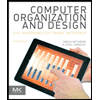
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
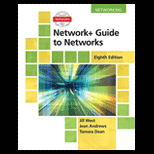
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
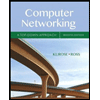
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
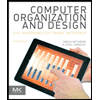
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
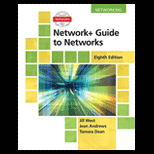
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
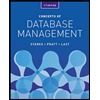
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
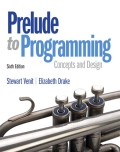
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
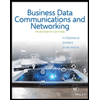
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY