I am writing a code to make a card game that will show the user's card and the computers card when "cut" is clicked. However, I don't quite know where to insert resize() to resize the images for the suits to make them smaller. from Button import Button import random ranks = ["2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", "A"] suits = ["Spades", "Hearts", "Diamonds", "Clubs"] image_filenames = ["spade.png", "heart.png", "diamond.png", "club.png"] images = [] blank_image = None btn = None def setup(): global btn, images, blank_image, image_filenames for i in range(len(image_filenames)): images.append(loadImage(image_filenames[i])) # may need to resize these! blank_image = loadImage("blank.png") size(400,400) btn = Button(width /2, height/2, 60, 40, "Cut") def draw(): global btn background(127) image(images[0], width*0.1, height*0.1) image(images[1], width*0.2, height*0.1) image(images[2], width*0.3, height*0.1) image(images[3], width*0.4, height*0.1) btn.show() def mouseClicked(): if btn.collide(mouseX, mouseY): rank, suit_index = cut() print(rank + ' ' + suits[suit_index]) def cut(): rank = random.choice(ranks) suit_index = random.randrange(4) return rank, suit_index The code for file "Button": class Button: def __init__(self, x, y, w, h, text): self.X = x self.Y = y self.W = w self.H = h self.text = text def collide(self, x, y): if x >= self.X - self.W / 2 and x <= self.X + \ self.W / 2 and y >= self.Y - self.H / 2 and y <= self.Y + self.H / 2: return True return False def show(self): fill(255) stroke(0) rectMode(CENTER) rect(self.X, self.Y, self.W, self.H) textSize(22) textAlign(CENTER,CENTER) fill(0) noStroke() text(self.text,self.X,self.Y)
I am writing a code to make a card game that will show the user's card and the computers card when "cut" is clicked. However, I don't quite know where to insert resize() to resize the images for the suits to make them smaller.
from Button import Button
import random
ranks = ["2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", "A"]
suits = ["Spades", "Hearts", "Diamonds", "Clubs"]
image_filenames = ["spade.png", "heart.png", "diamond.png", "club.png"]
images = []
blank_image = None
btn = None
def setup():
global btn, images, blank_image, image_filenames
for i in range(len(image_filenames)):
images.append(loadImage(image_filenames[i])) # may need to resize these!
blank_image = loadImage("blank.png")
size(400,400)
btn = Button(width /2, height/2, 60, 40, "Cut")
def draw():
global btn
background(127)
image(images[0], width*0.1, height*0.1)
image(images[1], width*0.2, height*0.1)
image(images[2], width*0.3, height*0.1)
image(images[3], width*0.4, height*0.1)
btn.show()
def mouseClicked():
if btn.collide(mouseX, mouseY):
rank, suit_index = cut()
print(rank + ' ' + suits[suit_index])
def cut():
rank = random.choice(ranks)
suit_index = random.randrange(4)
return rank, suit_index
The code for file "Button":
class Button:
def __init__(self, x, y, w, h, text):
self.X = x
self.Y = y
self.W = w
self.H = h
self.text = text
def collide(self, x, y):
if x >= self.X - self.W / 2 and x <= self.X + \
self.W / 2 and y >= self.Y - self.H / 2 and y <= self.Y + self.H / 2:
return True
return False
def show(self):
fill(255)
stroke(0)
rectMode(CENTER)
rect(self.X, self.Y, self.W, self.H)
textSize(22)
textAlign(CENTER,CENTER)
fill(0)
noStroke()
text(self.text,self.X,self.Y)


Step by step
Solved in 3 steps

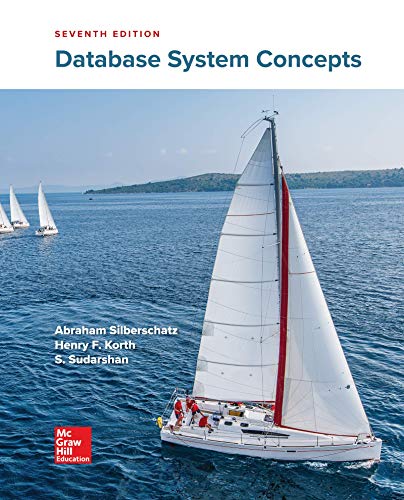
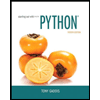
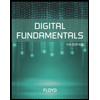
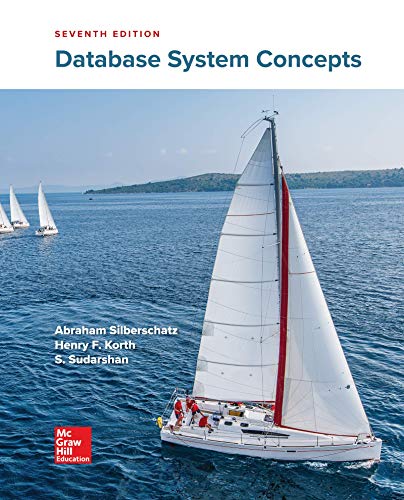
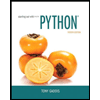
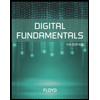
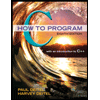
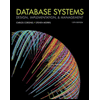
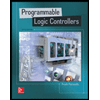