Celsius Temperature Table //write code under void challenge and create function The formula for converting a temperature from Fahrenheit to Celsius is C=59(F−32)C=59(F-32) where F is the Fahrenheit temperature and C is the Celsius temperature. Write a function named celsius that accepts a Fahrenheit temperature as an argument. The function should return the temperature, converted to Celsius. Demonstrate the function by calling it in a loop that displays a table of the Fahrenheit temperatures 0 through 20 and their Celsius equivalents.\ my code below please adust and use chapter 1- 6 terminology //use only chapter 1-6 terminology!! // must validate input using do while loop examle line 98 - 108 // //call functions and follow the challenge instructions //Description: Assignment6 C++ Chapter 6 #include #include #include #include using namespace std; // Function prototypes int menuOption(); double getSales(string divisionName); void findHighest(double salesNE, double salesSE, double salesNW, double salesSW); void Challenge3(); double fallingDistance(int time); void Challenge5(); double celsius(); void Challenge7(); void Challenge9(); void Challenge10(); void Challenge11(); void Challenge18(); void Challenge20(); void Challenge23(); void Challenge24(); // driver function int main() { do { switch (menuOption()) { case 0: exit(1); break; case 3: system("cls"); Challenge3(); break; case 5: system("cls"); Challenge5(); break; case 7: system("cls"); Challenge7(); break; case 9: system("cls"); Challenge9(); break; case 10: system("cls"); Challenge10(); break; case 11: system("cls"); Challenge11(); break; case 18: system("cls"); Challenge18(); break; case 20: system("cls"); Challenge20(); break; case 23: system("cls"); Challenge23(); break; case 24: system("cls"); Challenge24(); break; default: cout << "\t\tERROR - Invalid option. Please re-enter."; break; } cout << "\n"; system("pause"); } while (true); return EXIT_SUCCESS; } int menuOption() { system("cls"); cout << "\n\t\tChapter 6: Functions (page 372-380) "; cout << "\n\t" + string(60, char(205)); cout << "\n\t\t 3. Winning Division "; cout << "\n\t\t 5. Falling Distance "; cout << "\n\t\t 7. Celsius Temperature Table "; cout << "\n\t\t 9. Present Value "; cout << "\n\t\t 10. Future Value "; cout << "\n\t\t 11. Lowest Score Drop "; cout << "\n\t\t 18. Paint Job Estimator "; cout << "\n\t\t 20. Stock Profit "; cout << "\n\t\t 23. Prime Number List "; cout << "\n\t\t 24. Rock, Paper, Scissors Game"; cout << "\n\t" + string(60, char(196)); cout << "\n\t\t 0. Quit"; cout << "\n\t" + string(60, char(205)); cout << "\n\t\t Option: "; int option; cin >> option; cin.clear(); cin.ignore(999, '\n'); return option; } double getSales(string divisionName) { double sales; // local variable do { cout << "Enter the quarterly sales for " << divisionName << ": $"; cin >> sales; if (sales < 0) cout << "Error: invalid input sales.\n"; else return sales; } while (true); } //declaring prototype void findHighest(double salesNE, double salesSE, double salesNW, double salesSW) { if (salesNE >= salesSE && salesNE >= salesNW && salesNE >= salesSW) cout << "Sales North East: $" << salesNE; else if (salesSE >= salesNE && salesNE >= salesNW && salesSE >= salesSW) cout << "Sales South East: $" << salesSE; else if (salesNW >= salesNE && salesNE >= salesNW && salesSE >= salesSW) cout << "Sales NorthWest: $" << salesNW; else cout << "Sales South West: $" << salesSW; } // Winning Division - page 373 void Challenge3() { double salesNE = getSales("NorthEast"); double salesSE = getSales("SouthEast"); double salesNW = getSales("NorthWest"); double salesSW = getSales("SouthWest"); //Output findHighest(salesNE, salesSE, salesNW, salesSW); } double fallingDistance(int time) { return 0.5 * 9.8 * pow(time, 2); } // Falling Distance - pg 374 void Challenge5() { int time = 0; // do while loop for validation do { cout << "Enter the time in seconds: "; cin >> time; if (time < 0) cout << "Error: invalid input time.\n"; else break; // break out of the loop } while (true); cout << "The distance: " << fallingDistance(time) << '\n'; } //Celsius Temperature Table double celsius() { return 0.0; } void Challenge7() { const int minTemp = 0, maxTemp = 20; double C; } void Challenge9() { // You will implement } void Challenge10() { // You will implement } void Challenge11() { // You will implement } void Challenge18() { // You will implement } void Challenge20() { // You will implement } void Challenge23() { // You will implement } void Challenge24() { // You will implement }
7.Celsius Temperature Table //write code under void challenge and create function
The formula for converting a temperature from Fahrenheit to Celsius is
where F is the Fahrenheit temperature and C is the Celsius temperature. Write a function named celsius that accepts a Fahrenheit temperature as an argument. The function should return the temperature, converted to Celsius. Demonstrate the function by calling it in a loop that displays a table of the Fahrenheit temperatures 0 through 20 and their Celsius equivalents.\
my code below please adust and use chapter 1- 6 terminology
//use only chapter 1-6 terminology!!
// must validate input using do while loop examle line 98 - 108
// //call functions and follow the challenge instructions
//Description: Assignment6 C++ Chapter 6
#include <iostream>
#include <string>
#include <iomanip>
#include <fstream>
using namespace std;
// Function prototypes
int menuOption();
double getSales(string divisionName);
void findHighest(double salesNE, double salesSE, double salesNW, double salesSW);
void Challenge3();
double fallingDistance(int time);
void Challenge5();
double celsius();
void Challenge7();
void Challenge9();
void Challenge10();
void Challenge11();
void Challenge18();
void Challenge20();
void Challenge23();
void Challenge24();
// driver function
int main()
{
do
{
switch (menuOption())
{
case 0: exit(1); break;
case 3: system("cls"); Challenge3(); break;
case 5: system("cls"); Challenge5(); break;
case 7: system("cls"); Challenge7(); break;
case 9: system("cls"); Challenge9(); break;
case 10: system("cls"); Challenge10(); break;
case 11: system("cls"); Challenge11(); break;
case 18: system("cls"); Challenge18(); break;
case 20: system("cls"); Challenge20(); break;
case 23: system("cls"); Challenge23(); break;
case 24: system("cls"); Challenge24(); break;
default: cout << "\t\tERROR - Invalid option. Please re-enter."; break;
}
cout << "\n";
system("pause");
} while (true);
return EXIT_SUCCESS;
}
int menuOption()
{
system("cls");
cout << "\n\t\tChapter 6: Functions (page 372-380) ";
cout << "\n\t" + string(60, char(205));
cout << "\n\t\t 3. Winning Division ";
cout << "\n\t\t 5. Falling Distance ";
cout << "\n\t\t 7. Celsius Temperature Table ";
cout << "\n\t\t 9. Present Value ";
cout << "\n\t\t 10. Future Value ";
cout << "\n\t\t 11. Lowest Score Drop ";
cout << "\n\t\t 18. Paint Job Estimator ";
cout << "\n\t\t 20. Stock Profit ";
cout << "\n\t\t 23. Prime Number List ";
cout << "\n\t\t 24. Rock, Paper, Scissors Game";
cout << "\n\t" + string(60, char(196));
cout << "\n\t\t 0. Quit";
cout << "\n\t" + string(60, char(205));
cout << "\n\t\t Option: ";
int option;
cin >> option;
cin.clear();
cin.ignore(999, '\n');
return option;
}
double getSales(string divisionName)
{
double sales; // local variable
do
{
cout << "Enter the quarterly sales for " << divisionName << ": $";
cin >> sales;
if (sales < 0)
cout << "Error: invalid input sales.\n";
else
return sales;
} while (true);
}
//declaring prototype
void findHighest(double salesNE, double salesSE, double salesNW, double salesSW)
{
if (salesNE >= salesSE && salesNE >= salesNW && salesNE >= salesSW)
cout << "Sales North East: $" << salesNE;
else if (salesSE >= salesNE && salesNE >= salesNW && salesSE >= salesSW)
cout << "Sales South East: $" << salesSE;
else if (salesNW >= salesNE && salesNE >= salesNW && salesSE >= salesSW)
cout << "Sales NorthWest: $" << salesNW;
else
cout << "Sales South West: $" << salesSW;
}
// Winning Division - page 373
void Challenge3()
{
double salesNE = getSales("NorthEast");
double salesSE = getSales("SouthEast");
double salesNW = getSales("NorthWest");
double salesSW = getSales("SouthWest");
//Output
findHighest(salesNE, salesSE, salesNW, salesSW);
}
double fallingDistance(int time)
{
return 0.5 * 9.8 * pow(time, 2);
}
// Falling Distance - pg 374
void Challenge5()
{
int time = 0;
// do while loop for validation
do
{
cout << "Enter the time in seconds: ";
cin >> time;
if (time < 0)
cout << "Error: invalid input time.\n";
else
break; // break out of the loop
} while (true);
cout << "The distance: " << fallingDistance(time) << '\n';
}
//Celsius Temperature Table
double celsius()
{
return 0.0;
}
void Challenge7()
{
const int minTemp = 0,
maxTemp = 20;
double C;
}
void Challenge9()
{
// You will implement
}
void Challenge10()
{
// You will implement
}
void Challenge11()
{
// You will implement
}
void Challenge18()
{
// You will implement
}
void Challenge20()
{
// You will implement
}
void Challenge23()
{
// You will implement
}
void Challenge24()
{
// You will implement
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

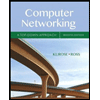
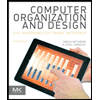
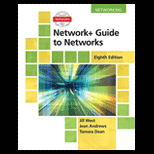
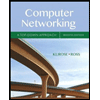
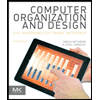
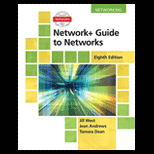
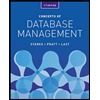
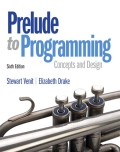
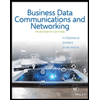